Creating Sliders with Slick and Swiper in HTML: A Comprehensive Guide

Written by
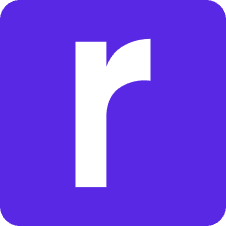
Radial Code
IT Services, Education & Consultancy
Table of contents
Build with Radial Code
Slick slider
Slick Slider is a versatile carousel plugin known for creating responsive sliders with customizable content like images, text, and videos. It offers extensive features including animation settings, autoplay, and navigation controls like arrows, dots, and thumbnails. Slick Slider prioritizes accessibility, ensuring a smooth user experience across devices and for users of assistive technologies, making it an ideal choice for dynamic website sliders.
Swiper Slider
Swiper Slider is a touch-enabled slider known for its seamless transitions and high performance in both mobile and web applications. It's versatile, supporting slides, carousels, and galleries with features like parallax effects and lazy loading. Developers appreciate its flexibility for customization via CSS and JavaScript, making it suitable for diverse design needs.
Both Slick Slider and Swiper Slider are popular choices for developers looking to enhance user interfaces with interactive and responsive slideshows.
How to setup Slick
Including Libraries
Slick Slider, you'll need to include their CSS and JavaScript files. You can either download these files or use a CDN.

Include the Slick CSS and JS files in your HTML:
Initialize Slick Using cdn/npm/yarn/bun
<!-- Slick CSS -->
<link rel="stylesheet" type="text/css"
href="https://cdnjs.cloudflare.com/ajax/libs/slick-carousel/1.8.1/slick.css" />
<link rel="stylesheet" type="text/css"
href="https://cdnjs.cloudflare.com/ajax/libs/slick-carousel/1.8.1/slick-theme.css" />
<!-- Slick JS -->
<script type="text/javascript"
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js">
</script>
<script type="text/javascript"
src="https://cdnjs.cloudflare.com/ajax/libs/slick-carousel/1.8.1/slick.min.js">
</script>
Include the Slick CSS and npm install in your React and Next Js:
In your project directory, run the following command to install react-slick and its dependencies:
npm i react-slick
npm i slick-carousel
Open src/index.js or src/App.js and import the Slick CSS files:
import 'slick-carousel/slick/slick.css';
import 'slick-carousel/slick/slick-theme.css';
Creating a Slick Slider

Basic Setup
HTML Structure:
<div class="container">
<div class="slickSlider">
<div class="slider-content mx-2">
<p class="paragraph">Slide 1</p>
</div>
<div class="slider-content mx-2">
<p class="paragraph">Slide 2</p>
</div>
<div class="slider-content mx-2">
<p class="paragraph">Slide 3</p>
</div>
</div>
</div>
CSS Structure:
.container {
max-width: 600px;
}
.paragraph {
font-weight: 700;
font-size: 30px;
font-family: 'Courier New', Courier, monospace;
color: rgb(10, 235, 239);
}
.slider-content {
display: flex !important;
width: 100%;
max-width: 350px;
height: 200px;
border: 1px solid white;
justify-content: center;
align-items: center;
}
Output:

Initialize Slick Slider:
<script>
$('.slickSlider').slick({
dots: true,
infinite: true,
arrows: true,
slidesToShow: 2,
slidesToScroll: 1,
});
</script>
Advanced Features
Custom Navigation:
<div class="slickSlider">
<!-- Slides -->
</div>
<button class="prev-btn">Prev</button>
<button class="next-btn">Next</button>
<script>
$('.slickSlider').slick({
dots: true,
infinite: true,
arrows: false,
slidesToShow: 3,
slidesToScroll: 1,
centerMode:true,
});
$('.prev-btn').click(function () {
$('.slickSlider').slick('slickPrev');
});
$('.next-btn').click(function () {
$('.slickSlider').slick('slickNext');
});
</script>
Output:

Responsive Settings:
<script>
$('.slickSlider').slick({
dots: true,
infinite: true,
autoplay: true,
speed: 300,
autoplaySpeed: 500,
arrows: true,
slidesToShow: 4,
slidesToScroll: 1,
responsive: [
{
breakpoint: 992,
settings: {
slidesToShow: 3,
}
},
{
breakpoint: 768,
settings: {
slidesToShow: 2,
}
},
{
breakpoint: 576,
settings: {
slidesToShow: 1,
}
},
]
});
</script>
How to setup Swiper

Include the Swiper CSS and Js files in your HTML:
<!-- Swiper CSS -->
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css" />
<!-- Swiper JS -->
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
Include the Swiper CSS and npm install in your React and Next Js:
npm i swipe
Creating a Swiper Slider

Basic Setup
HTML Structure:
<div class="container">
<div class="swiper-container ">
<div class="swiper-wrapper">
<div class="swiper-slide">
<p class="paragraph">Swiper Slide 1</p>
</div>
<div class="swiper-slide">
<p class="paragraph">Swiper Slide 2</p>
</div>
<div class="swiper-slide">
<p class="paragraph">Swiper Slide 3</p>
</div>
</div>
<!-- Add Pagination -->
<div class="swiper-pagination"></div>
<div class="swiper-button-next"></div>
<div class="swiper-button-prev"></div>
</div>
</div>
CSS Structure:
.container {
height: 250px;
max-width: 1000px;
transform: translateY(130%);
position: relative;
overflow: hidden !important;
}
.paragraph {
font-weight: 700;
font-size: 40px;
font-family: 'Courier New', Courier, monospace;
color: rgb(10, 235, 239);
}
.swiper-slide {
display: flex !important;
width: 100%;
max-width: 490px;
height: 200px;
border: 1px solid white;
justify-content: center;
align-items: center;
}
.swiper-pagination-bullet {
background: white !important;
opacity: 0.5 !important;
}
.swiper-pagination-bullet-active {
background: white !important;
opacity: 1 !important;
}
Output:

Initialize Swiper:
<script>
var swiper = new Swiper('.swiper-container', {
slidesPerView: 2,
spaceBetween: 30,
dots: true,
autoplay: {
delay: 2000,
},
loop: true,
pagination: {
el: '.swiper-pagination',
},
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
});
</script>
Advanced Features
Thumbnails Navigation:
<div class="swiper-container gallery-top">
<div class="swiper-wrapper">
<!-- Slides -->
</div>
</div>
<div class="swiper-container gallery-thumbs">
<div class="swiper-wrapper">
<!-- Thumbnails -->
</div>
</div>
<script>
var galleryThumbs = new Swiper('.gallery-thumbs', {
spaceBetween: 10,
slidesPerView: 4,
freeMode: true,
watchSlidesVisibility: true,
watchSlidesProgress: true,
});
var galleryTop = new Swiper('.gallery-top', {
spaceBetween: 10,
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
thumbs: {
swiper: galleryThumbs,
},
});
</script>
Responsive Settings:
<script>
var swiper = new Swiper('.swiper-container', {
slidesPerView: 3,
spaceBetween: 30,
breakpoints: {
1024: {
slidesPerView: 2,
spaceBetween: 20,
},
600: {
slidesPerView: 1,
spaceBetween: 10,
},
},
});
</script>
Configuration Options
Configuration options are critical for customizing the behavior and appearance of sliders. They allow developers to tailor the functionality of the slider to meet specific needs and enhance user experience. Below is a description of the key configuration options available for Slick Slider and Swiper Slider, which are two popular libraries used for implementing sliders in web projects.

Configuration Option |
Slick Slider |
Swiper Slider |
---|---|---|
autoplay |
Enables automatic scrolling of slides. When set to true, slides will advance automatically. |
An object that enables autoplay functionality, including settings such as delay (time between slide transitions) and other configurations. |
dots / pagination |
Displays navigation dots at the bottom of the slider. These dots indicate the current slide and allow direct navigation to specific slides. |
An object to customize pagination, defining the element for pagination, whether it is clickable, and other related settings. |
arrows / navigation |
Enables navigation arrows on the sides of the slider for manual navigation through the slides. |
An object to enable and customize navigation buttons, specifying elements for the 'next' and 'prev' buttons. |
infinite/loop |
Allows the slider to loop infinitely. When the end of the slides is reached, it will seamlessly start again from the beginning |
Enables continuous loop mode, ensuring seamless transition from the last slide back to the first. |
slidesToShow / slidesPerView |
Specifies the number of slides to display at one time within the viewport. |
Sets the number of slides to display within the viewport simultaneously. |
Slick Slider Example:-
<script>
$('.slickSlider').slick({
dots: true,
infinite: true,
autoplay: true,
speed: 300,
autoplaySpeed: 500,
arrows: true,
slidesToShow: 2,
slidesToScroll: 1,
pouseonhover: true,
cssEase: 'linear',
});
</script>
Swiper slider Example:-
<script>
var swiper = new Swiper('.swiper-container', {
slidesPerView: 2,
spaceBetween: 30,
dots: true,
autoplay: {
delay: 2000,
},
loop: true,
pagination: {
el: '.swiper-pagination',
},
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
});
</script>
Comparing Slick and Swiper
Sure, here's a comparison table between Slick and Swiper sliders, along with some additional context :

Feature |
Description |
Specifications |
Specifications |
---|---|---|---|
Performance |
Generally performs well, but can be affected by large numbers of slides or complex layouts. |
Optimized for performance, with features like lazy loading and hardware acceleration. |
Swiper is known for its performance optimizations, making it suitable for demanding applications and large slide sets. |
Community Support |
Large user base with active community forums and resources available online. |
Well-established community with active support channels and third-party plugins. |
Both Slick and Swiper have strong communities of developers and users, providing assistance, troubleshooting, and additional resources. |
Compatibility |
Works with modern browsers and older versions of Internet Explorer. |
Supports modern browsers and mobile devices, with graceful degradation for older browsers. |
Swiper prioritizes compatibility with modern web standards and devices, ensuring consistent performance across a wide range of platforms. |
Dependencies |
Requires jQuery for core functionality. |
Written in vanilla JavaScript, with no external dependencies. |
Swiper's lack of dependencies simplifies integration and reduces the overhead associated with managing additional libraries like jQuery. |
Popularity |
Widely used in web development projects, with a large number of installations./p> |
Popular choice among developers, with widespread adoption in websites and applications. |
Both Slick and Swiper are trusted solutions for creating sliders, with many websites and projects relying on their functionality and performance. |
Conclusion
Both Slick and Swiper are excellent choices for creating sliders on your website. Your decision will depend on your particular needs and preferences. Slick is great for those who prefer simplicity and jQuery, while Swiper is ideal for those looking for a modern, lightweight solution with advanced features.
