Chakra UI for Mobile: Building Responsive Apps

Written by
Preeti Yadav
Front End Developer

Anil Kumar
Front End Developer
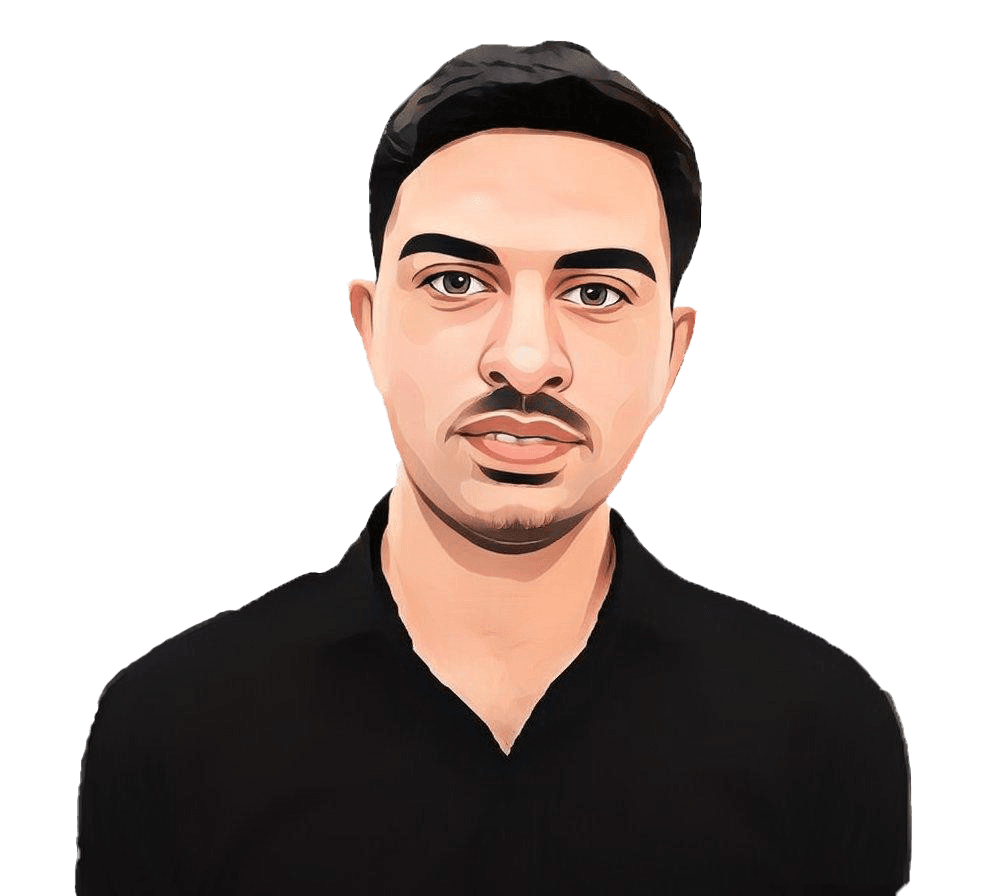
Table of contents
Build with Radial Code
Creating responsive and attractive user interfaces is key in mobile app development, necessitating a strong UI framework for smooth cross-device experiences. Chakra UI, a React component library, simplifies this process with a focus on responsiveness. This blog delves into using Chakra UI to craft adaptive, user-friendly interfaces, detailing how to utilize its features to create scalable apps for various screen sizes. Suitable for both seasoned and new developers, the guide offers insights on maximizing Chakra UI in your projects. Dive into Chakra UI for mobile, where ease meets efficiency in app development. Let's get started!
Understanding Chakra UI
Chakra UI is a set of accessible and composable React components that make it easier to design consistent and responsive user interfaces. With its theming system, style props, and extensive documentation, Chakra UI has become a go-to choice for many developers aiming to streamline the UI development process, exploring its core principles, features, and benefits:

- Composable Components: Chakra UI facilitates UI construction through small, reusable components for assembling complex interfaces.
- Accessibility-First Approach: Emphasizes built-in accessibility and ARIA attributes to create user-friendly apps for those with disabilities.
- Emotion for Styling: Utilizes Emotion for dynamic CSS-in-JS styling and easy theme management within JavaScript.
- Theming System: Offers a flexible theming system with runtime adjustments and a ThemeProvider for consistent application of themes.
- Developer-Friendly API: Features an intuitive, well-documented API for easy learning and quick prototyping, allowing developers to focus on feature creation.
Setting Up Chakra UI for Mobile
Before diving into the details of mobile app development, let's set up a basic React project with Chakra UI. Utilize popular tools like Create React App or Next.js, and integrate Chakra UI as the UI component library. This step lays the foundation for building responsive mobile apps with Chakra UI.

- Project Initialization:
- Create a New React Project: If you don't already have a React project, start by creating one using a tool like Create React App or Next.js. These tools provide a quick and standardized way to set up a new React application.
- Install Chakra UI:
- Install Chakra UI Packages: Use npm or yarn to install the necessary Chakra UI packages. Chakra UI provides a core package ( @chakra-ui/react) and an optional package for icons ( @chakra-ui/react). Install them in your project:
- Chakra Provider Integration:
- Wrap Your App with ChakraProvider: In your src/index.jsor src/index.jsx. file, import ChakraProvider from @chakra-ui/reactand wrap your entire application with it. This provider ensures that Chakra UI components have access to the theme and configuration.
- Start Developing:
- Start the Development Server: With Chakra UI integrated into your project, start the development server and begin building your mobile app with responsive Chakra UI components.
- Explore Chakra UI Components:
- Explore Chakra UI Components: Familiarize yourself with the Chakra UI component library by exploring the documentation. Chakra UI provides a rich set of components, each with its own set of props and customization options.
- Visit the official documentation Chakra UI Documentation.
npx create-react-app my-chakra-mobile-app
cd my-chakra-mobile-app
or for Next.js:
npx create-next-app my-chakra-mobile-app
cd my-chakra-mobile-app
npm install @chakra-ui/react @chakra-ui/icons
or with yarn:
yarn add @chakra-ui/react @chakra-ui/icons
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { ChakraProvider } from '@chakra-ui/react';
import App from './App';
ReactDOM.render(
<ChakraProvider>
<App />
</ChakraProvider>,
document.getElementById('root')
);
npm start
// or with yarn:
yarn start
Responsive Design with Chakra UI
One of the key features of Chakra UI is its support for responsive design out of the box. Learn how to utilize responsive design principles to adapt your app's layout and components based on different screen sizes. Here's a brief overview of how Chakra UI facilitates responsive design:

- Responsive Utility Functions:
- Breakpoints: Chakra UI provides predefined breakpoints for different screen sizes, allowing developers to apply responsive styles based on these breakpoints. This makes it easy to create designs that adapt to small screens, tablets, and larger desktop displays.
- Responsive Styles: Chakra UI offers utility functions that enable developers to apply responsive styles directly in their components. For example, you can use the order property to control the order of flex items on different screen sizes.
- Breakpoint System:
- Customizable Breakpoints: While Chakra UI comes with default breakpoints, developers have the flexibility to customize these breakpoints according to their project's requirements. This adaptability ensures that responsive design can be tailored to specific use cases.
- Media Queries: Chakra UI internally handles media queries, making it convenient for developers to create styles that are applied conditionally based on the screen size. This abstraction simplifies the process of managing responsive design without the need for manual media query configurations.
- Responsive Components:
- Adaptable Components: Chakra UI's component library is designed with responsiveness in mind. Components automatically adjust their styling based on the screen size, providing a consistent user experience across devices.
- Responsive Typography: Chakra UI allows developers to create responsive typography by using the Textcomponent with style props that adapt to different screen sizes. This ensures that text remains readable and visually appealing on various devices.
- Flexibility in Design:
- Grid System: Chakra UI offers a responsive grid system that simplifies the creation of layouts that adapt to different screen sizes. Developers can easily define the number of columns and breakpoints for a responsive grid layout.
- Spacing Utilities: Chakra UI provides responsive spacing utilities, allowing developers to adjust margins and paddings based on screen sizes. This ensures proper spacing between elements on different devices. Example:
- Theming for Responsive Design:
- Dynamic Theming: Chakra UI's theming system extends to responsive design, enabling dynamic changes to the theme based on the user's preferences or the application's requirements. This includes support for dark mode, which can be dynamically toggled for a responsive user experience.
import { Box } from "@chakra-ui/react";
const ExampleComponent = () => (
<Box display={{ base: "block", md: "flex" }} flexDirection={{ base: "column", md: "row" }}>
<Box width={{ base: "100%", md: "50%" }}>First Column</Box>
<Box width={{ base: "100%", md: "50%" }}>Second Column</Box>
</Box>
);
export default ExampleComponent;
import { extendTheme } from "@chakra-ui/react";
const customTheme = extendTheme({
breakpoints: {
sm: "20em",
md: "40em",
lg: "60em",
xl: "80em",
},
});
export default customTheme;
<Heading fontSize={{ base: "2xl", md: "4xl", lg: "6xl" }}>Responsive Heading</Heading>
import { Box, Grid, GridItem } from "@chakra-ui/react";
const ResponsiveGrid = () => (
<Grid
templateColumns={{ base: "repeat(1, 1fr)", md: "repeat(2, 1fr)", lg: "repeat(3, 1fr)" }}
gap={{ base: 4, md: 6, lg: 8 }}>
<GridItem>
<Box p={4} bg="blue.200">Grid Item 1</Box>
</GridItem>
<GridItem>
<Box p={4} bg="green.200">Grid Item 2</Box>
</GridItem>
<GridItem>
<Box p={4} bg="orange.200">Grid Item 3</Box>
</GridItem>
</Grid>
);
export default ResponsiveGrid;
import { ChakraProvider, extendTheme, useColorMode, Button } from "@chakra-ui/react";
const customTheme = extendTheme({
config: {
initialColorMode: "light",
useSystemColorMode: false,
},
colors: {
primary: {
light: "#38B2AC",
dark: "#2C7A7B",
},
secondary: {
light: "#81E6D9",
dark: "#4A5568",
},
},
});
const DynamicThemingExample = () => {
const { toggleColorMode } = useColorMode();
return (
<ChakraProvider theme={customTheme}>
<Button onClick={toggleColorMode}>Toggle Dark Mode</Button>
</ChakraProvider>
);
};
export default DynamicThemingExample;
Mobile-Friendly Components
Chakra UI provides a wide range of pre-built components that are designed with mobile responsiveness in mind. Dive into these components, such as buttons, forms, and navigation elements, and understand how they can be customized and utilized to enhance the mobile user interface. Here's a brief overview of the mobile-friendly components in Chakra UI:

- Buttons:
- Chakra UI offers responsive button components with built-in styling for various use cases.
- Buttons are customizable and come with predefined styles for primary, secondary, and tertiary actions.
- Supports responsiveness out of the box, making it easy to create buttons suitable for different screen sizes.
- Forms:
- Chakra UI provides a suite of mobile-friendly form components, including inputs, checkboxes, radio buttons, and selects.
- Components are designed to be easily customizable and responsive, ensuring a seamless experience on mobile devices.
- Validation and error handling components are available for enhanced form interactions.
- Navigation Components:
- Chakra UI offers navigation components suitable for mobile apps, such as Tabs , Drawer , Navbar
- Components are designed to adapt to smaller screens, providing a user-friendly navigation experience.
- Responsive navigation options enhance the overall mobile app's usability.
- Mobile Modal Components:
- Chakra UI includes modal components optimized for mobile devices, allowing developers to create responsive and accessible modal dialogs.
- Features such as focus trapping enhance the user experience on mobile, preventing distractions from elements outside the modal.
- Alerts and Notifications:
- Chakra UI provides components for alerts, toasts, and notifications that are designed with mobile responsiveness in mind.
- Customizable styles and animations ensure that notifications are visually appealing on smaller screens.
- Responsive behavior allows for unobtrusive yet informative alerts on mobile devices.
Reference: https://chakra-ui.com/docs/components
Theming for Mobile Apps
Theming is a powerful aspect of Chakra UI that allows developers to customize the look and feel of their apps easily. Explor e how to create a theme that aligns with the branding of your mobile app. Understand Chakra UI's color modes, fonts, and spacing utilities to create a visually appealing and cohesive mobile app design. Here's a brief overview of theming for mobile apps in Chakra UI:

- Color Modes:
- Chakra UI introduces color modes that facilitate the implementation of light and dark themes. Users can switch between these color modes, providing a comfortable experience in various lighting conditions and personal preferences.
- Customizable Colors:
- Developers can easily customize the color palette used in their mobile apps. Chakra UI's theming system allows for the definition of primary and secondary colors, ensuring a cohesive and branded visual identity.
- Spacing and Sizing:
- Theming in Chakra UI extends to spacing and sizing configurations. Developers can define default spacing values and sizing scales, ensuring a harmonious layout throughout their mobile app.
- Dynamic Theming:
- Chakra UI supports dynamic theming, enabling developers to define and switch between themes dynamically. This is particularly useful for mobile apps that may need to adapt their visual style based on user preferences or environmental conditions.
Accessibility in Mobile Apps
Ensuring accessibility is a crucial aspect of mobile app development. Discover how Chakra UI promotes accessibility best practices and how you can implement them in your mobile apps. Learn about ARIA roles, focus management, and other accessibility features provided by Chakra UI.
Here's a brief overview of accessibility features in Chakra UI:

- ARIA Roles and Attributes:
- Built-in ARIA Support: Chakra UI components come with built-in support for Accessible Rich Internet Applications (ARIA) roles and attributes. This ensures that the components convey the appropriate semantics to assistive technologies, making the user interface more understandable for users who rely on screen readers or other assistive devices.
- Semantic HTML Elements: Chakra UI encourages the use of semantic HTML elements, contributing to a more accessible document structure. This is essential for screen readers and other assistive technologies to interpret and convey information accurately.
- Focus Management:
- Accessible Focus Styles: Chakra UI provides accessible focus styles out of the box. These focus styles help users with visual impairments or mobility challenges understand which element has focus, improving navigation and interaction within the mobile app.
- Trap Focus and Modals: Chakra UI includes utilities for trapping focus within modal dialogs, ensuring that users who rely on keyboard navigation can seamlessly navigate through the content of the modal without being distracted by elements outside the modal.
- Screen Reader Support:
- Accessible Text and Labels: Chakra UI encourages developers to provide descriptive text and labels for interactive elements, enhancing the experience for users relying on screen readers. Components like buttons and form inputs can be easily customized to include meaningful labels.
- Live Regions: Chakra UI supports the creation of live regions, allowing developers to announce dynamic content changes to screen readers. This is particularly useful for notifying users about updates or changes in the app's state.
- Keyboard Navigation:
- Support for Keyboard Navigation: Chakra UI components are designed to be accessible via keyboard navigation. Users who cannot rely on a mouse or touchscreen can navigate through the app using standard keyboard controls, ensuring a seamless experience.
- Skip to Content: Chakra UI supports the implementation of a "skip to content" link, enabling users to bypass repetitive content and directly access the main content of the mobile app. This is beneficial for users who rely on screen readers and want to quickly navigate to the core information.
- Documentation and Guidelines:
- Accessibility Documentation: Chakra UI provides comprehensive documentation on accessibility best practices. Developers can refer to the documentation to understand how to implement accessible designs, use ARIA roles appropriately, and make their mobile apps inclusive.
- Community Support: The Chakra UI community actively discusses and promotes accessibility practices. Developers can seek guidance and share experiences related to building accessible mobile apps with Chakra UI.
Real-World Example: Building a Responsive Mobile App
To solidify the concepts discussed, let's walk through a real-world example of building a responsive mobile app using Chakra UI. Cover topics such as navigation, data fetching, and state management to demonstrate how Chakra UI can be seamlessly integrated into a mobile app project. Let's outline a brief overview of the real-world example of building a responsive mobile app using Chakra UI:

- Navigation:
- Responsive Navigation: Implement responsive navigation using Chakra UI's components like Drawer or Tabs to create a user-friendly navigation structure for mobile devices.
- Data Fetching:
- Fetch Data Dynamically: Simulate real-world data fetching by incorporating APIs or mock data into your mobile app.
- State Management:
- Manage State: Implement state management to handle dynamic and interactive elements within the app. Showcase how to use React state or a state management library compatible with Chakra UI.
- Building Sample Screens:
- Create Multiple Screens: Demonstrate the creation of multiple screens within the app, each showcasing different Chakra UI components and responsive design features.
- Interactivity: Implement interactive elements, such as buttons and form submissions, to highlight the seamless integration of Chakra UI components.
- Testing and Debugging:
- Test Responsiveness: Use browser developer tools to test the app's responsiveness on various device sizes.
- Debugging Techniques: Address common challenges in responsive design and provide debugging tips for layout adjustments and styling issues.
Conclusion
In conclusion, Chakra UI has proven to be more than a React component library; it's a dynamic toolkit that empowers developers to shape the future of mobile user experiences. As we venture forward, armed with the knowledge gained from this exploration, let's continue to leverage Chakra UI's capabilities, push the boundaries of responsive design, and craft mobile apps that not only meet but exceed user expectations. For more in-depth information and updates on Chakra UI, visit the official website at Chakra UI. Additionally, explore further insights and resources at Radialcode.
