Getting Started with React Hooks: A Beginner's Handbook

Written by
Mohit Bishnoi
Front End Developer

Preeti Yadav
Front End Developer

Table of contents
Build with Radial Code
React is an open-source JavaScript library that is commonly used for building user interfaces (UIs) for web applications. It was developed and maintained by Facebook. React empowers developers to craft dynamic and efficient websites. It also facilitates the process of breaking down larger components into sub-components, improving code organization and reusability. React is easily integrated with libraries and tools to manage apps. With the help of the react we can manage the state and routing easily. Its syntax is easy to understand.
A Step-by-Step Guide to Create React App
Install Node.js and npm
React requires Node.js and npm (Node Package Manager) to be installed on your machine. You can obtain and install them from the official Node.js website.
Create a new React app
Now that you have create-react-app installed it, you can use it to create a new React app. Run the following command:
npx create-react-app your-app-name
Replace your-app-name with the desired name for your React app. This command will generate a new directory with the given name and establish a fundamental React project structure within it.
Navigate to the project directory
Move into the newly created app directory using the following command:
cd your-app-name
code .
Start the development server
Once you're inside your project directory, start the development server by running.
npm start
This command will start the development server, and you should see your new React app running at http://localhost:3000 in your browser.
Exploring User Components
In React, components serve as the foundational building blocks of a user interface. There are main two types of the function:
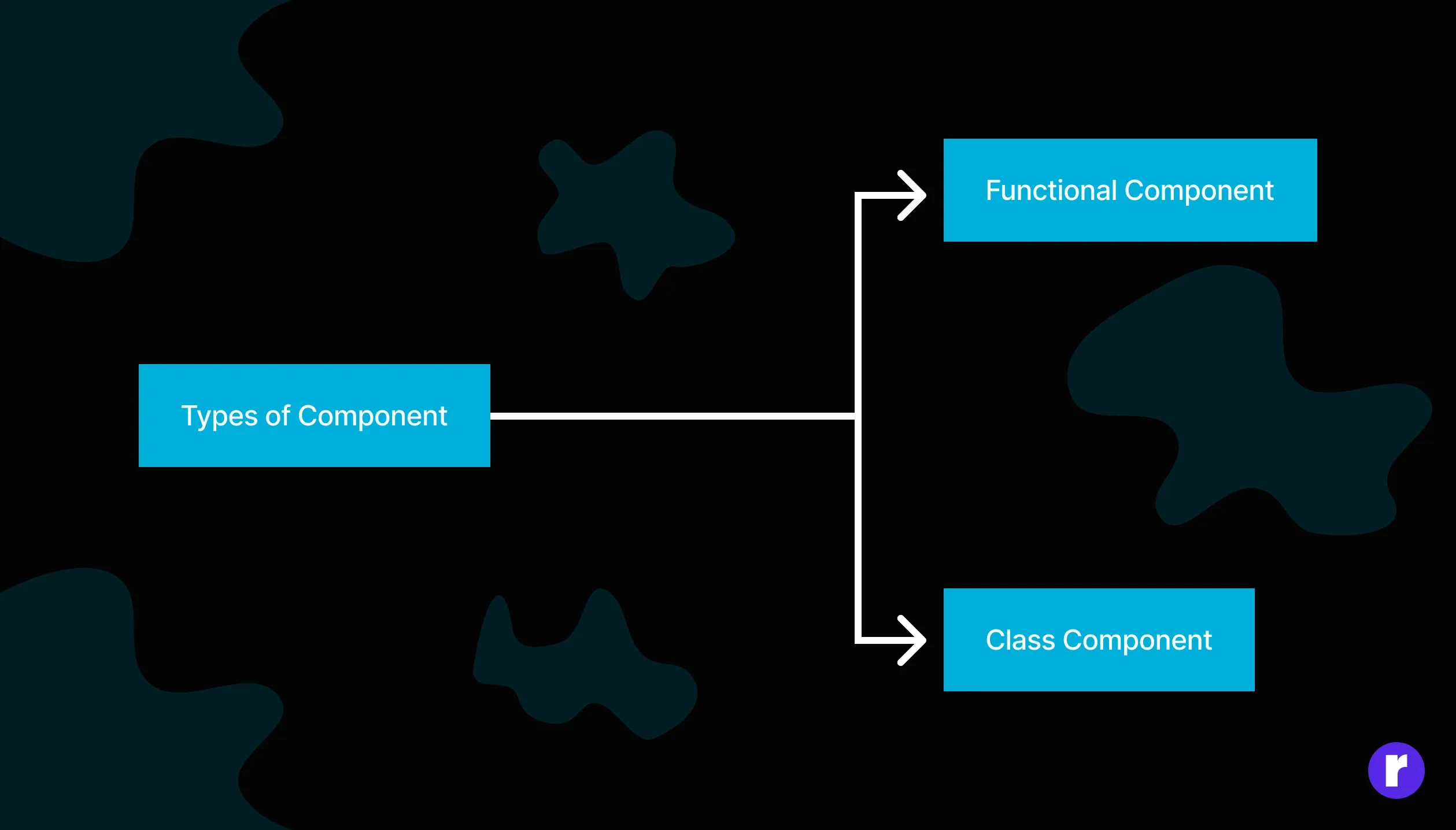
A Deep Dive into Functional Components
Functional components are created with the function keywords. It returns the react element. You can define these functions in JavaScript in two ways: the first involves using the fat arrow function, and the second simple function keyword.
Fat Arrow function
import React from 'react'
const App = () => {
return (
<div>App</div>
)
}
export default App
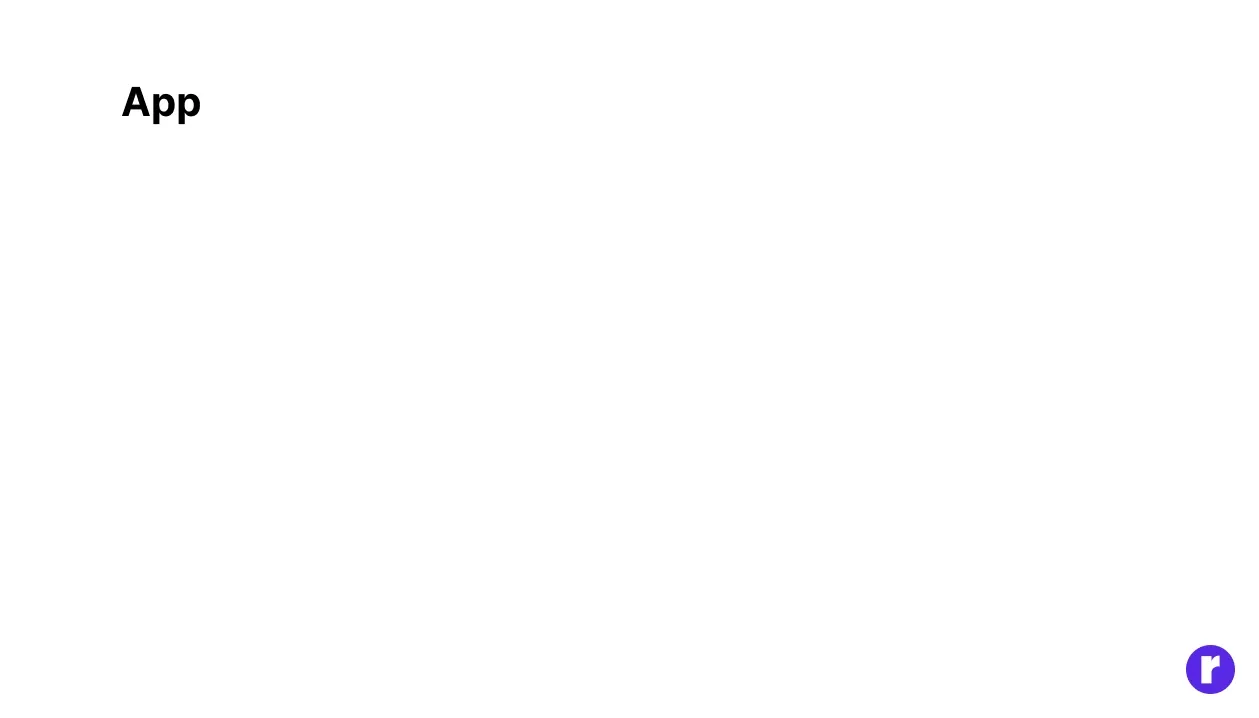
Simple Function
import React from 'react';
function FunctionalComponent() {
return (
<div>
<h1>Hello from the Functional Component!</h1>
<p>This is a functional component without any props.</p>
</div>
);
}
export default FunctionalComponent;
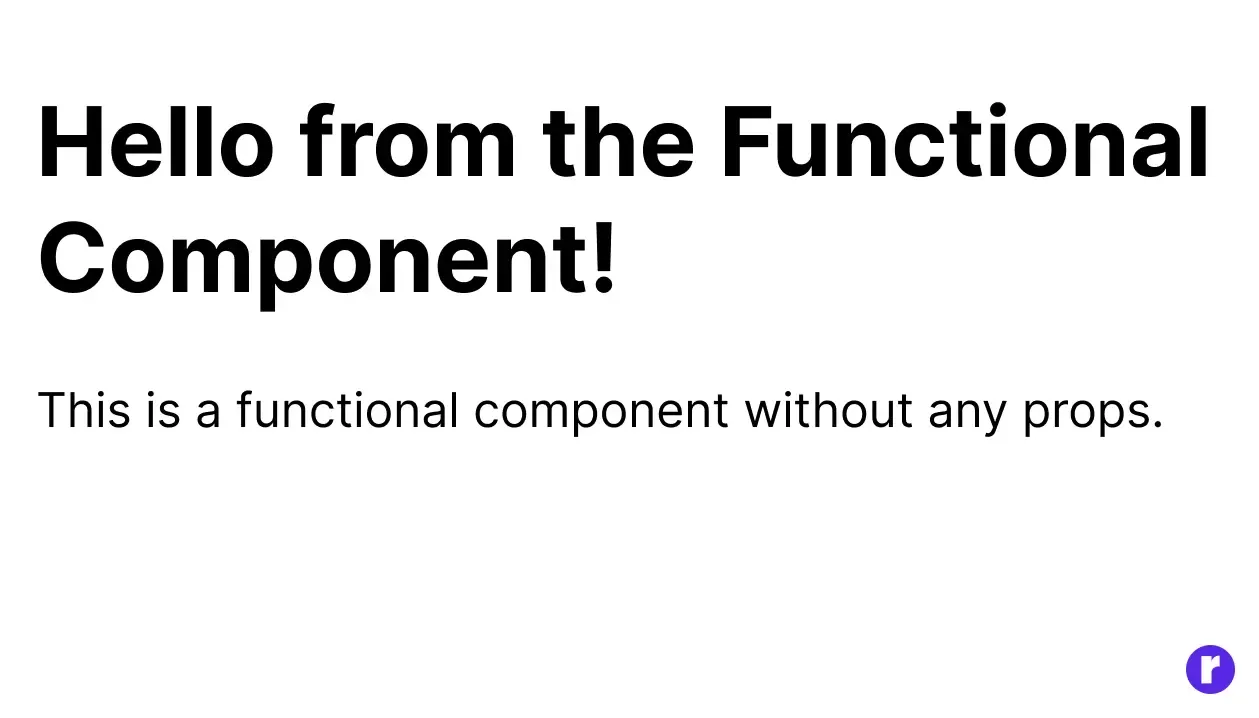
Refactoring Class Components
Class components are created with the class keyword. It does not directly return the react element. The render function returns the react element.
import React, { Component } from 'react';
class MyComponent extends Component {
render() {
return (
<div>
<h1>Hello, World!</h1>
<p>This component does not use props.</p>
</div>
);
}
}
export default MyComponent;
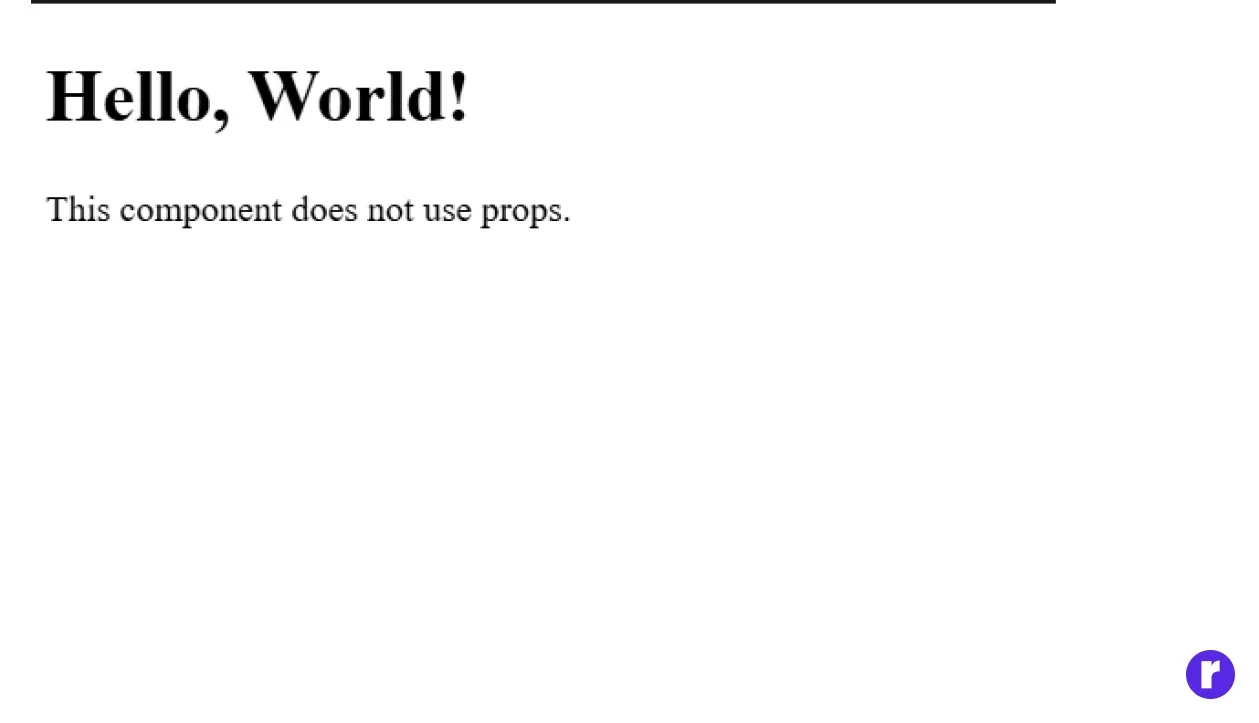
Exploring what is Props?
Props are used for passing the data from one component to another component. In other words, we can say that we can pass the data parent component to the child component with the help of props.
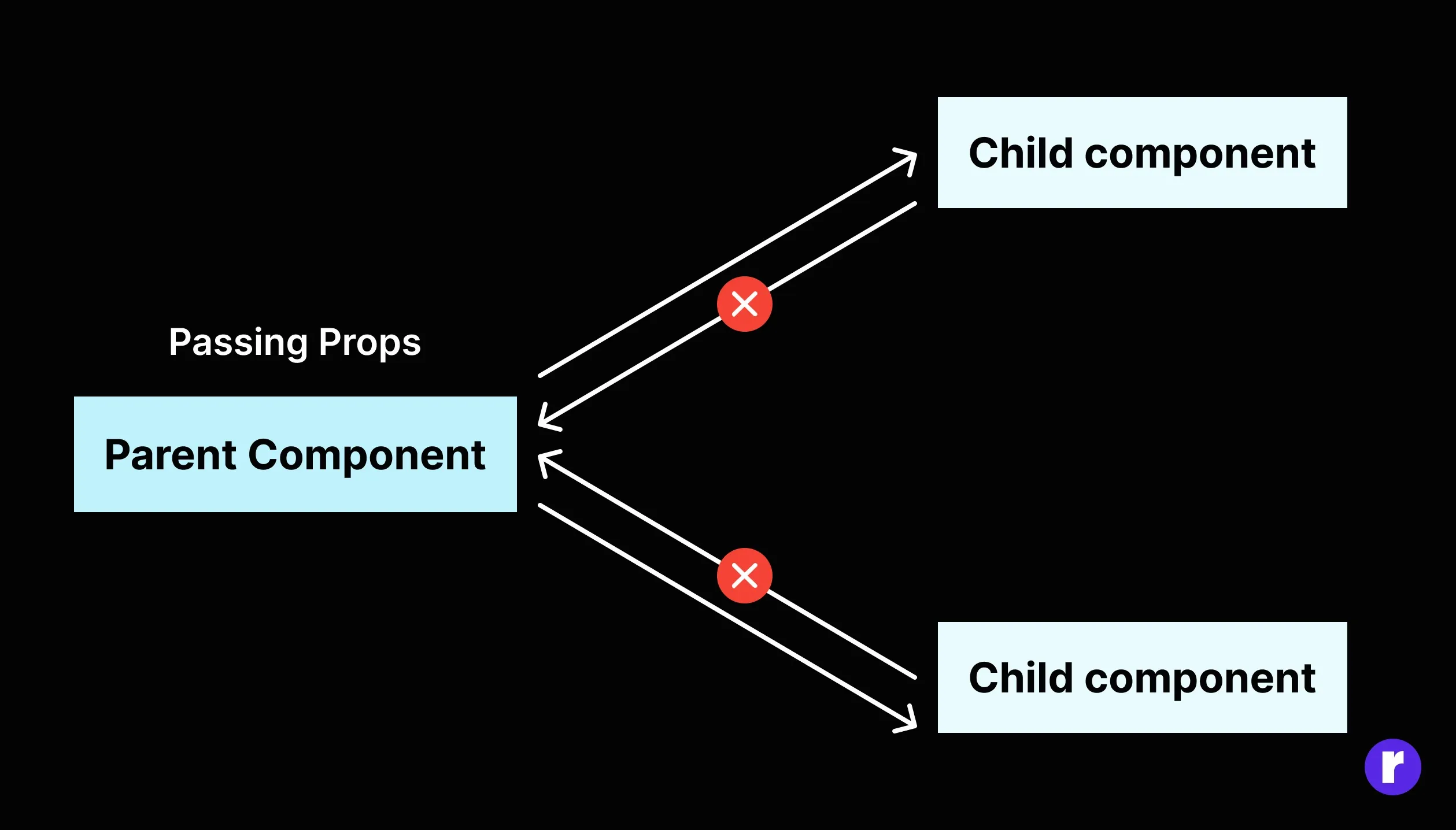
We can define the value and get the value of the props in two ways.
Using the props keyword
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const data = "Hello from Parent";
return (
<div>
<ChildComponent message={data} />
</div>
);
}
function ChildComponent(props) {
return (
<div>
<p>{props.message}</p>
</div>
);
}
export default ParentComponent;
Without using the props keyword
// ParentComponent.js
import React, { useState } from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const [data, setData] = useState("Hello from Parent");
return (
<div>
<ChildComponent data={data} />
</div>
);
}
export default ParentComponent;
//ChildComponent.js
import React from 'react';
function ChildComponent({ data }) {
return (
<div>
<p>{data}</p>
</div>
);
}
export default ChildComponent;
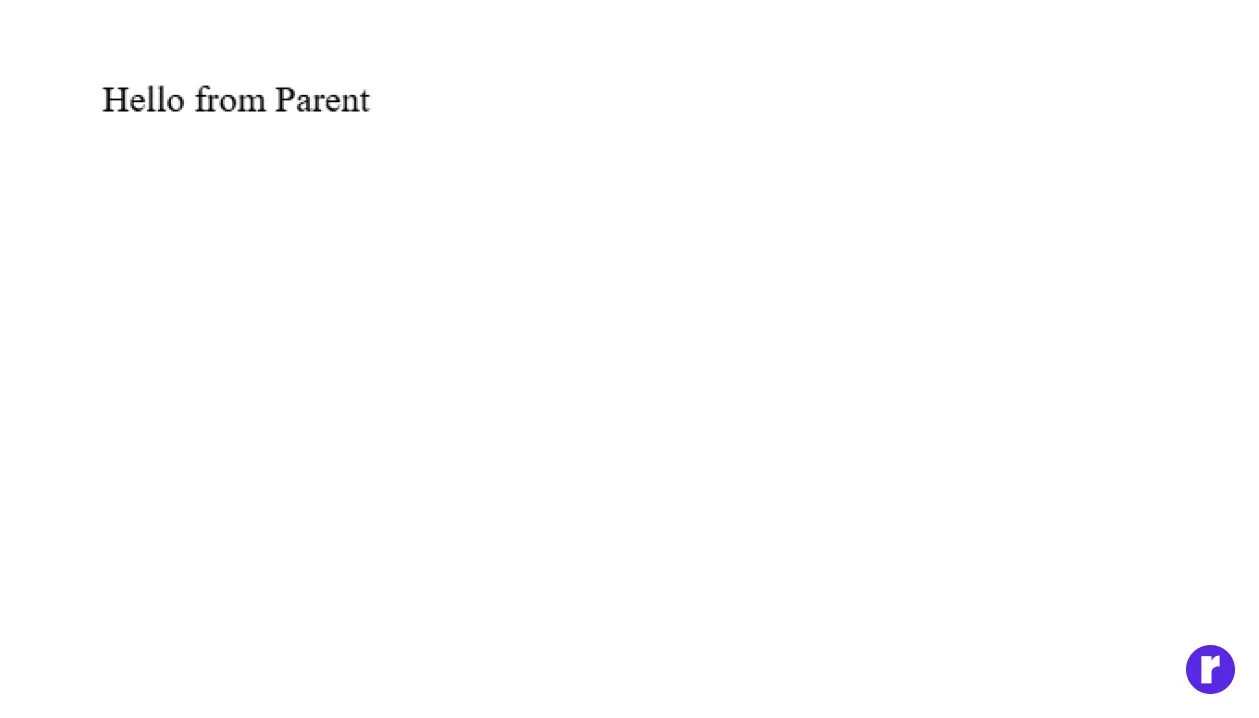
Here's an example of Props With props keyword on CodeSandbox for reference :- codesandbox example
The Power and Purpose of a Hook
Hooks in React are used to add state and lifecycle features to functional components. Before the introduction of hooks in React version 16.8, stateful logic and lifecycle methods were only available in class components. Hooks provide a way for functional components to have a local component state, perform side effects, and manage the lifecycle of a component.
Hooks in React provide a more flexible and powerful way to work with state and lifecycle in functional components, promoting code reuse, readability, and the adoption of modern React features. They have become an integral part of React development and are widely used in building modern React applications.
Here are some reasons why hooks are used in React:
- State Management in Functional Components: With the useStatehook, functional components can now declare and manage the local state. This eliminates the need for class components if the only reason for using them is to manage the state.
- Code Reusability: Hooks enable the extraction and reuse of stateful logic across multiple components. Custom hooks allow developers to create their reusable hooks, encapsulating complex logic and promoting code reusability.
- Readability and Maintainability: Functional components with hooks tend to be more concise and easier to read compared to class components with lifecycle methods. This can lead to more maintainable and understandable code.
- Encapsulation of Related Logic: Hooks allow developers to encapsulate related logic in separate custom hooks. This helps in organizing and separating concerns in a modular way, making the codebase more manageable.
- Better Support for Functional Programming: Hooks align well with the functional programming paradigm, making it easier to reason about and test components. They promote a more functional approach to building React components.
- Easier Adoption of React Features: Hooks make it easier for developers to adopt and understand React features such as context, reducers, and memoization in functional components, without the need for class components.
- Improved Performance: Hooks like useMemo and useCallback can help optimize performance by memoizing values and functions, preventing unnecessary re-renders.
Interested to learn more about React Hooks? Contact Us
Some of the commonly used React hooks include:-
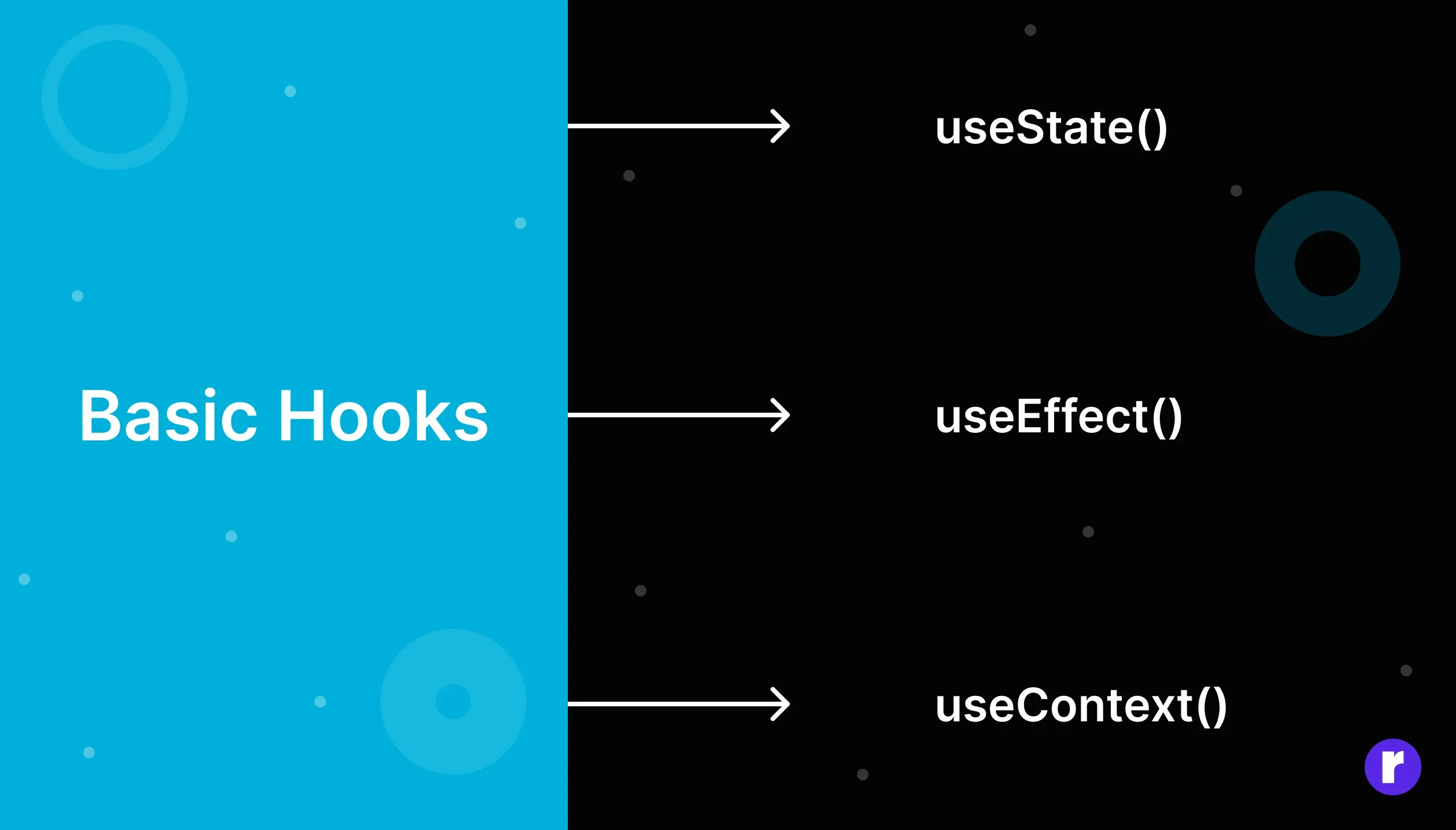
Exploring React's useState
In React, useState is a Hook that allows functional components to manage state. State in React is an object that represents the parts of your application that can change over time. It's a way to make components dynamic and interactive.
This mechanism helps manage the state of a component cleanly and predictably, making it easier to build dynamic and interactive user interfaces in React.
Here's a brief overview of why and how useState is used:
- Functional Components: In React, there are two types of components: class components and functional components. Before React 16.8, functional components were stateless, meaning they couldn't manage their state. With the introduction of Hooks, including useState, functional components can now have state.
- State Management: useStateis used to declare state variables in functional components. These variables can hold values that change during the component's lifecycle. When the state changes, React re-renders the component, updating the user interface to reflect the new state.
- Immutable Updates: The state in React should be treated as immutable. Instead of directly modifying the state variable, you use the function returned by useStateto update the state. This ensures that React can properly track changes and trigger re-renders as needed.
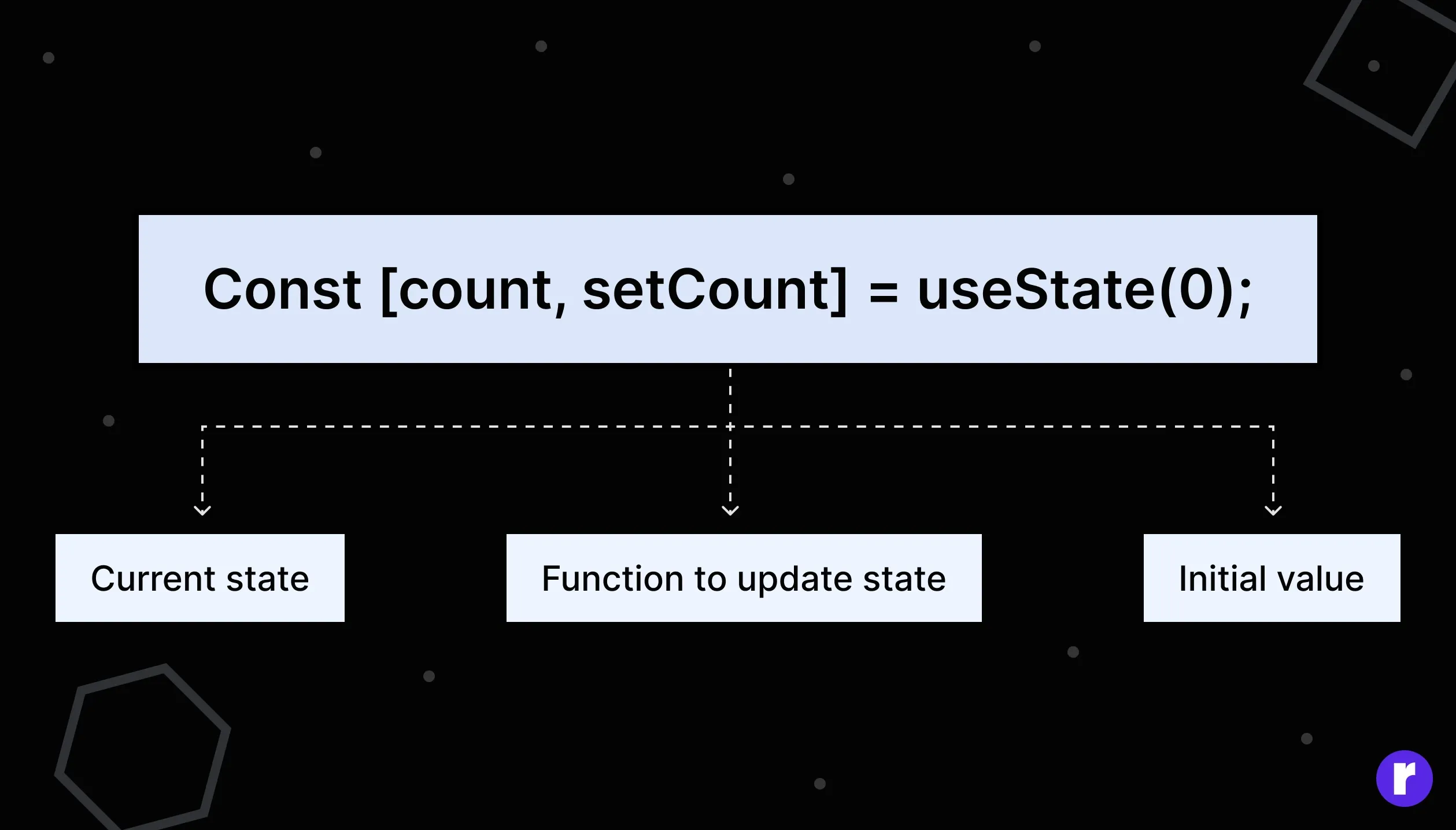
for example:
import React, { useState } from 'react';
function Counter() {
// Declare a state variable named "count" with an initial value of 0
const [count, setCount] = useState(0)
// Function to increment the count
const increment = () => {
setCount(count + 1);
};
// Function to decrement the count
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
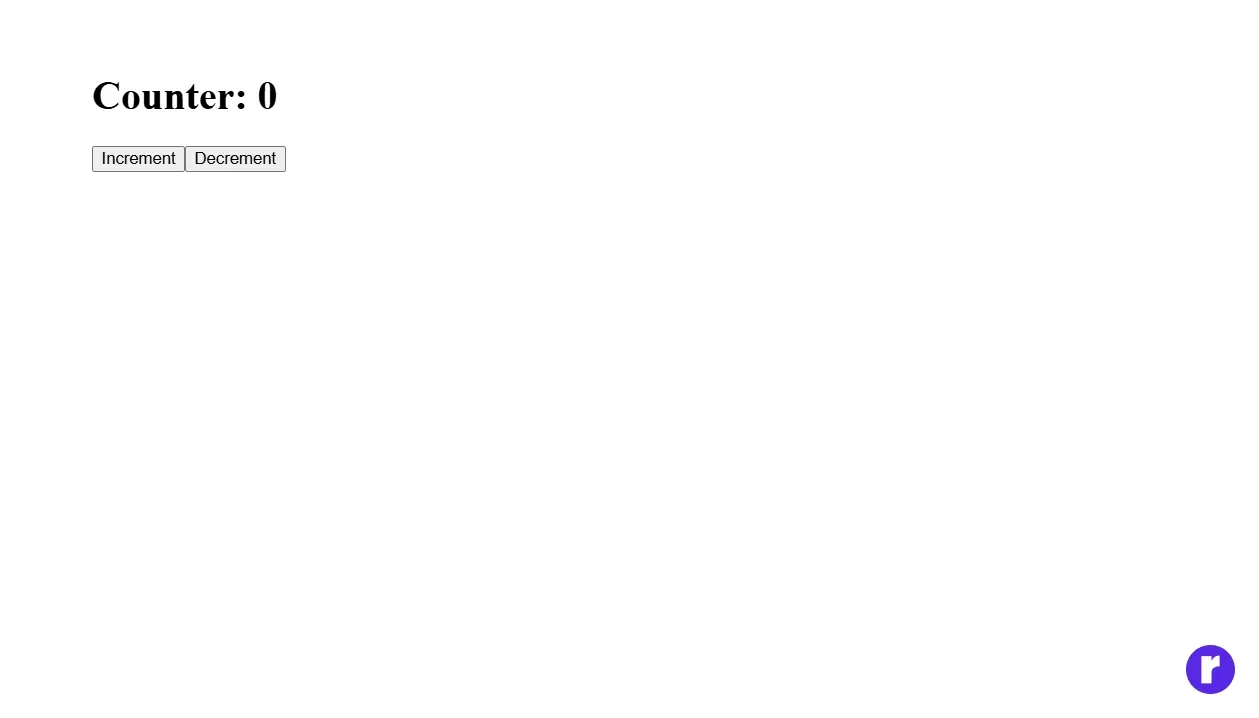
Optimizing React Component Lifecycle with useEffect
useEffectis a type of hook. It is utilized when we render a component without performing any specific event. Additionally, it is employed for updating the component's state and mounting the component.
In React, the useEffecthook facilitates executing side effects within functional components. Side effects in this context refer to any code that needs to be executed outside the scope of the current function, such as data fetching, subscriptions, manual DOM manipulations, or setting up and cleaning up timers.
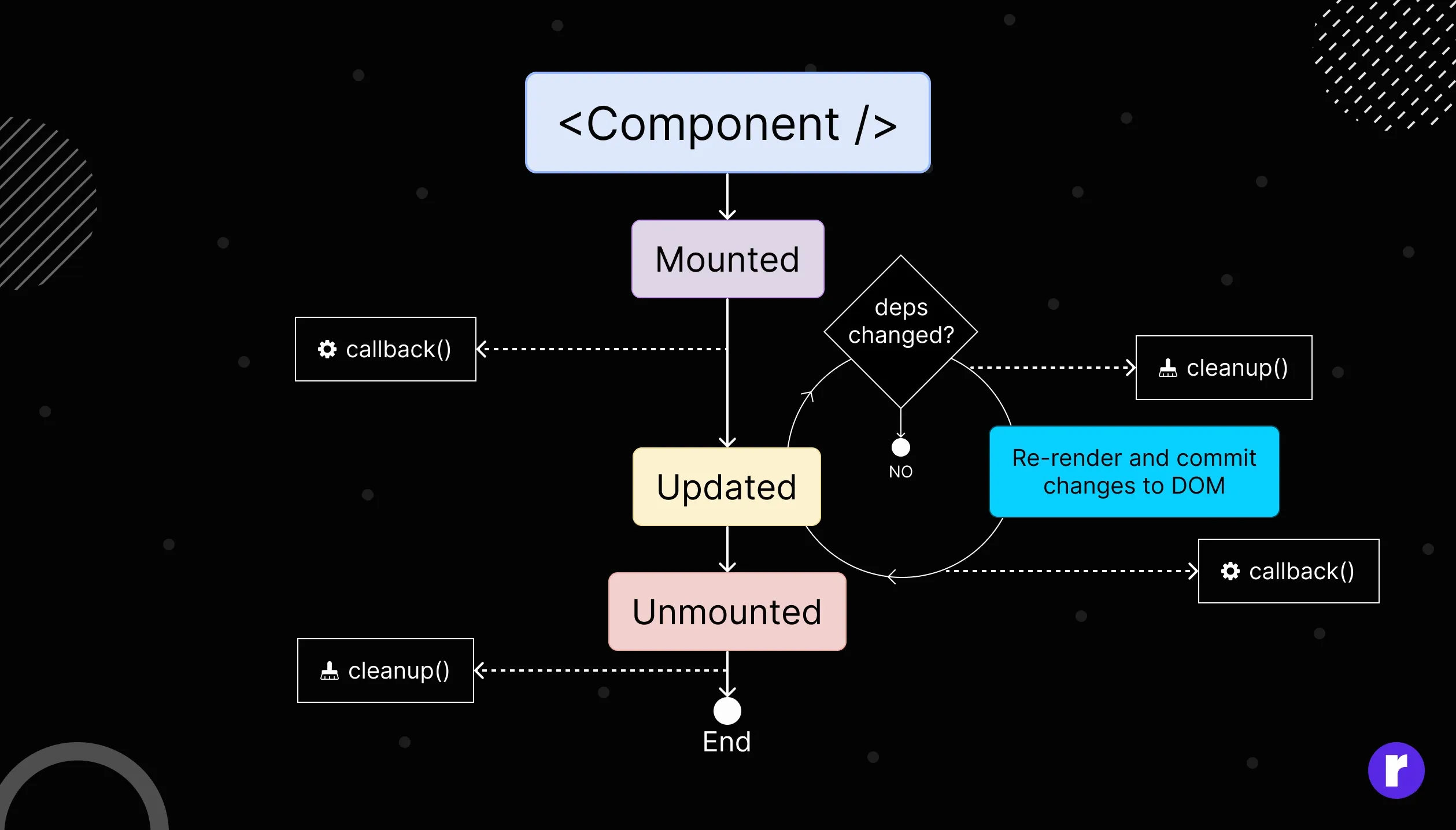
The basic syntax of useEffect looks like this:
// General structure of useEffect
useEffect(() => {
// Side effect code goes here
// Cleanup function (optional)
return () => {
// Code to run on component unmount or when dependencies change
};
}, [dependency1, dependency2, ...]);
- The first argument is a function containing the code for the side effect.
- The second argument is an optional array of dependencies. If any of the dependencies change between renders, the effect will be re-run. If no dependencies are provided, the effect will execute after each render.
Common use cases for useEffect include:
- Data fetching: Fetching data from an API or a server when the component mounts.
- Manual DOM manipulations: Manipulating the DOM directly.
- Cleanup operations: Performing cleanup when the component is unmounted.
useEffect(() => {
const fetchData = async () => {
// Fetch data
const result = await fetchDataFromAPI();
// Update state with fetched data
setData(result);
};
fetchData();
}, []); // Empty dependency array means this effect runs once after initial render
useEffect(() => {
// Update the title of the document
document.title = `You clicked ${count} times`;
}, [count]); // Run this effect only when the count changes
useEffect(() => {
// Initialize something
// Cleanup function
return () => {
// Clean up resources or subscriptions
};
}, []); // Empty dependency array for cleanup on unmount only
Empowering React Components with useContext
Context in React is a mechanism enabling data to be transmitted through the component tree without necessitating the manual passing of props at each level. It provides a way to share values, such as states or functions, across components without explicitly passing them as props.
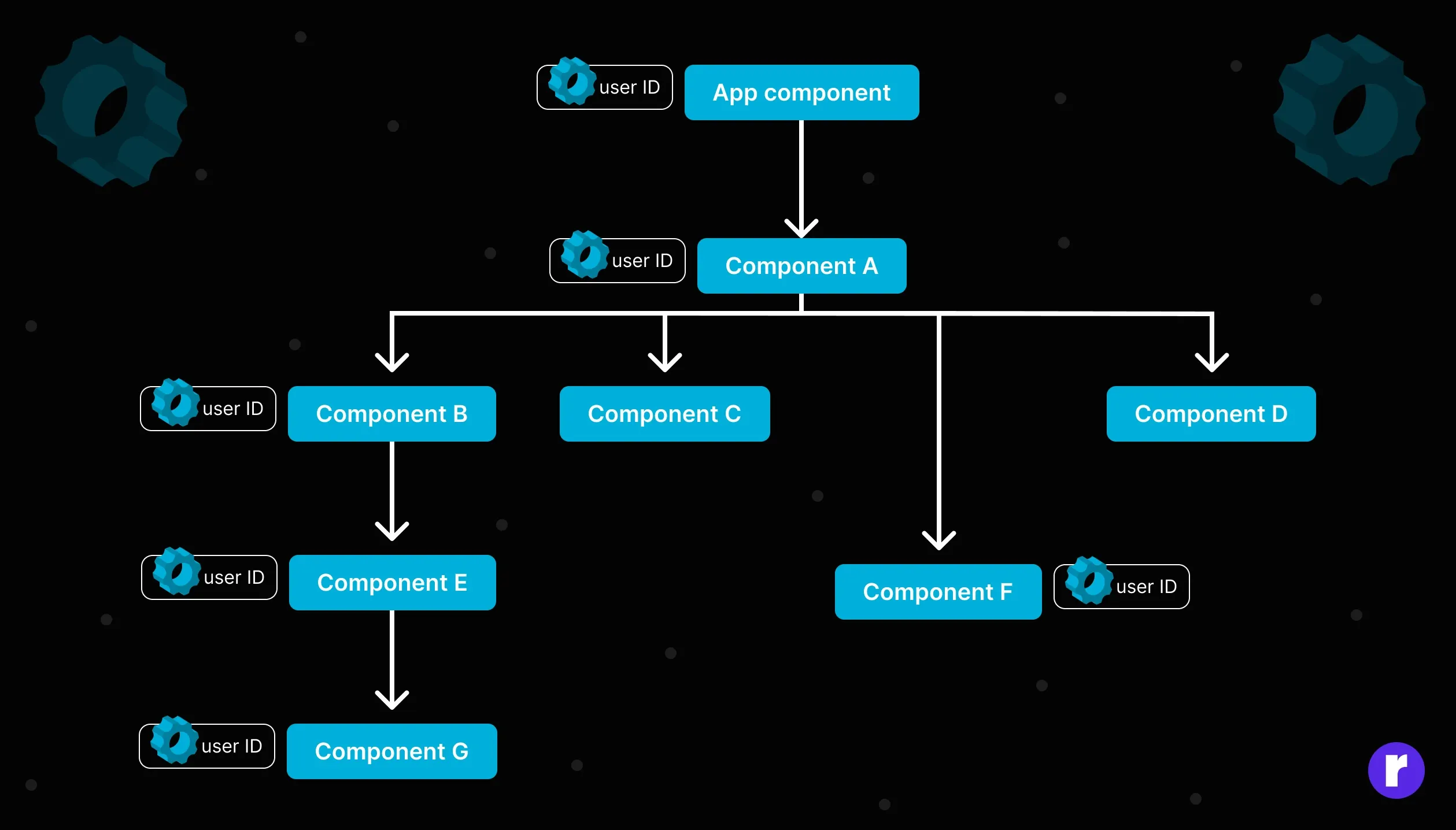
Why Use React Context?
- Avoid Prop Drilling: Context helps avoid prop drilling by providing a way to share values at any level of the component tree without passing them explicitly through each component.
- Global State Management: Context is commonly used for managing the global state in a React application. Instead of lifting the state up through parent components, you can use context to share the state across components.
- Theme Switching, Authentication, and Other Globals: Context is particularly useful for scenarios where you have global settings, such as theme information, authentication status, or any other application-wide data.
- Simplifies Component Composition: Context simplifies the process of composing components. Components can focus on their specific concerns, and shared data can be accessed when needed without cluttering the component API with unnecessary props.
import { createContext } from 'react';
const MyContext = createContext();
const ThemeContext = createContext('light');
function App() {
return (
<ThemeContext.Provider value="dark">
<MyComponent />
</ThemeContext.Provider>
);
}
function MyComponent() {
const theme = useContext(ThemeContext);
const data = useContext(MyContext);
return (
<div>
<div>Theme: {theme}</div>
<div>Data: {data}</div>
</div>
);
}
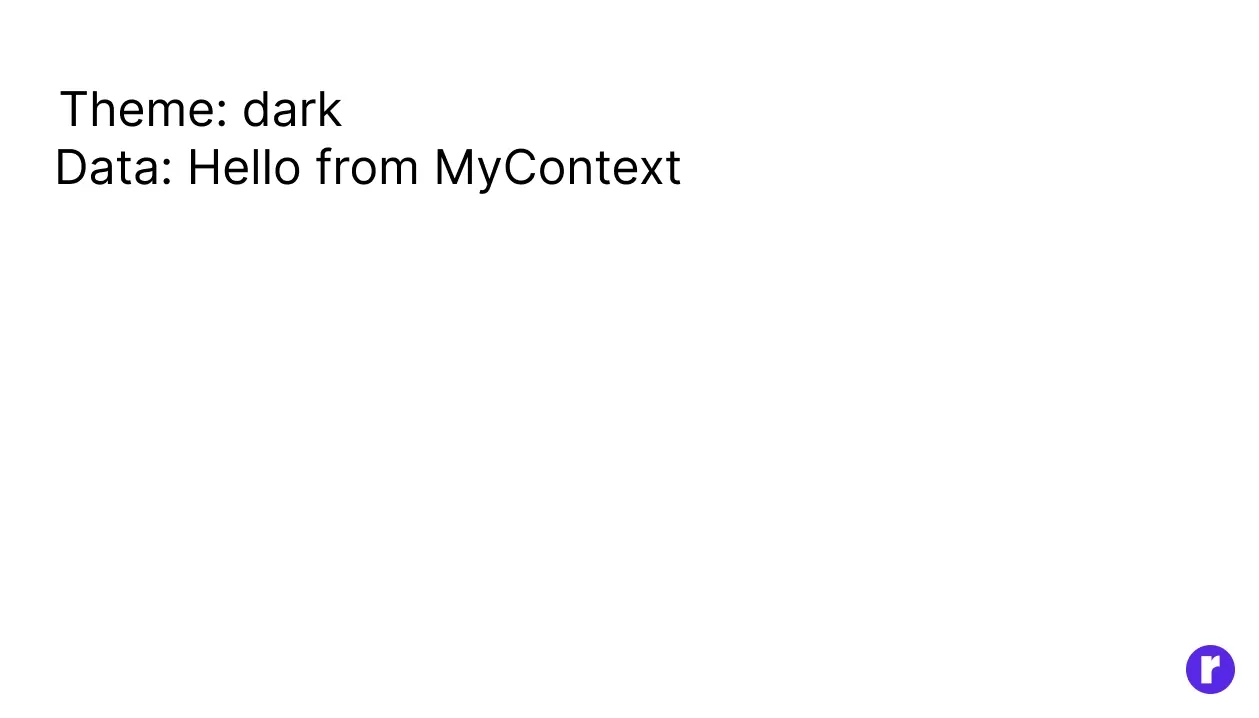
Conclusion
In conclusion, React hooks offer a more straightforward and versatile way of building and managing components in React applications. They simplify code, improve reusability, and enhance the overall developer experience, making them the preferred choice for many React developers.
React and hooks continue to be widely adopted in the web development community. They provide a robust foundation for building scalable and maintainable applications, and the ecosystem around React remains active and supportive. Developers find React's declarative approach, component-based architecture, and the introduction of hooks valuable for creating modern, efficient user interfaces.
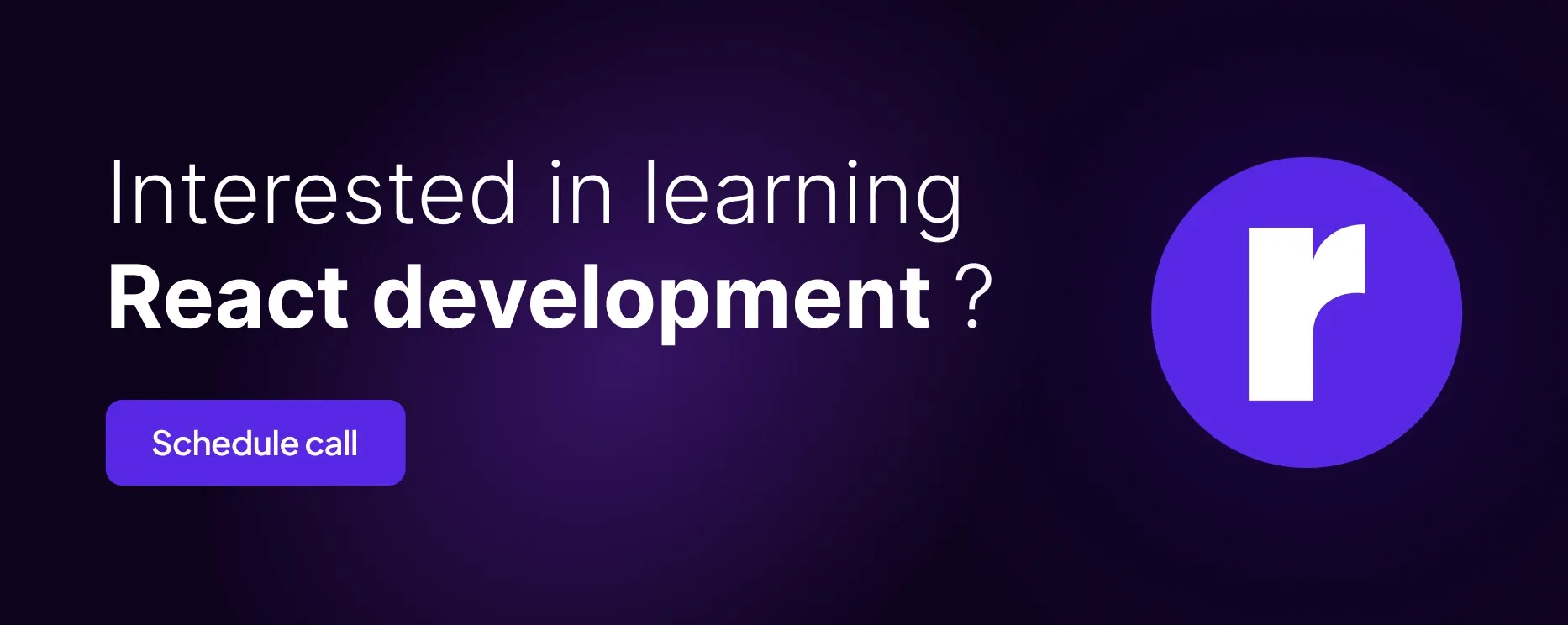