Essential Front-End Development Libraries You Should Know
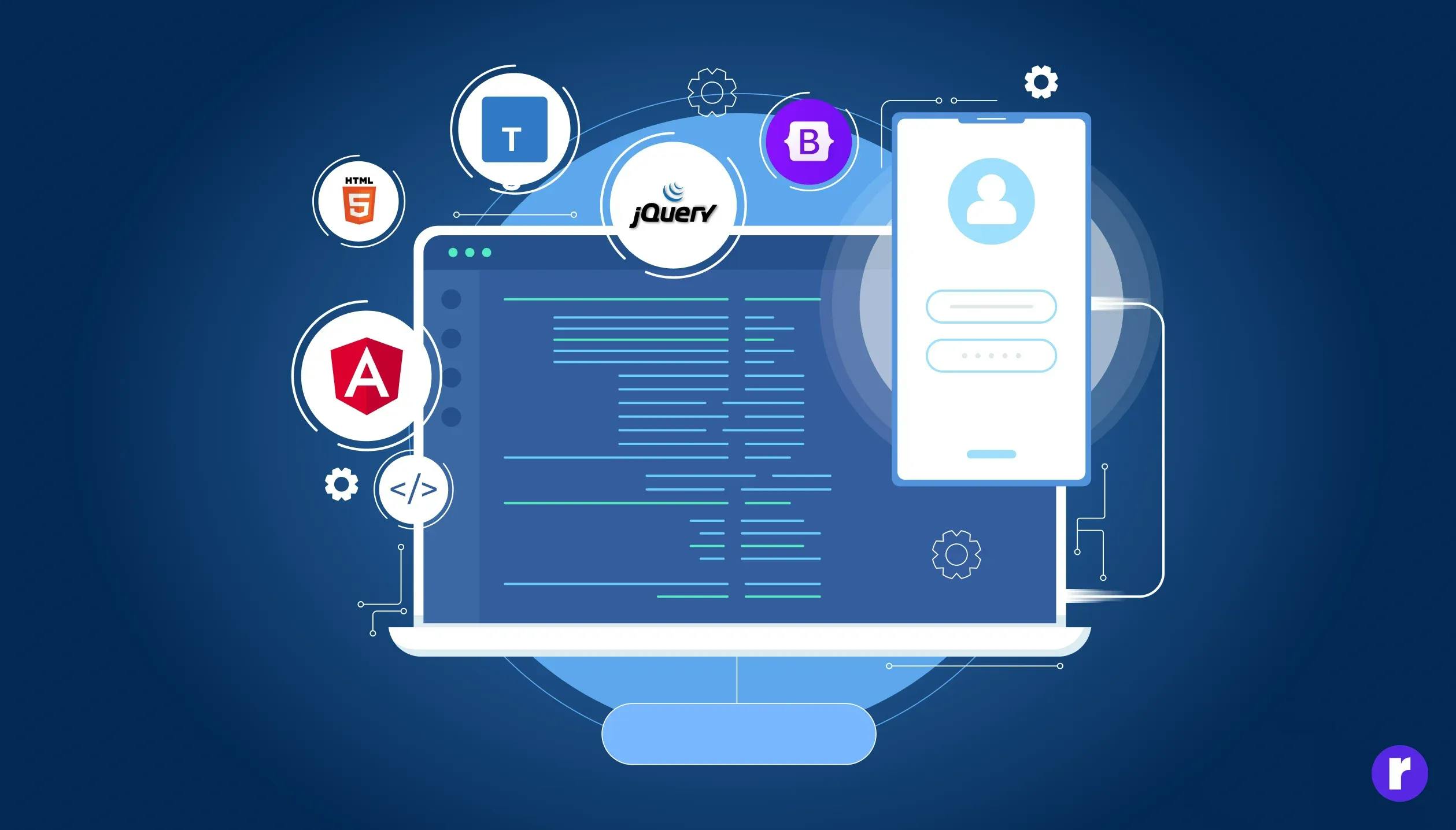
Front-end developers rely on a variety of basic libraries and frameworks to build the user interface and enhance the user experience of websites and web applications. These libraries facilitate tasks such as DOM manipulation, handling user interactions, managing styles, and optimizing performance. Here are some of the fundamental libraries commonly used by front-end developers
jQuery

While less popular today, jQuery remains a widely used library for simplifying DOM manipulation and handling events. It provides a concise syntax for tasks like selecting elements and performing actions on them.
- DOM Manipulation: jQuery allows you to easily select and manipulate HTML elements. For example, you can select elements by their tag name, class, or ID, and then perform actions on them, like changing their content, style, or structure.
- Event Handling: You can attach event handlers to elements, such as click, hover, or submit events, to respond to user interactions with your webpage.
- AJAX: jQuery simplifies making asynchronous HTTP requests using AJAX, which enables you to load data from a server without requiring a full page refresh.
- Animations: You can create smooth animations and transitions on elements using jQuery. This includes fading elements in/out, sliding elements, and more.
- Utilities: jQuery provides a variety of utility functions for common tasks like working with arrays, objects, and performing other operations.
React

Developed and maintained by Facebook, React is a JavaScript library for building user interfaces. It enables the creation of reusable UI components and efficiently updates the DOM through a virtual DOM mechanism.
- Component-Based Architecture: React follows a component-based architecture, where you build user interfaces by creating and composing reusable components. Components are like building blocks that encapsulate a part of the UI and its behavior. This enhances the modularity and maintainability of your codebase.
- Virtual DOM: React employs a Virtual DOM to enhance performance. Rather than directly manipulating the real DOM, React operates on a virtual representation of the DOM and subsequently computes the most efficient updates to the actual DOM. This approach minimizes DOM manipulations, resulting in faster rendering.
- Declarative Syntax: React uses declarative syntax, which means you describe what you want the UI to look like, and React takes care of updating the DOM to match that description. This is in contrast to imperative programming, where you specify how to change the DOM step by step.
- Unidirectional Data Flow: React enforces a unidirectional data flow, where data moves exclusively from parent components to child components. This structure simplifies comprehension of data changes within your application and mitigates issues associated with data mutation, aiding in bug prevention.
- Ecosystem and Community: React has a large and active community, which means there are plenty of resources, libraries, and tools available to help you build robust applications. It's commonly used in conjunction with other libraries and frameworks, such as React Router for routing and Redux for state management.
Angular

Angular, developed by Google, is a comprehensive front-end framework designed for constructing dynamic web applications. It uses declarative templates and supports two-way data binding.
- Component-Based Architecture: Angular is built around a component-based architecture. You create and organize your application as a hierarchy of components, each responsible for a specific part of the user interface. This promotes modularity and reusability in your code.
- Two-Way Data Binding: Angular offers two-way data binding, which allows automatic synchronization of data between the model (JavaScript objects) and the view (HTML). This means that changes in the UI are automatically reflected in the underlying data and vice versa.
- Dependency Injection: Angular features a powerful dependency injection system that helps manage the instantiation and sharing of application services and components. This promotes the creation of maintainable and testable code by facilitating the separation of concerns.
- Directives: Angular provides a variety of built-in directives that extend HTML's capabilities. These directives allow you to create dynamic views and manipulate the DOM easily. For example, ngFor is used for repeating elements, and ngIf for conditional rendering.
- TypeScript: Angular is primarily written in TypeScript, which is a statically typed superset of JavaScript. TypeScript brings strong typing, interfaces, and other modern features to your development, making it easier to catch errors early and maintain large codebases.
Vue.js

Vue is a progressive JavaScript framework that focuses on building user interfaces incrementally. It is renowned for its simplicity and seamless integration into existing projects.
- Progressive Framework: Vue.js is often described as a "progressive" framework because it can be incrementally adopted in your projects. You can use as much or as little of Vue as you need, making it easy to integrate into existing applications or start fresh.
- Component-Based Architecture: Vue.js promotes a component-based architecture, similar to React. You build your application by creating and composing reusable components, which helps keep your code organized and maintainable.
- Reactive Data Binding: Vue.js offers a simple and efficient way to handle data binding. It uses a two-way data binding system, which means changes in the UI automatically update the underlying data and vice versa. This helps streamline the development of interactive and responsive user interfaces.
- Directives: Vue.js provides a set of directives that can be used to declaratively render dynamic content in the DOM. Directives like v-if, v-for, and v-bind (shorthand :) make it easy to manipulate the DOM and create dynamic templates.
- Vue Router and Vuex: Vue Router is the official router for Vue.js, allowing you to build Single Page Applications (SPAs) with client-side navigation. Vuex is a state management library for Vue.js that helps manage the state of your application and facilitates data flow between components.
Bootstrap

This CSS framework, originally developed by Twitter, offers a set of pre-designed UI components and a responsive grid system. It simplifies the process of creating attractive and responsive designs.
- Responsive Grid System: Bootstrap provides a responsive grid system that allows you to create flexible and responsive layouts. It uses a 12-column grid, which can be easily customized to adapt to different screen sizes. This makes it easy to build web pages that look good on various devices, from desktops to mobile phones.
- Pre-Designed CSS Components: Bootstrap includes a wide range of pre-designed CSS components, such as buttons, forms, navigation bars, and typography styles. These components follow a consistent design language, making it quick and easy to create a professional-looking UI without the need for extensive custom CSS.
- JavaScript Plugins: Bootstrap includes a collection of JavaScript plugins that enhance the functionality and interactivity of your web pages. Examples include modal dialogs, carousels, tooltips, and popovers. These can be easily integrated into your project, reducing the need for custom JavaScript development.
- Customization and Theming: Bootstrap allows for easy customization through its SASS variables and build tools. You can customize the framework to match your project's unique design requirements. This makes it suitable for creating both out-of-the-box and highly customized designs.
- Active Community and Documentation: Bootstrap has a large and active community of developers and designers. It is well-documented with extensive guides and examples, making it accessible for developers of all levels. You can find many resources, themes, and templates created by the community to jumpstart your projects.
Sass/SCSS

These are pre-processors for CSS that allow front-end developers to write more maintainable and organized styles. They introduce features like variables, nesting, and mixins.
- Nested Syntax: Sass/SCSS allows you to nest CSS rules within one another, making your code more organized and easier to read. This nesting mirrors the structure of your HTML, which can help maintain a clear and logical relationship between HTML and CSS.
- Variables: Sass/SCSS allows you to define variables to store reusable values such as colors, font sizes, or margins. This promotes consistency in your styles and simplifies maintenance by allowing you to change a value in one place and have it apply throughout your stylesheet.
- Mixins and Functions: Sass/SCSS provides the ability to define reusable pieces of CSS using mixins and functions. Mixins allow you to encapsulate a set of CSS rules and then include them wherever needed. This can reduce code duplication and make your codebase more maintainable.
- Inheritance: In Sass, you can use the @extend directive to create a relationship between selectors, allowing one selector to inherit the properties of another. This can be useful for creating a base set of styles and then extending them for more specific cases.
- Modularity and Imports: Sass/SCSS supports modularity through the use of separate files and the @import directive. You can split your styles into smaller, more manageable files and then import them into a single stylesheet. This can improve code organization and reusability.
Webpack

A popular module bundler and build tool, Webpack helps manage project assets and dependencies. It can compile, minify, and bundle JavaScript, CSS, and other resources.
- Module Bundling: Webpack allows you to bundle various assets and modules, including JavaScript, CSS, images, and more, into a single or multiple output files. This bundling process optimizes the loading and execution of resources in the browser.
- Loaders: Webpack uses loaders to process different types of files, transforming them into modules that can be included in your application. Loaders can handle tasks like transpiling ES6 code with Babel, processing CSS with PostCSS, or optimizing image assets.
- Plugins: Plugins in Webpack offer extended functionality to your build process. They can perform a wide range of tasks, from code minification and environment-specific configuration to generating HTML files and even deploying your application.
- Code Splitting: Webpack supports code splitting, which enables you to split your code into smaller bundles that can be loaded on-demand. This improves initial load times and can lead to better application performance. Dynamic imports are commonly used for code splitting.
- Configuration: Webpack relies on a configuration file (typically webpack.config.js) where you specify entry points, output settings, module rules, and other build-related configurations. This file allows you to customize the build process to suit your project's requirements.
Babel

Babel is a JavaScript compiler that allows developers to write modern JavaScript code and translate it into older versions that are compatible with a broader range of browsers.
- ECMAScript Compatibility: Babel enables developers to write code in the latest ECMAScript syntax and features while ensuring compatibility with older browsers. It does this by transpiling (compiling) modern JavaScript code into a version of JavaScript that older browsers can understand.
- Extensible and Configurable: Babel is highly extensible and configurable. You can customize the Babel configuration to include or exclude specific transformations and plugins, tailoring it to your project's specific needs. This flexibility allows you to adapt Babel to your coding standards and project requirements.
- Presets: Babel provides presets that encapsulate a set of plugins and configurations commonly used together. For example, the "env" preset includes all the necessary plugins to compile to the latest supported JavaScript version. You can use presets to simplify your configuration and save time.
- Plugin System: Babel's plugin system allows you to add or customize transformations for your code. You can use existing plugins or develop custom ones to address specific requirements in your project. This extensibility is a powerful feature that enables you to adapt Babel to various use cases.
- Integration with Build Tools: Babel is often integrated into build tools and development workflows. It's commonly used with tools like Webpack, Gulp, and Grunt to automate the compilation process. This ensures that your modern JavaScript code is transpiled into older versions as part of your development and deployment pipeline.
Lodash

Lodash is a utility library that provides a wide range of helpful functions for manipulating and working with data, making JavaScript development more efficient.
- Utility Functions: Lodash offers a comprehensive collection of utility functions that cover common tasks in JavaScript development. These functions address array manipulation, object manipulation, string operations, and more. Lodash can significantly reduce the amount of custom code you need to write.
- Consistency and Compatibility: Lodash provides a consistent and cross-browser-compatible API. It ensures that your code behaves predictably across different environments and eliminates the need for complex cross-browser workarounds. This consistency is especially valuable for web development.
- Performance: Lodash is well-known for its performance optimizations. The library is highly optimized, and its functions are designed for efficiency. It often outperforms custom implementations of the same tasks and can help improve the performance of your applications.
- Chaining: Lodash supports method chaining, allowing you to chain multiple functions together in a readable and concise manner. This enables you to perform a sequence of operations on data with minimal code, resulting in more maintainable and understandable code.
- Modular Architecture: Lodash has a modular architecture, allowing you to import only the specific functions you need, reducing the size of your bundle and optimizing load times. This modularity ensures that you include only the parts of Lodash that are necessary for your project.
Axios

For handling HTTP requests, Axios is a popular library that simplifies the process of making asynchronous calls to APIs, providing a more intuitive and consistent interface.
- HTTP Requests: Axios simplifies making HTTP requests in JavaScript. It supports all HTTP methods, such as GET, POST, PUT, DELETE, and more, and provides a straightforward API for sending requests to servers or APIs.
- Promise-Based: Axios uses a Promise-based API, making it easy to work with asynchronous operations and manage HTTP requests in a more structured and readable way. You can use .then() and .catch() to handle responses and errors.
- Interceptors: Axios allows you to define request and response interceptors, enabling you to globally handle requests or responses before they are sent or received. This can be useful for tasks like adding authentication headers, handling errors, or logging.
- Cancellation: Axios supports request cancellation, which is beneficial in scenarios where you want to cancel an ongoing HTTP request, especially in situations like user-initiated actions that may change during the request.
- JSON Data Handling: Axios automatically converts JSON data to JavaScript objects and handles the serialization of data when making POST requests, simplifying working with JSON-based APIs.
Redux

Often used in conjunction with React, Redux is a state management library that helps developers manage the state of their application in a predictable and scalable way.
- Centralized State Management: Redux provides a central store to manage the entire state of an application. This centralization simplifies state management and makes it easier to understand how data flows through the application.
- Predictable State Updates: Redux enforces a strict unidirectional data flow, making it predictable and easier to debug. State changes occur through actions, which are dispatched to reducers that specify how the state should change in response to an action.
- Immutable State: Redux encourages the use of immutable data structures. This means that the state cannot be directly modified but must be replaced with a new state object. Immutable state helps prevent unintentional side effects and simplifies tracking changes.
- Middleware: Redux supports middleware, which allows you to extend its capabilities. Common use cases for middleware include logging, asynchronous operations (e.g., AJAX requests), and routing. Middleware can intercept actions and add custom behavior.
- DevTools and Debugging: Redux has a powerful ecosystem of developer tools, including Redux DevTools, which helps visualize and debug the application's state changes and actions. These tools are invaluable for tracking and understanding how the application's state evolves.
These libraries and frameworks are just a starting point for front-end development, and the choice of which to use depends on the specific project requirements and developer preferences. Front-end development continues to evolve, and staying up to date with the latest tools and libraries is essential to create modern, efficient, and user-friendly web applications.
Key Features of Front-End Development Libraries

Front-end development libraries offer various features and tools to simplify the development process. Here are some common characteristics:
- Pre-designed UI Components: Libraries provide a range of UI components like buttons, forms, navigation bars, modals, and more, reducing the need to create these elements from scratch.
- Grid Systems: Many libraries include responsive grid systems for layout management, ensuring that web pages adapt to different screen sizes.
- Typography and Styles: Libraries offer predefined typography settings, color schemes, and styles, maintaining a consistent look and feel throughout the application.
- JavaScript Functionality: Some libraries include JavaScript plugins for interactive features like carousels, modals, tooltips, and more.
- Customizable Themes: Developers can modify and extend the library's styles and components to create unique themes and designs that match their project's requirements.
- Accessibility: Good front-end libraries pay attention to accessibility features, making it easier to create web interfaces that are usable by individuals with disabilities.
Conclusion
In conclusion, front-end development libraries and frameworks play a crucial role in simplifying and enhancing the process of building user interfaces for websites and web applications. These libraries offer a range of features and tools that empower developers to create attractive, responsive, and interactive web experiences. The choice of which library or framework to use depends on project requirements and developer preferences, and it's essential to stay updated with the latest tools and best practices in the ever-evolving field of front-end development.
Key features of front-end development libraries include pre-designed UI components, grid systems, typography and styles, JavaScript functionality, customizable themes, and a focus on accessibility. Some of the prominent libraries and frameworks, as mentioned earlier, include jQuery, React, Angular, Vue.js, Bootstrap, Sass/SCSS, Webpack, Babel, Lodash, Axios, and Redux.
Front-end development continues to evolve, and developers should adapt to new technologies and best practices to create modern, efficient, and user-friendly web applications that meet the needs and expectations of today's digital world.
