Choosing the Right Styling Approach: Sass vs CSS vs Tailwind
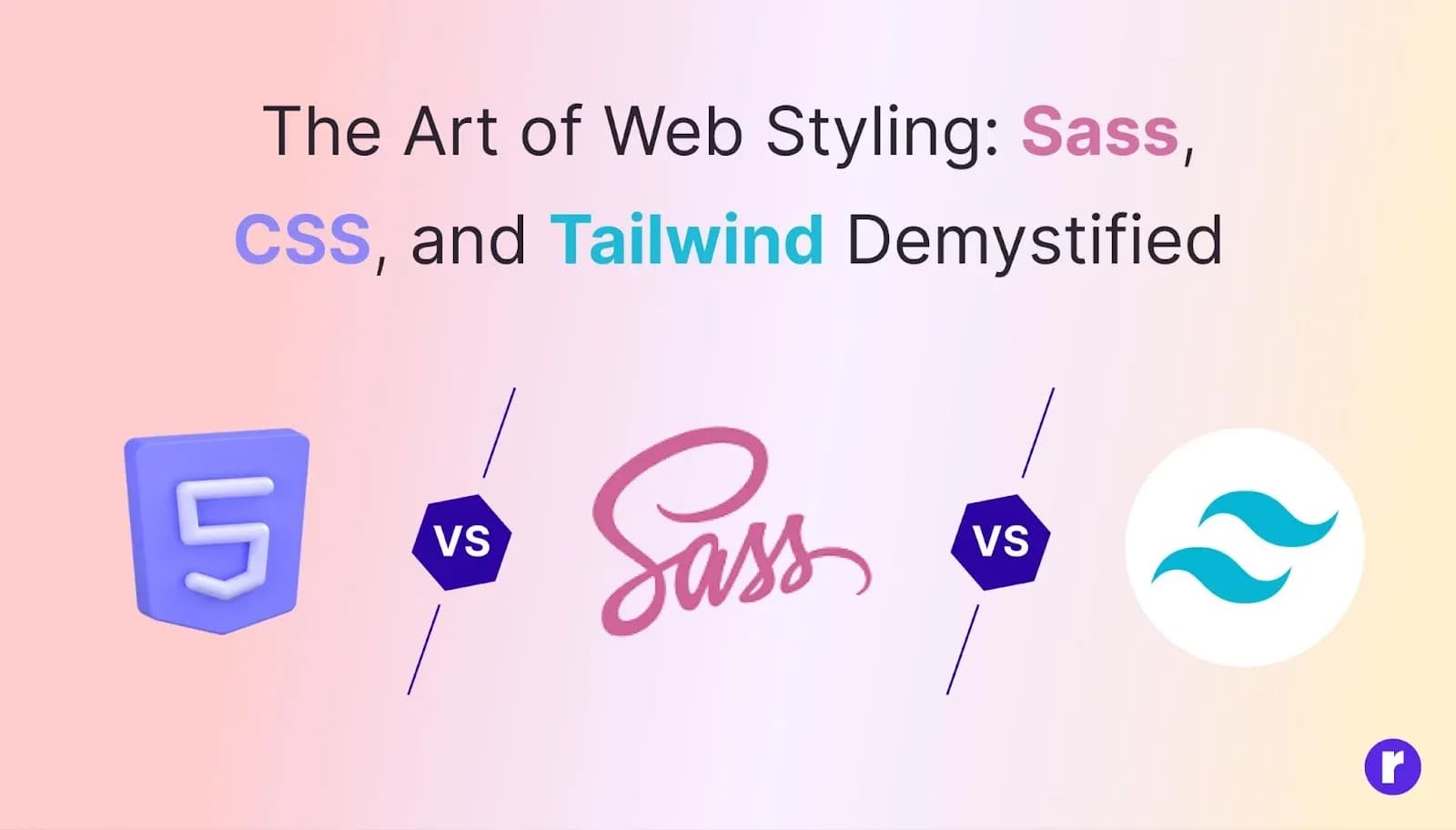
Written by
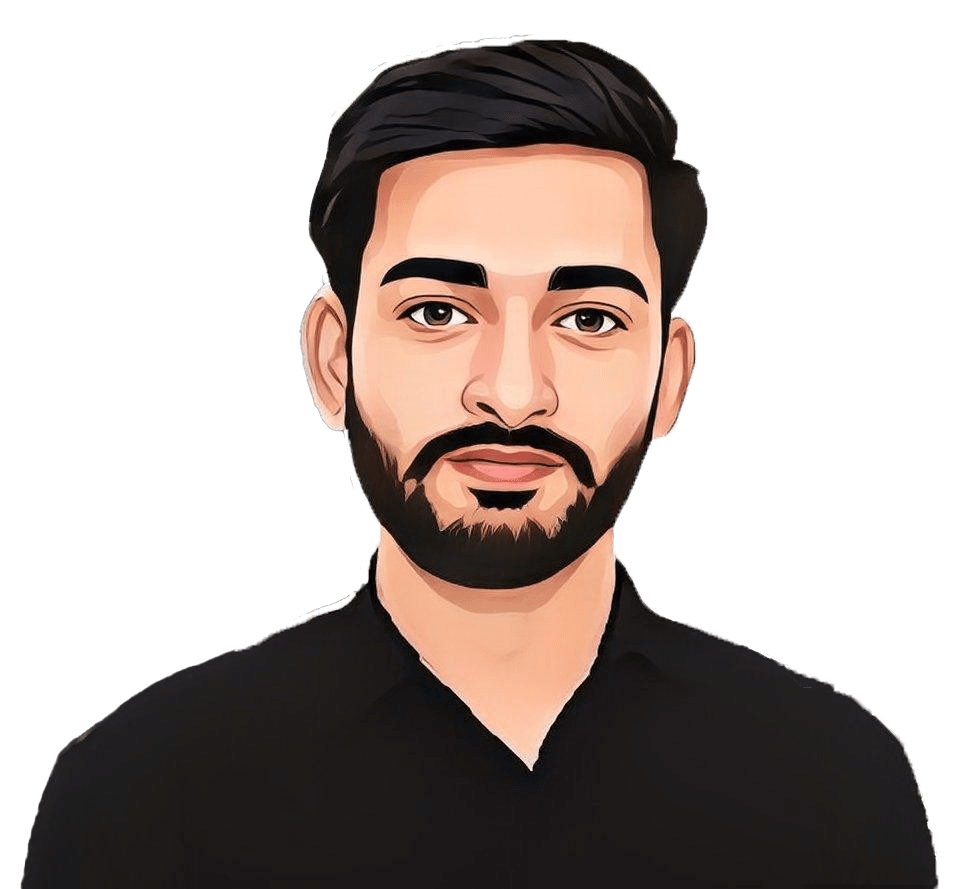
Naveen Ninu
Front End Developer
Table of contents
Build with Radial Code
In the ever-evolving world of web development, styling plays a crucial role in creating visually appealing and user-friendly interfaces. Among the myriad tools and frameworks available, Sass, CSS, and Tailwind CSS are powerful options for crafting elegant and maintainable styles. Let's delve into the art of web styling and demystify these essential tools.
Cascading Style Sheets (CSS)

1.1 Definition:-
- Cascading Style Sheets (CSS) form the cornerstone of web design, a language that transforms HTML elements into visually appealing, harmonious web pages.
1.2 Basic Syntax:-
- CSS operates on a syntax of selectors, properties, and values, acting as the stylist’s code to dictate a website's font, layout, and color harmonies.
/* CSS Comment: This is a stylesheet example */
/* Selector and Block with Properties and Values */
body {
font-family: 'Arial', sans-serif;
font-size: 16px;
line-height: 1.5;
color: #333;
background-color: #f4f4f4;
}
/* Descendant Selector */
h1 {
color: #3498db;
}
/* Class Selector */
.button {
display: inline-block;
padding: 10px 20px;
background-color: #2ecc71;
color: #fff;
text-decoration: none;
border-radius: 5px;
}
/* Pseudo-class Selector */
.button:hover {
background-color: #27ae60;
}
/* ID Selector */
#header {
text-align: center;
background-color: #333;
color: #fff;
padding: 20px;
}
/* Universal Selector */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
1.3 Box Model:-
- Imagined as a digital sculptor’s toolkit, CSS encapsulates the box model content, padding, border, and margin meticulously determining the visual structure and spatial relationships of elements on a webpage.
1.4 Selectors:-
- CSS provides an arsenal of selectors, from broad strokes like elements to pinpoint precision with class, ID, or attribute selectors.
/* Pseudo-class Selector */
button:hover {
background-color: #2ecc71;
}
1.5 Inheritance and Specificity:-
- As styles cascade through the web page, the laws of specificity govern, ensuring order and consistency in the visual narrative.
Benefits of Using CSS

Separation of Concerns:
CSS separates the structure (HTML) from the presentation, promoting a cleaner and more maintainable codebase.
Consistent Styling:
Allows for consistent styling across a website or application.
Responsive Design:
Supports the creation of responsive layouts for various devices and screen sizes.
Browser Compatibility:
Helps ensure consistent rendering across different web browsers.
Ease of Maintenance:
Enables easy updates and modifications to the visual appearance of a website.
Accessibility:
Provides tools and techniques to enhance accessibility for users with disabilities.
Syntactically Awesome Stylesheets (Sass)

2.1 Definition:-
- Syntactically Awesome Stylesheets, or Sass, elevate the artistry of styling by introducing a layer of abstraction, offering variables, nesting, mixins, and more for a more efficient and maintainable design process.
2.2 Variables:-
- Sass allows the declaration and reuse of variables, akin to a palette of consistent colors that bring coherence to the design.
$primary-color: #3498db;
body {
background-color: $primary-color;
}
2.3 Nesting:-
- The CSS nesting module enhances the readability, modularity, and maintainability of stylesheets by eliminating repetitive selectors. This optimization not only improves file size but also ensures parsing directly by the browser, distinguishing it from preprocessors like Sass. Moreover, the specificity of the & nesting selector resembles that of the :is() function, being determined by the highest specificity in the related selector list
/* Without nesting selector */
parent {
/* parent styles */
child {
/* child of parent styles */
}
}
/* With nesting selector */
parent {
/* parent styles */
& child {
/* child of parent styles */
}
}
/* the browser will parse both of these as */
parent {
/* parent styles */
}
parent child {
/* child of parent styles */
}
2.4 Mixins:-
Think of mixins as reusable design patterns snippets of code that can be applied across different elements, streamlining the development process.
- Custom properties --small-window, --medium-window and --large-window define the breakpoints for different window sizes.
- Media queries are used to apply styles based on the window size.
@mixin border-radius($radius) { border-radius: $radius;
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
}
.box {
@include border-radius(5px);
background-color: #ccc;
}
/* Define mixins for window size */
:root {
--small-window: 600px;
--medium-window: 900px;
--large-window: 1200px;
}
/* Usage example */
@media (max-width: var(--small-window)) {
/* Styles for small window */
}
@media (min-width: var(--small-window)) and (max-width: var(--medium-window)) {
/* Styles for medium window */
}
@media (min-width: var(--medium-window)) and (max-width: var(--large-window)) {
/* Styles for large window */
}
@media (min-width: var(--large-window)) {
/* Styles for extra-large window */
}
2.5 Functions and Operators:-
Sass introduces mathematical operations, functions, and control directives, enabling dynamic and conditional styling, akin to the artist's brushstrokes adapting to the canvas.
/* Function definition */
@function add($a, $b) {
@return $a +$b;
}
/* Using the function */
$sum: add(5, 3);
.result {
value: $sum; // Output: 8
}
/* Arithmetic operators */
$width: 100px;
$padding: 20px;
$margin: $width + $padding;
.element {
width: $width;
padding: $padding;
margin: $margin; // Output: 120px
}
/* Comparison operators */
$number1: 10;
$number2: 5;
.is-equal: $number1 ==$number2; // Output: false
.is-not-equal: $number1 !=$number2; // Output: true
.is-greater-than: $number1 >$number2; // Output: true
.is-less-than: $number1 < $number2; // Output: false
2.6 Control Directives:-
- @if, @else, @for, @each, @while
- Conditional and looping constructs in Sass
@for $i from 1 through 3 {
.col-#{$i} {
width: 100px * $i;
}
}
2.7 Importing and Partials:-
- Breaking styles into smaller, manageable files
- Importing partials
Usage and Compilation
File Extension:
- Sass files typically use the .scss extension.
Compilation:
- Sass code needs to be compiled into standard CSS for web browser interpretation.
- This can be done using a Sass compiler or integrated into build tools like Webpack, Gulp, or Grunt.
- Compiled CSS files can then be linked in HTML documents.
Benefits of Using Sass
Code Maintainability:
Organizes and modularizes code, improving maintainability.
Reusable Code:
Variables, mixins, and functions promote the reuse of code snippets.
Improved Readability:
Nested syntax enhances the structure and readability of stylesheets.
Dynamic Styling:
Functions and control directives allow for dynamic and responsive styles.
Easier Debugging:
Provides more advanced features that can aid in debugging and development.
In summary, Sass is a powerful tool that enhances the capabilities of CSS, making stylesheets more maintainable, readable, and flexible. Its features are particularly beneficial for large and complex web development projects.
Optimize Your Styling Workflow: Compare Sass, CSS, and Tailwind to Find the Perfect Fit. Learn more.
Tailwind (CSS)

3.1 Definition:-
- Tailwind CSS, a revolutionary utility-first framework, turns styling into a declarative and efficient process by providing a comprehensive set of utility classes.
3.2 Utility Classes:-
- Tailwind’s utility classes function as building blocks, directly embedded in HTML, offering unprecedented speed and flexibility in crafting layouts, typography, and more.
@mixin border-radius($radius) { border-radius: $radius;
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
}
.box {
@include border-radius(5px);
background-color: #ccc;
}
3.3 Configuration:-
- Like a malleable material, Tailwind is customizable to fit specific project needs through a configuration file, ensuring a harmonious blend of utility and personalization.
// tailwind.config.js
module.exports = {
theme: {
extend: {
colors: {
primary: '#3498db',
},
},
},
variants: {},
plugins: [],
};
3.4 Plugins:-
- Tailwind extends its capabilities through plugins, adding versatility to its repertoire, similar to an artist expanding their toolkit to achieve diverse effects.
// tailwind.config.js
module.exports = {
theme: {
extend: {},
},
variants: {},
plugins: [
require('@tailwindcss/typography'),
],
};
3.5 Pros and Cons:-
- Pros and Cons: Tailwind's efficiency comes with a learning curve and potential file size trade-offs, presenting a choice between speed and ease in web development.
<!-- Tailwind Pros Example -->
<div class="bg-green-300 p-2">
Tailwind makes it easy to create consistent and responsive designs.
</div>
<!-- Tailwind Cons Example -->
<div class="bg-red-500 text-white p-2">
While Tailwind is efficient, it may have a learning curve, and using many utility classes can increase file size.
</div>
Benefits of Using Tailwind

Rapid Development:
Tailwind's utility-first approach allows for quick and efficient development by applying pre-defined classes directly in the HTML, speeding up the styling process..
Consistent Design:
With a comprehensive set of utility classes, Tailwind ensures a consistent and cohesive design language throughout the project, reducing the need for custom styles for each element.
Flexibility and Customization:
Tailwind is highly customizable, enabling developers to tailor the framework to specific project requirements while maintaining the utility-first methodology.
Responsive Design:
Tailwind provides responsive utility classes, making it easy to create designs that adapt to different screen sizes, contributing to a seamless and responsive user experience.
Efficient Debugging and Maintenance:
Tailwind's clear and intuitive naming conventions in utility classes simplify debugging, and the utility-first approach often results in more maintainable code, particularly for smaller to medium-sized projects.
Conclusion
Learning Sass, CSS, and Tailwind helps you become an expert in designing websites that not only look great but also work well. It's like being an artist with tools to create stunning and functional web pages. Whether you prefer Sass for organized coding, CSS for classic styling, or Tailwind for quick and flexible designs, mastering these tools boosts your web design skills. Just like an artist choosing the right brush, picking the best styling tool is crucial for creating awesome websites. So, whether you dive into Sass for flexibility, stick to CSS for basics, or embrace Tailwind for efficiency, each choice adds its own touch to your web projects.

Keep Reading
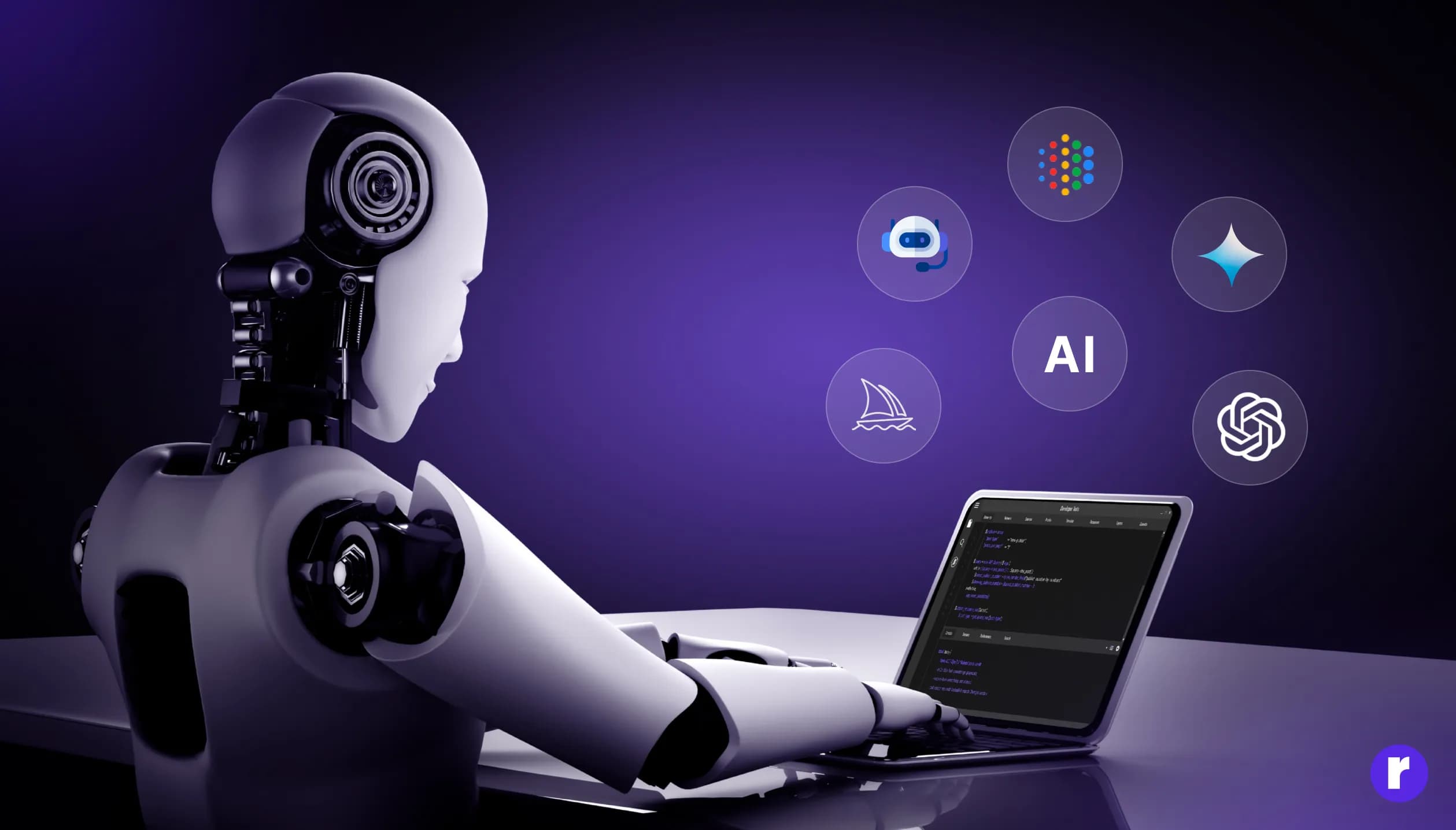
Anshul Poonia
Feb 14, 2025
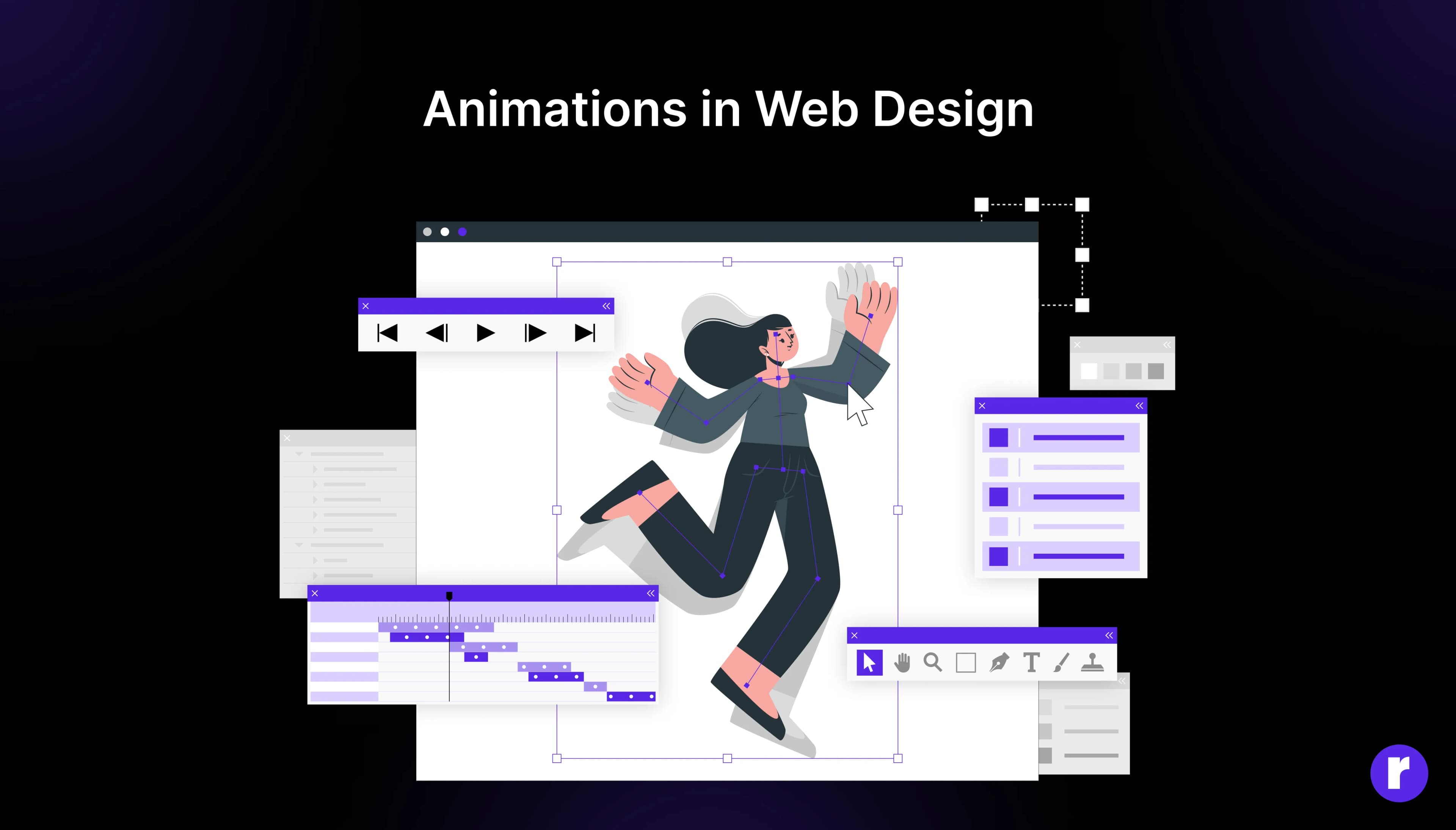
Sanjay Jyani
Feb 14, 2025

Sanjay Jyani
Feb 14, 2025
Stay up to date with all
news & articles.
Email address