Class vs. Functional Components in React: A Comprehensive Comparison

Written by
Ravi Kumar
Front End Developer
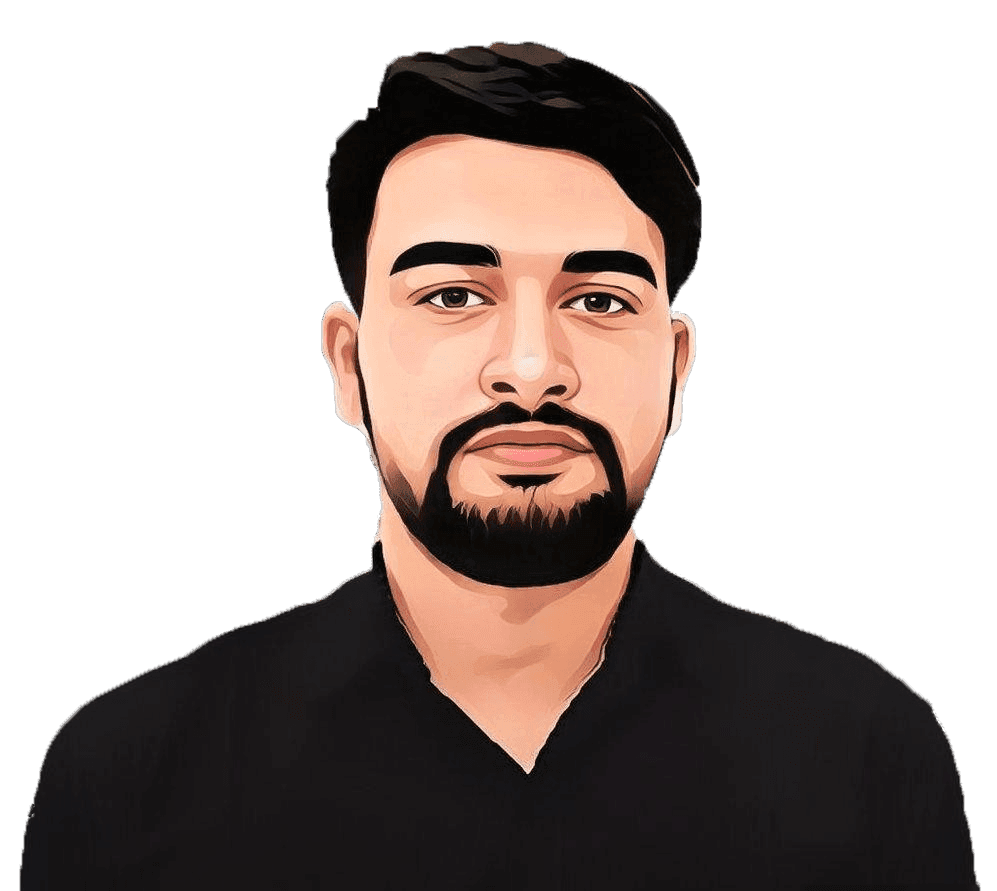
Palvi Tiwari
Front End Developer
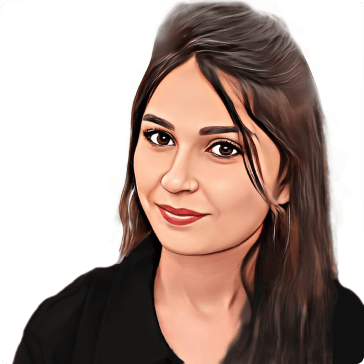
Table of contents
Build with Radial Code
React has become the go-to library for building modern web applications, offering a component-based architecture that allows developers to create reusable and modular UI components. Among the key concepts in React are components, and there are two primary types: Class Components and Functional Components. Understanding the differences between these two types is crucial for making informed choices in React development.
Introduction to Class Components
Class components were the standard way to define components in React before the introduction of hooks in version 16.8. These components are ES6 classes that extend from React.Component and must include a render() method that returns JSX.
Example of a Class Component:
import React, { Component } from 'react';
class Greeting extends Component {
render() {
return Hello, {this.props.name}!
;
}
}
export default Greeting;
Key Features of Class Components:
- State Management: Class components can manage internal state using this.state and update it using this.setState().
- Lifecycle Methods: Class components have access to various lifecycle methods, such as componentDidMount, componentDidUpdate, and componentWillUnmount, allowing developers to perform side effects at different stages of a component’s lifecycle.
- Ref Handling: Refs are typically handled using React.createRef() in class components, providing a way to access DOM elements directly.
Introduction to Functional Components
Functional components are stateless components that were initially introduced as a simpler way to write components that only render UI based on props. With the introduction of hooks, functional components acquired the capability to manage state and side effects, bringing them on par with class components in terms of functionality.
Example of a Functional Component:
import React from 'react';
function Greeting({ name }) {
return Hello, {name}!
;
}
export default Greeting;
Key Features of Functional Components:
- Hooks: With hooks like useState, useEffect, useContext, and others, functional components can manage state, perform side effects, and tap into React’s lifecycle without needing a class structure.
- Simplified Syntax: Functional components are simpler and easier to write, especially for smaller components. They also avoid the complexity of this bindings that are inherent to class components.
- Improved Performance: Functional components generally offer better performance because they do not require the overhead of class inheritance and the methods that come with it.
State Management: Class vs. Functional Components

Comparison:
- Class Components: Involve the use of the this keyword, often making them more verbose, particularly for simpler components.
- Functional Components: Offer a more concise syntax and avoid the confusion of this bindings. With hooks, they provide a more modern approach to managing state.
Lifecycle Methods: Class vs. Functional Components
Class components have specific lifecycle methods that can be overridden to execute code at various stages:
componentDidMount() {
// Runs after the component is mounted
}
In functional components, hooks like useEffect can be used to mimic lifecycle methods:
import React, { useEffect } from 'react';
function Example() {
useEffect(() => {
// Runs after the component is mounted
}, []);
return Example Component;
}
Comparison:
- Class Components: Offer explicit lifecycle methods that are easy to understand for those familiar with traditional object-oriented programming.
- Functional Components: useEffect can replicate lifecycle behavior and offers more flexibility by allowing multiple effects to be declared within a single component.
Comparing Class Components and Functional Components
Feature |
Class Components |
Functional Components |
---|---|---|
Definition |
A JavaScript class that extends React.Component and includes a render() method which returns a JSX element. |
A javaScript function that returns a JSX element |
Syntax |
ES6 class syntax |
JavaScript function |
State Management |
Managed with this.state and this.setState() |
Managed with useState and other hooks |
Props |
Props are accessed through this.props |
Props are received as a parameter to the function |
Lifecycle Methods |
Built-in lifecycle methods |
Handled with useEffect and other Hooks |
Context API |
Access via contextType or Consumer |
Access via useContext |
Performance |
Slightly lower due to overhead |
Generally faster |
Code Size |
More verbose |
More concise |
Function vs Class: Lifecycle methods
Here's a table showing the equivalent of class component lifecycle methods using React Hooks:
Class Components |
Functional Components |
---|---|
componentDidMount |
useEffect with empty dependency array ([]) |
componentDidUpdate |
useEffect with a dependency array containing state/prop values to watch for changes |
componentWillUnmount |
useEffect with a cleanup function returned |
shouldComponentUpdate |
React.memo or useMemo hook |
getDerivedStateFromProps |
useMemo or useEffectto update the state based on props |
getSnapshotBeforeUpdate |
There is no direct hook equivalent but can be achieved with refs |
When to Use Class Components
Class components are still relevant, particularly in legacy codebases where refactoring to functional components is not feasible. They are also beneficial when you need to handle complex state management or lifecycle scenarios that can be more intuitive with explicit lifecycle methods.
When to Use Functional Components
Functional components are the preferred choice in modern React development due to their simplicity and the power of hooks. They are ideal for new projects, smaller components, and scenarios where you want to take advantage of React's latest features like concurrent mode, suspense, and more.
Conclusion
While both class and functional components are powerful tools in React, functional components, enhanced by hooks, have become the preferred standard due to their simplicity, flexibility, and performance benefits. However, class components remain an important part of React’s history and are still widely used in many codebases. Understanding both allows you to make the best choice for your project’s needs.
As React continues to evolve, it’s crucial to stay updated on best practices and new features, ensuring that your applications are built with the most efficient and maintainable patterns available.
Are you interested in coding? We're here to help!
