HTML Forms Modern Techniques for Enhanced User Interaction

Written by
Preeti Yadav
Front End Developer

Surender Singh
Front End Developer
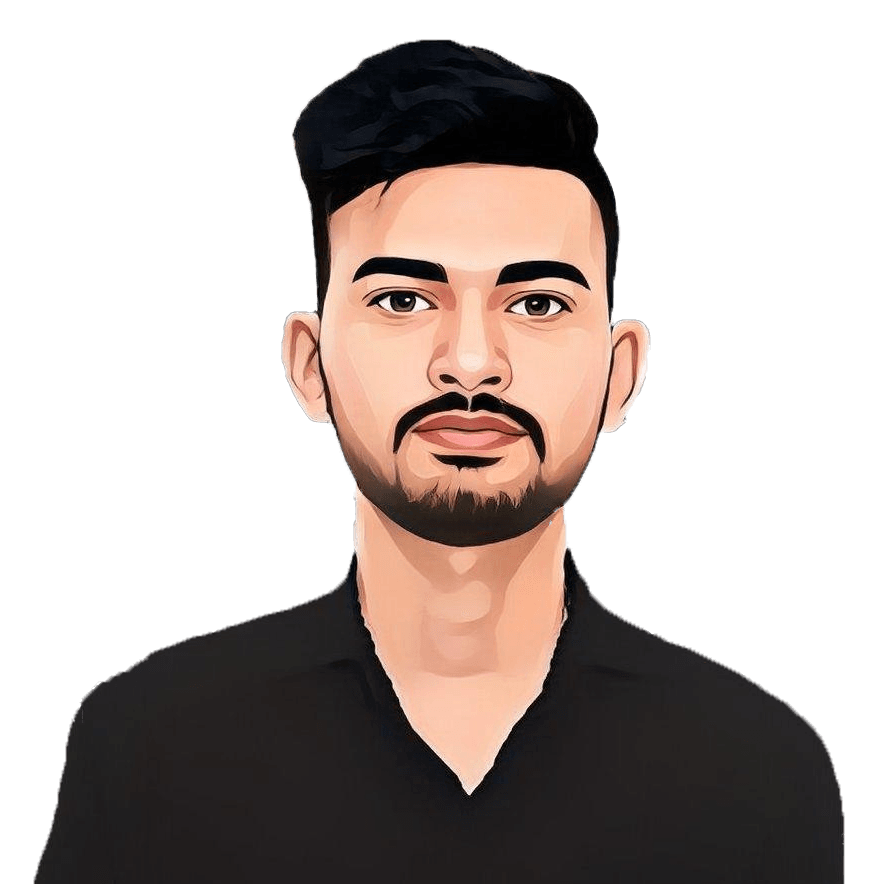
Table of contents
Build with Radial Code
In the dynamic landscape of web development, creating engaging and interactive user experiences is crucial. HTML forms play a vital role in collecting user input, facilitating communication, and driving user engagement on websites. As technology evolves, so do the techniques for designing and implementing HTML forms. In this blog post, we will explore modern techniques that enhance user interaction and improve the overall user experience when working with HTML forms.
HTML5 Input Types and Attributes
HTML 5, the fifth version of the Hypertext Markup Language, has ushered in a new era of web development by introducing a wide array of features that elevate the capabilities of web forms. This iteration of HTML not only focuses on providing a cleaner and more semantic structure for documents but also places a significant emphasis on enhancing the user experience through the introduction of various input types and attributes for forms.

- Email Input Types: The `email` input type is designed for capturing email addresses and includes built-in validation to ensure that users enter a properly formatted email. Here's an example:-
- URL Input Type: The URL input type is used for capturing website URLs and, like the email type, includes validation to ensure proper formatting. Here's an example:-
- Date Input Types: The date input type allows users to select a date from a native date picker. Here's an example:-
- Time Input Types: The time input type allows users to input a specific time using a native time picker. Here's an example:-
- Pattern Attribute: The `pattern` attribute allows developers to specify a regular expression pattern for the input value, enforcing a specific format. Here's an example of using the pattern attribute to create a custom `pattern` for a phone number:
- Color Input Type: The color input type empowers users to select a color effortlessly by utilizing a color picker dialog. This intuitive feature enhances the visual appeal of web forms and facilitates the input of specific color preferences. Let's explore a practical example:-
- Search Input Type: The search input type is tailor-made for search boxes, offering a specialized input field designed to enhance the search experience on websites. Although visually similar to a standard text input, the search input may exhibit distinct styling in various browsers, ensuring a consistent and user-friendly interface. Let's examine an illustrative example:-
- Range Input Type: The range input type in HTML introduces a versatile slider control, offering users an intuitive way to select a numeric value within a predefined range. This feature is particularly impactful in scenarios where fine-tuned adjustments are crucial. Here's a practical example showcasing the implementation of the range input:-
- type="range": Indicates that this input is a range slider.
- id="volume": A unique identifier linking the label with the input, enhancing user understanding.
- name="volume": The name attribute, which becomes valuable when processing form data on the server.
- min="0": Specifies the minimum value of the range (in this case, 0).
- max="100": Specifies the maximum value of the range (in this case, 100).
- step="1": Defines the incremental steps by which users can adjust the slider.
- value="50": Sets the initial position of the slider to 50.
<label for="email"> Email: </label>
<input type="email" id="email" name="email" required>
In this example, the type="email" attribute informs the browser that this input field should accept only valid email addresses. The required attribute ensures that the field must be filled out before submitting the form.
<label for="website">Website URL:</label>
<input type="url" id="website" name="website" required>
Similar to the email example, the type="URL" attribute enables URL-specific validation , and ensures the field is not left empty.
<label for="birthdate">Birthdate:</label>
<input type="date" id="birthdate" name="birthdate" required>
The type="date" attribute triggers a date picker in supporting browsers, making it easier for users to input dates. The required attribute ensures that a date is selected.
<label for="meeting-time">Meeting Time:</label>
<input type="time" id="meeting-time" name="meeting-time" required>
As with the date example, the type="time" attribute activates a time picker, facilitating the selection of specific times.
<label for="phone">Phone Number (Format: XXX-XXX-XXXX):</label>
<input type="text" id="phone" name="phone" pattern="\d{3}-\d{3}-\d{4}" title="Please enter a valid phone number (XXX-XXX-XXXX)" required>
In this example, the pattern attribute is set to \d{3}-\d{3}-\d{4}, where \d represents a digit. The title attribute provides a message that will be displayed if the entered value doesn't match the specified pattern. The required attribute ensures the field is not left empty.
<label for="fav-color">Favorite Color:</label>
<input type="color" id="fav-color" name="fav-color">
In this instance, the `color` input type provides users with a convenient color picker, allowing them to choose their favorite color with a simple click or drag. This functionality is particularly useful in applications where color customization plays a significant role, providing a seamless and engaging user experience.
<label for="email"> Email: </label>
<input type="email" id="email" name="email" required>
The placeholder attribute provides a hint to users about the expected input.
<label for="volume">Volume:</label>
<input type="range" id="volume" name="volume" min="0" max="100" step="1" value="50">
Label: This label serves to describe the purpose of the range input, contributing to clarity and accessibility.
Range Input Element: The range input is configured with several attributes:
Form Styling Elevating Aesthetics and Responsiveness
While the basic structure of HTML forms provides functionality, the visual appeal and responsiveness can be further enhanced through advanced CSS techniques. Let's explore how you can make your forms not only functional but also visually appealing using custom styling and CSS frameworks.

Custom Styling with CSS: To add a personal touch to your forms, consider custom styling using CSS. Use selectors to target specific form elements and apply styles such as colors, fonts, and spacing. Utilize pseudo-classes for hover effects or focus states to provide visual feedback to users as they interact with the form.
/* Example custom styling for input fields */
input{
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 5px;
}
/* Highlight input on focus */
input:focus{
border-color: #007bff;
box-shadow: 0 0 5px rgba(0, 123, 255, 0.5);
}
CSS Frameworks - Bootstrap: Bootstrap is a popular CSS framework that simplifies the process of styling HTML elements, including forms. It offers a grid system, pre-built components, and responsive tools. To integrate Bootstrap into your project, you can link the Bootstrap stylesheet in the
of your HTML document.<!-- Link to Bootstrap CSS -->
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
Now, you can leverage Bootstrap classes to style your forms easily:
<form class="container">
<div class="form-group">
<label for="exampleInputEmail">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail" placeholder="Enter email">
</div>
<div class="form-group">
<label for=" exampleInputPassword">Password</label>
<input type="password" class="form-control" id="exampleInputPassword" placeholder="Password">
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
CSS Frameworks - Tailwind CSS: Tailwind CSS is another popular utility-first CSS framework. It furnishes fundamental utility classes, empowering you to construct designs seamlessly within your markup. To use Tailwind CSS, include the CDN link in your HTML file.
<!-- Link to Tailwind CSS -->
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@^2.2.19/dist/tailwind.min.css" rel="stylesheet">
Now, you can apply Tailwind CSS classes directly in your HTML:
<!-- Example Tailwind CSS-styled form -->
<form class="container mx-auto my-8 p-8 bg-gray-200 rounded-md shadow-md">
<label class="block mb-2 text-1g font-bold text-gray-800" for="email">Email address</label>
<input class="w-full p-2 mb-4 border rounded-md" type="email" id="email" placeholder="Enter your email">
<input class="block mb-2 text-1g font-bold text-gray-800" for="password">Password</label>
<label class="w-full p-2 mb-4 border rounded-md" type="password" id="password" placeholder="Enter your password">
<button class="w-full p-2 bg-blue-500 text-white font-bold rounded-md" type="submit">Submit</button>
</form>
Upgrade Your HTML Forms: Discover Modern Techniques for Enhanced User Interaction. Click here to Learn More!
Security Measures
Briefly touch upon security measures like HTTPS, secure coding practices, and CSRF protection when handling form submissions. Encourage the use of secure connections and proper data handling.

- HTTPS (SSL/TLS Encryption): Ensure that your website uses HTTPS to encrypt data transmitted between the user's browser and your server. This helps in protecting sensitive information like login credentials, personal details, and payment information from being intercepted by attackers.
- Secure Coding Practices: Emphasize the importance of secure coding practices among developers. This includes validating user inputs, avoiding code injection vulnerabilities, and regularly updating and patching software to fix any security vulnerabilities.
- CSRF (Cross-Site Request Forgery) Protection: Implement measures to protect against CSRF attacks. This involves generating and validating unique tokens for each user session, ensuring that malicious actors cannot perform unauthorized actions on behalf of users.
- Secure Connection: Encourage users to connect to your website or application using secure and private networks. Avoid public Wi-Fi for sensitive activities, as these networks may expose users to potential security threats.
- Proper Data Handling: Highlight the importance of handling user data responsibly. This includes storing sensitive information securely, implementing proper access controls, and regularly auditing and monitoring data access to detect any unauthorized activities.
Accessibility Considerations Ensuring Inclusive Web Forms
As we delve deeper into the realm of modern HTML forms, it is imperative to shine a spotlight on accessibility considerations. Creating web forms that are inclusive and cater to users of all abilities is not just a best practice; it's a fundamental aspect of responsible web development. Let's explore key considerations for making HTML forms accessible to everyone.

- Semantic HTML for Improved Screen Reader Experience: Utilizing semantic HTML elements not only aids in creating well-structured documents but also enhances the experience for screen reader users. Employing <fieldset> and <legend> elements provides a clear and organized structure, making it easier for users to navigate through the form.
- Descriptive Form Labels: Clearly and concisely label each form element to provide context and guidance. Screen readers often rely on these labels to convey information to users. Using the
- Error Handling and Messaging: When errors occur during form submission, present clear and descriptive error messages. These messages should not rely solely on color but should also include text that describes the issue. Users with visual impairments or color blindness will benefit from well-crafted error messages.
- Keyboard Navigation: Ensure that all form elements are accessible and usable through keyboard navigation alone. Users who rely on keyboards or other assistive devices should be able to navigate through the form, select fields, and submit without encountering any obstacles. Testing your forms using only a keyboard can reveal potential issues that need addressing.
<fieldset>
<legend>Contact Information</legend>
<!-- Form fields go here -->
</fieldset>
<label for="email"> Email Address</label>
<input type="email" id="email" name="email">
Conclusion
In conclusion, modernizing HTML forms involves leveraging the rich set of features offered by HTML5, embracing advanced CSS techniques for enhanced aesthetics, and prioritizing security and accessibility. Utilizing HTML5 input types and attributes provides users with intuitive options, improving both user experience and data accuracy. Form styling, whether through custom CSS or popular frameworks like Bootstrap and Tailwind CSS, adds a layer of visual appeal and responsiveness to the functionality. Implementing robust security measures such as HTTPS, secure coding practices, and CSRF protection safeguards user data and maintains the integrity of form submissions. Lastly, ensuring accessibility considerations through semantic HTML, descriptive labels, thoughtful error handling, and keyboard navigation ensures that web forms are inclusive and user-friendly for individuals of all abilities. By combining these techniques, web developers can create HTML forms that not only collect information efficiently but also contribute to a positive and engaging user experience in the ever-evolving landscape of web development.
