LocalStorage vs. Session Storage vs. Cookies: Which Solution Reigns Supreme?
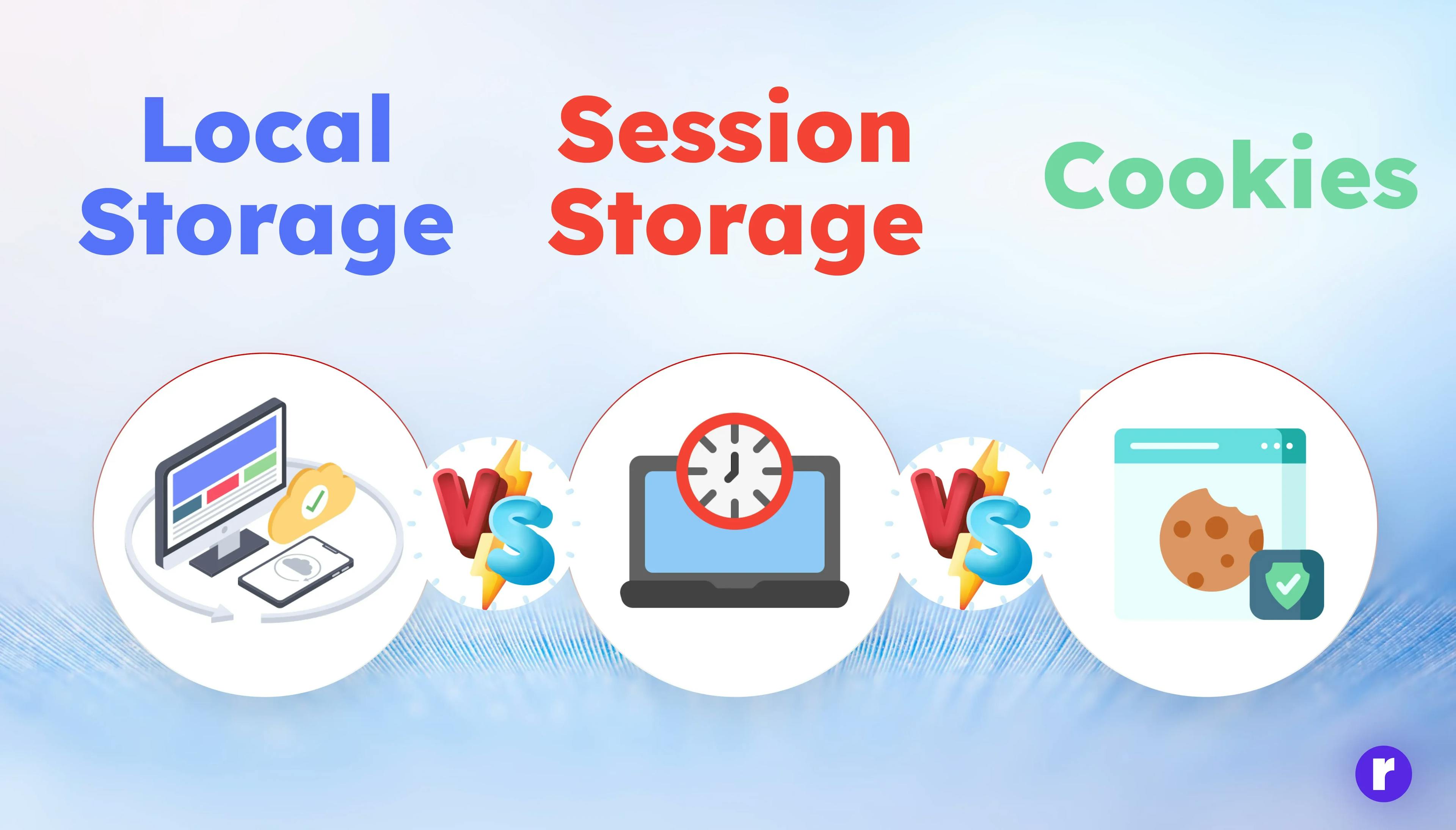
Written by
Preeti Yadav
Front End Developer

Kapil Dev
Front End Developer
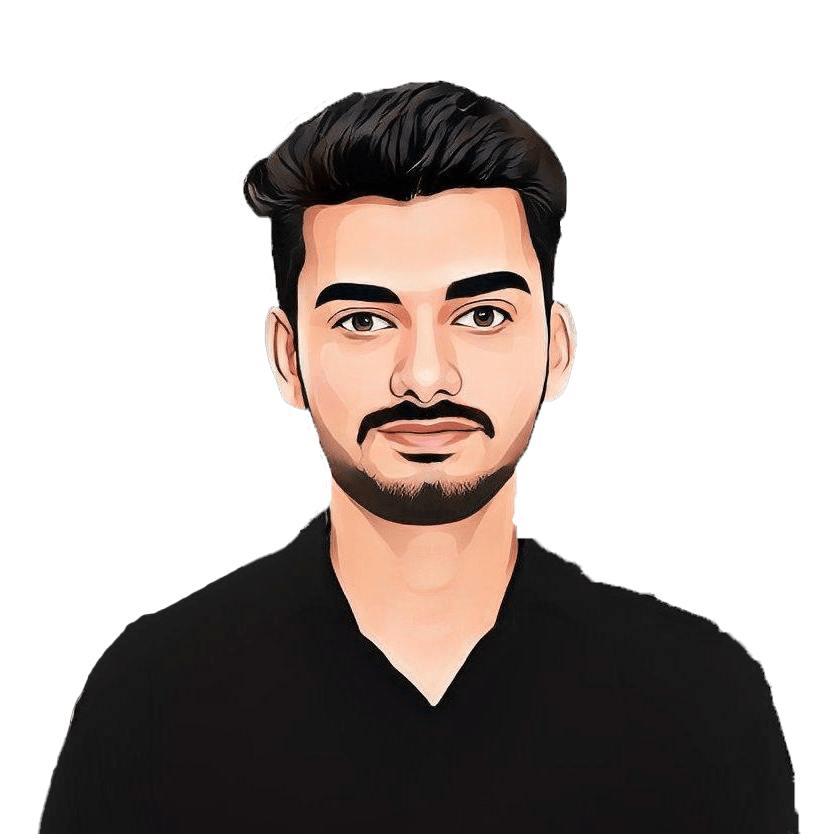
Table of contents
Build with Radial Code
When it comes to storing data right in your web browser, you've got three main options: LocalStorage, Session Storage, and Cookies. Each of these methods serves a unique purpose in managing data within the browser environment. Let's explore the differences and use cases for each of these storage solutions.
What's LocalStorage?
Think of LocalStorage as a backpack that stays with you all day, every day. It's a place in your web browser where you can store data, and it won't disappear even if you close your browser or turn off your computer. You can come back days or weeks later, open your browser, and voila! Your data is still there.

Here's an example of how you can use local storage in JavaScript to save a user's name so that even if they close the browser and come back later, their name will still be remembered:
// This program asks the user for their name, saves it, and greets them.
// Function to ask for the user's name and save it in local storage
function saveUserName() {
var userName = prompt("What is your name?");
if (userName) {
// Save the name in local storage
localStorage.setItem('userName', userName);
alert("Your name has been saved.");
} else {
alert("You didn't enter a name.");
}
}
// Function to greet the user using the name from local storage
function greetUser() {
// Get the name from local storage
var storedName = localStorage.getItem('userName');
if (storedName) {
// If a name was found, greet the user with it
alert("Hello, " + storedName + "! Welcome back.");
} else {
// If no name was found, ask for it
saveUserName();
}
}
// Check if we already have a user's name when the page loads
greetUser();
In this code, we have two functions: saveUserName() and greetUser(). The saveUserName() function asks the user for their name with prompt() and then uses localStorage.setItem() to store it. The key we use to save the item is 'userName'.
The greetUser() function tries to get the user's name from local storage using localStorage.getItem(). If it finds a name, it greets the user. If not, it calls saveUserName() to ask for a name.
When the page loads, greetUser() is called to either greet the user or ask for their name if it's not saved yet. This way, the user's name is remembered by the browser.
Pros of LocalStorage:
- Stays Forever: Your data sticks around until you decide to clear it out.
- Space to Spare: You get a lot of space to store things (usually about 5MB).
- Easy Access: It's super simple to use with JavaScript.
Cons of LocalStorage:
- Security Watch: Since it never goes away on its own, sensitive info might stick around too long.
- No Expiry Date: You have to manually remove data when you don't need it anymore.
What's Session Storage?
Now, imagine Session Storage as a lunchbox. You fill it up in the morning, use it throughout the day, but once the day is over, it gets cleaned out. Session Storage is similar; it keeps your data for one session. If you close the tab or the browser, poof! The data is gone.

Here's an example of how you can use session storage in your web pages with JavaScript:
// Saving data to sessionStorage
sessionStorage.setItem('key', 'value');
// Getting saved data from sessionStorage
var data = sessionStorage.getItem('key');
// Removing saved data from sessionStorage
sessionStorage.removeItem('key');
// Removing all saved data from sessionStorage
sessionStorage.clear();
Let me explain what each line does:
- sessionStorage.setItem('key', 'value'); - This line saves a piece of data to the session storage. The first argument, 'key', is like a label for our data, and 'value' is the actual information we want to store.
- var data = sessionStorage.getItem('key'); - With this line, we retrieve the data we stored earlier using its key. Now, the variable data will hold the value 'value'
- sessionStorage.removeItem('key'); - If we want to delete a specific piece of data from the session storage, we use this line. It removes the data associated with the key 'key'.
- sessionStorage.clear(); - When we want to clear everything out of the session storage, we use this command. It's like hitting the reset button; everything saved in the session storage will be deleted.
Keep in mind, session storage is like local storage. However, the data in session storage gets wiped once you close the page. A page session goes on as long as your browser is open and it can handle page reloads and coming back to pages. If you open the page in a new tab or window, it will have a new session storage.
Pros of Session Storage:
- Short-Term Memory: It's perfect for temporary data like form inputs.
- Tab-Specific: Each browser tab has its own separate storage.
- Auto-Clean: Once you close the tab, it cleans up after itself.
Cons of Session Storage:
- Limited Lifespan: Not great if you need to keep data for a long time.
- Less Space: You still get a good amount of space, but it's not meant for huge amounts of data.
What are Cookies?
Cookies are small data bits saved in your browser. They remember things like your login details, what you like on a site, and what's in your shopping cart. Cookies have been key to surfing the web for years and serve many uses.

Here's an example of setting a cookie in JavaScript:
// Setting a cookie with an expiration date
document.cookie = "username=John Doe; expires=Thu, 18 Dec 2023 12:00:00 UTC";
// To add more cookies, you can use the same document.cookie syntax with different key-value pairs separated by semicolons.
// For example:
// document.cookie = "username=John Doe; expires=Thu, 18 Dec 2023 12:00:00 UTC";
// document.cookie = "theme=dark; path=/";
// document.cookie = "language=en; max-age=3600";
In this example, a cookie named "username" with the value "John Doe" is set to expire on December 18, 2023, at 12:00:00 UTC.
Pros of Cookies:
- Versatile: Cookies can store a wide range of data types.
- Cross-Domain Usage: Cookies can be accessed across different domains.
- Automatic Handling: Browsers automatically send cookies with each request to the same domain.
Cons of Cookies:
- Limited Size: Cookies have size limitations compared to LocalStorage and Session Storage.
- Security Concerns: As cookies are stored in plain text, they can be vulnerable to attacks like cross-site scripting.
- Privacy Issues: Some users may be concerned about privacy implications of persistent cookies tracking their online activities.
So, Who Wins the Storage Battle?

It's not really about who wins, but more about which one fits your mission. Need to save data for a long time, like user preferences or game scores? LocalStorage is your hero. But if you're looking for a place to keep data that's only needed while someone is using your site, like items in a shopping cart during a single visit, then Session Storage is your go-to sidekick.
Remember, both LocalStorage, Session Storage, and Cookies have their own superpowers. They're part of what we call the Web Storage API, and they make our online experience smoother and more personalized. Just choose the one that matches what you need, and you'll be all set!
Master Your Data Storage Choices: Explore LocalStorage, Session Storage, and Cookies with Our Expert Guidance. Click here to Decide the Best Solution for Your Needs!
Feature |
LocalStorage |
Session Storage |
Cookies |
---|---|---|---|
Duration |
Stays until manually cleared |
Clears when the session ends |
Expires based on set duration |
Capacity |
About 5MB to 10MB |
About 5MB to 10MB |
Up to 4KB |
Accessibility |
Any window |
Same tab or window |
Any window with correct domain |
Server Access |
No, only client-side |
No, only client-side |
Yes, sent with HTTP requests |
Use Case |
Long-term data storage |
Data for a single session |
Authentication, tracking |
Browser Support |
HTML5 browsers |
HTML5 browsers |
All browsers |
Security |
Vulnerable to XSS attacks |
Vulnerable to XSS attacks |
Vulnerable if not secure/httponly |
Example Code |
localStorage.setItem('user', 'Alice'); |
sessionStorage.setItem('sessionUser', 'Bob'); |
document.cookie = "userId=Charlie; expires=Fri, 31 Dec 9999 23:59:59 GMT"; |
Conclusion
In conclusion, when deciding between LocalStorage, Session Storage, and Cookies for your web projects, it's essential to understand the specific use cases and limitations of each storage method. LocalStorage is ideal for persistent data storage, Session Storage is suitable for temporary data needs, and Cookies are commonly used for user authentication and personalization. By leveraging the strengths of each storage solution, developers can create more efficient and user-friendly web applications tailored to their requirements.
