Getting Started with Node.js: A Beginner’s Guide
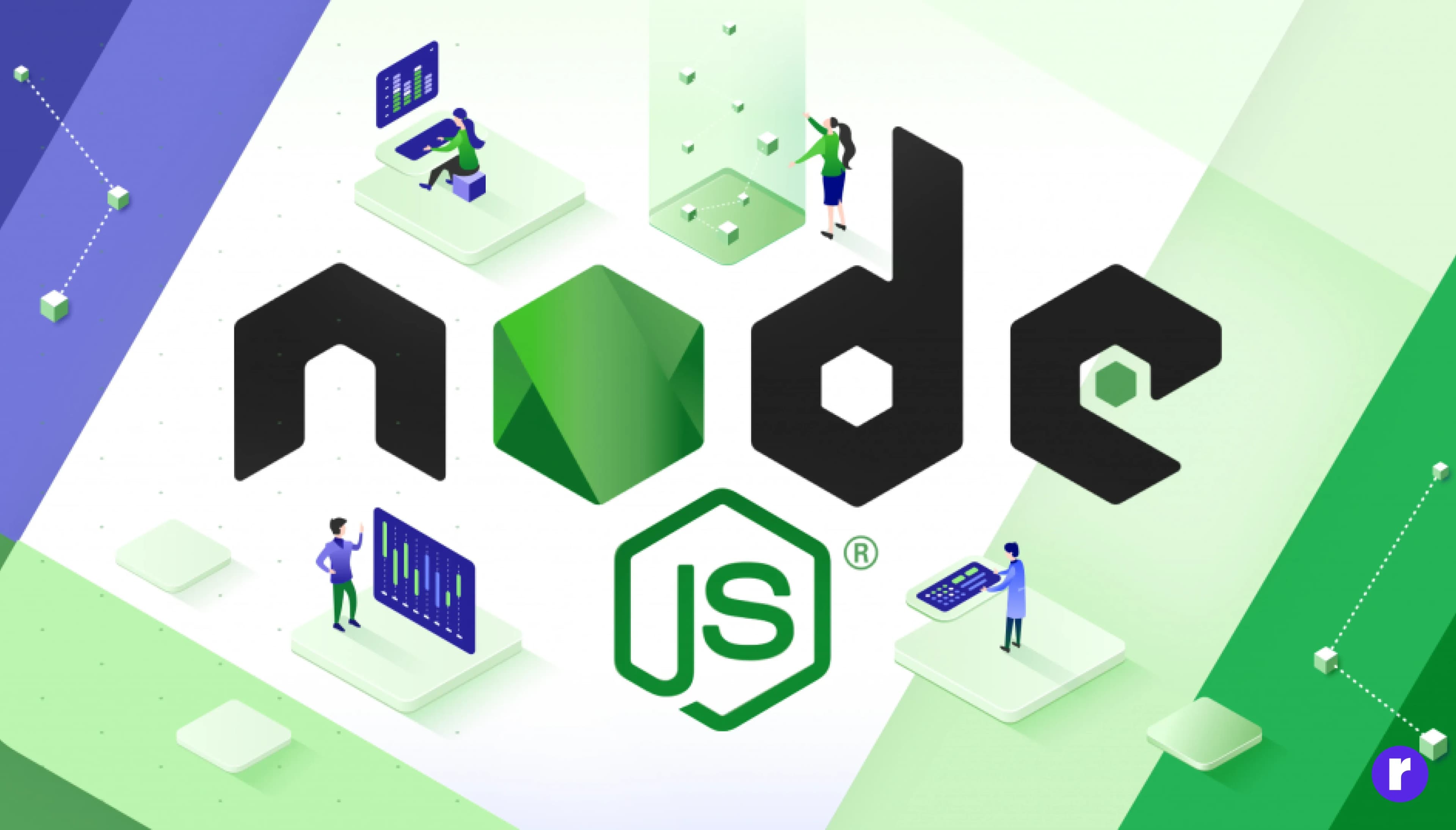
Written by
Keshav Saini
Front End Developer
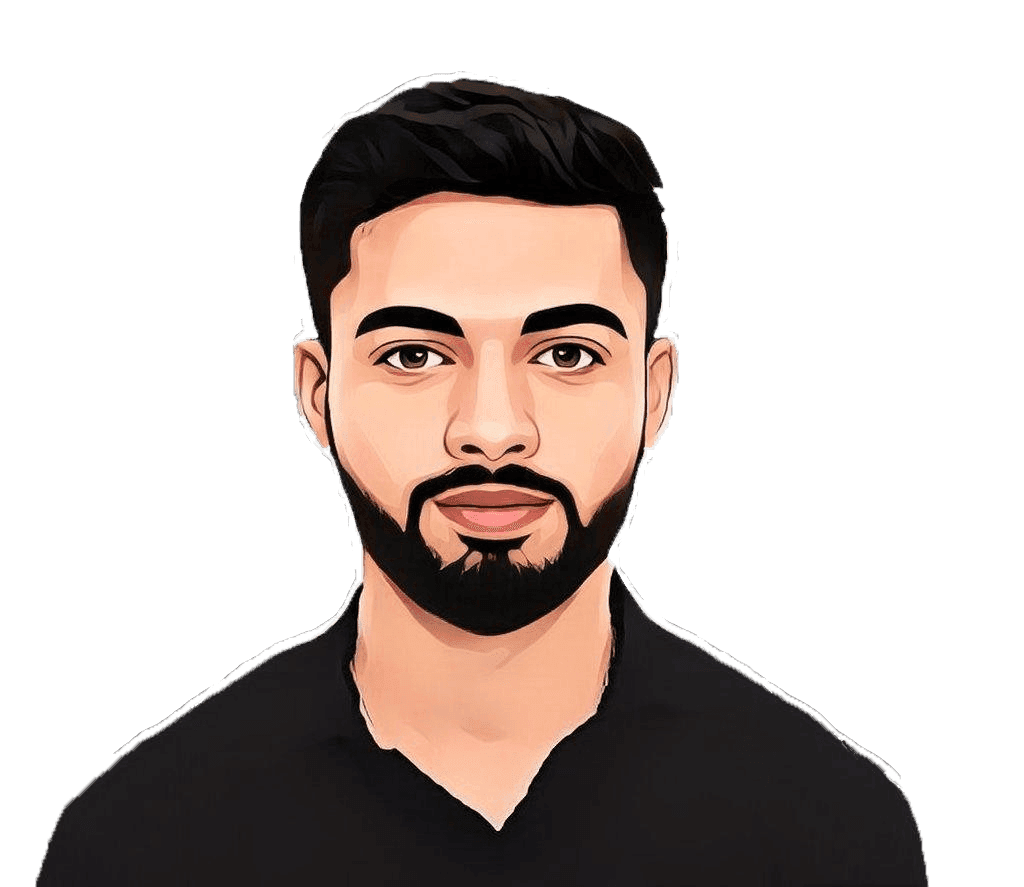
Ravi Kumar
Front End Developer
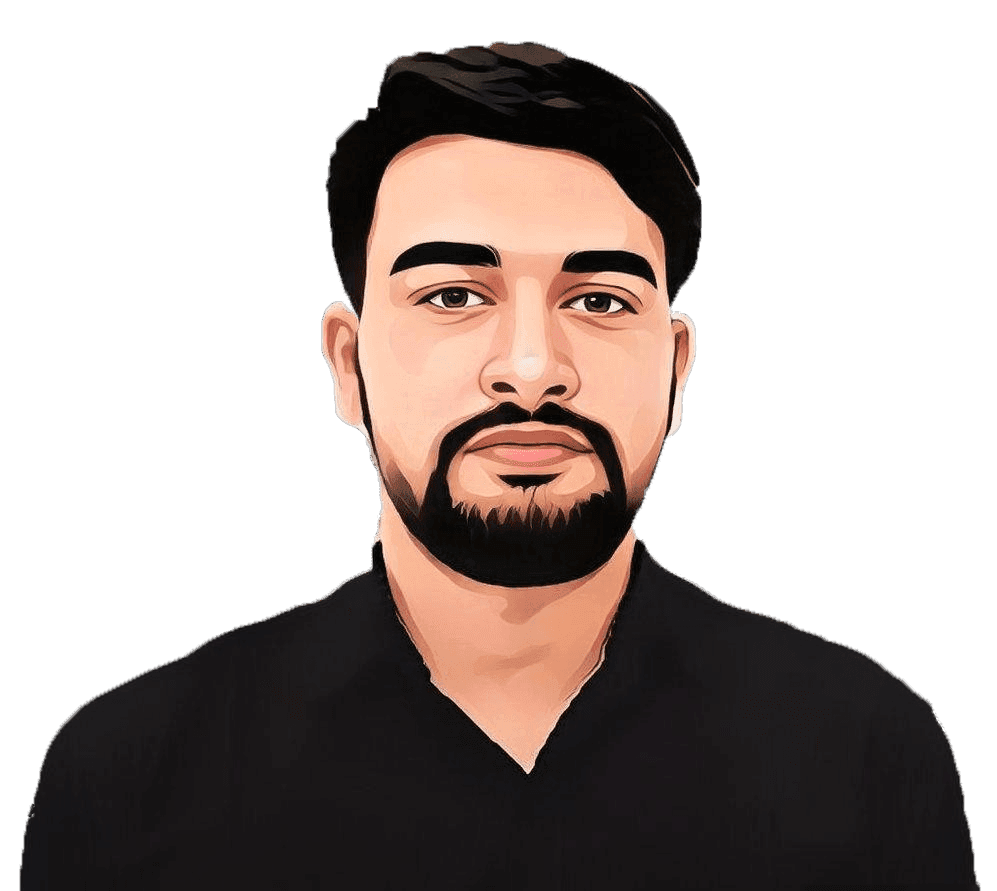
Table of contents
Build with Radial Code
Node.js is a powerful platform for building scalable and high-performance applications using JavaScript. Whether you’re a front-end developer looking to delve into server-side programming or a complete beginner, this guide will walk you through the basics of getting started with Node.js from scratch.
What is Node.js?
Node.js is a runtime environment that allows you to execute JavaScript code on the server side. Unlike traditional server-side languages, Node.js is built on the V8 JavaScript engine (the same engine that powers Google Chrome), and it utilizes an event-driven, non-blocking I/O model, making it efficient and suitable for I/O-heavy applications.
Node is meant for server-side programming, while those browser features are meant for client-side programming.
Why Use Node.js?

- Non-blocking I/O: Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient. This means it can handle many requests concurrently without waiting for each one to complete before starting the next.
- JavaScript Everywhere: With Node.js, developers can use JavaScript for both front-end and back-end development, streamlining the development process and reducing the need to switch contexts between languages.
- Large Ecosystem: Node.js has a vast ecosystem of open-source libraries available through npm (Node Package Manager), which can significantly accelerate development.
Prerequisites
Before diving into Node.js, ensure you have the following:
- Basic Understanding of JavaScript: Node.js uses JavaScript, so familiarity with the language will be helpful.
- A Code Editor: Any code editor like VSCode, Sublime Text, or Atom will work.
- Command Line Interface (CLI): You’ll need to interact with your terminal or command prompt to run Node.js commands.
Step 1: Install Node.js
- Download and Install: Visit the official Node.js website and download the installer for your operating system. The installer will include both Node.js and npm (Node Package Manager), which is essential for managing Node.js packages.
- Confirm Installation: Once installed, launch your terminal and execute:
node -v
npm -v
These commands will display the versions of Node.js and npm if they are installed correctly.
Step 2: Create Your First Node.js Application
- Set Up Your Project:
- Create a new directory for your project:
- Initialize a new Node.js project:
mkdir my-node-app
cd my-node-app
npm init
This command creates a package.json file with default settings, which is essential for man aging project dependencies and metadata.
These commands will display the versions of Node.js and npm if they are installed correctly.
Write Your Application
- Create a JavaScript File
Create a file named app.js in your project directory: - Add Basic Code
Open app.js and add the following code: - Run Your Application
In the terminal, run:
touch app.js
// Load the HTTP module
cost http = require('http');
// Create an HTTP server
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
// Define the port numberconst port = 3000;
// Start the server
server.listen(port, () => {
console.log(`Server running at http://localhost:${port}/`);
});
This code initializes a rudimentary HTTP server that returns the message "Hello, World!" when accessed. when accessed.
node app.js
You should observe the message "Server running at http://localhost:3000/" displayed in the terminal. Open your web browser and navigate to http://localhost:3000/ to see your message.
Understanding Node.js Modules
Node.js employs a module architecture to structure code into reusable elements. Here’s a quick overview:
- Built-in Modules: Node.js includes several built-in modules like http, fs(file system) and path. You can require these modules in your application using require().
- Example of using the fs module to read a file:
- External Modules:
You can install external modules from the npm registry. For example, to install the popular express framework, run:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
npm install express
Then use it in your application:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello from Express!');
});
app.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Here is an image showcasing commonly used core modules in Node.js and their applications:

Understanding Node.js Concepts:
- Modules: Node.js uses modules to organize code. You can use built-in modules (like http) or install external ones using npm.
- Asynchronous I/O: Node.js is non-blocking, which means operations like reading files or making network requests don't block the execution of other code. This allows for high performance and scalability.
- Event Loop: The event loop is the core mechanism of Node.js. It handles asynchronous operations and efficiently manages events.
- NPM: npm is the package manager for Node.js. It facilitates the straightforward installation and management of third-party libraries and packages.
Conclusion
Starting with Node.js is an exciting journey into server-side JavaScript development. By following these steps, you’ve set up a basic Node.js application, learned about modules, and managed dependencies. From here, you can explore more advanced features, and frameworks, and build complex applications.
Feel free to experiment, build projects, and continue learning to make the most of Node.js.
