Mastering CSS Grid Layout for Modern Web Design

Written by
Surender Singh
Front End Developer
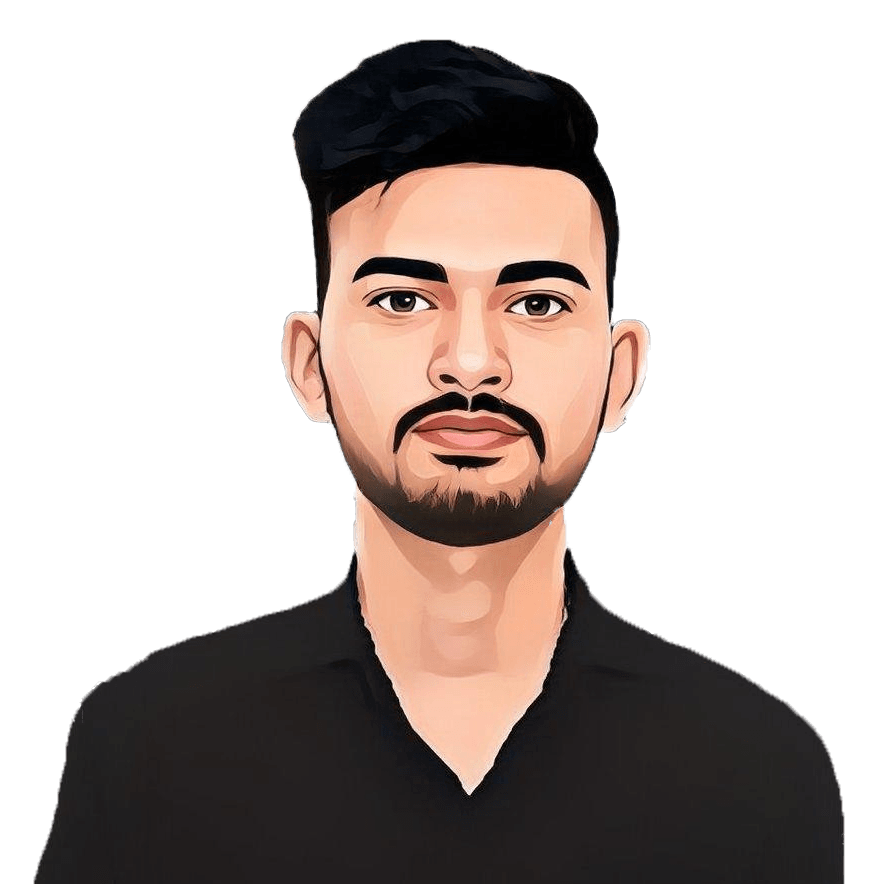
Preeti Yadav
Front End Developer

Table of contents
Build with Radial Code
In the ever-evolving landscape of web design, CSS Grid has emerged as a powerful tool for creating responsive and dynamic layouts. In this blog post, we will delve into the details of CSS Grid and explore how it can be mastered to enhance your web design skills.
What is CSS Grid?

CSS Grid is a two-dimensional layout system that allows you to design complex web layouts with ease. It provides a grid-based layout system, making it simpler to create both rows and columns for your web pages.
Key Concepts:
- Grid Container: This is the main part that holds all your grid items. To make something a grid container, you just set display: grid; Here's how:
- Grid Items: These are what you put inside the grid container. They can take up one or more rows and columns, so you can get creative with how things look. Like this:
- Grid Lines: Grid lines are the invisible lines that divide a CSS grid into rows and columns, helping to organize and place grid items. These lines are numbered starting from 1 at both the start and end of the grid. You can use these line numbers to position grid items precisely within the grid layout by specifying the starting and ending grid lines.
- Grid Gaps: Grid gaps, also known as grid spacings, are the spaces between rows and columns in a CSS grid layout. These gaps can be adjusted to control the amount of space between the grid lines, helping to improve the layout and visual organization of your content.
- Pixels (px): A fixed measurement unit. For example, 10px will always be 10 pixels wide.
- Percent (%) A relative measurement unit based on the size of the grid container. For example, 10% will be 10% of the grid container's size.
- Fraction (fr): A flexible unit that represents a fraction of the available space. For example, 1fr will take up one part of the available space, and 2fr will take up two parts of the available space.
- Grid Template: This defines your grid's layout by setting the number of rows and columns and their sizes. Use grid-template-rows and grid-template-columns to create your layout. See here:
- Grid Area: Grid area in CSS is used to define a specific section within a grid layout, allowing you to place grid items in designated areas of the grid. The grid-area property is used to assign a name to a grid item, which can then be referenced in the grid-template-areas property to create a layout template. This helps in organizing content in a visually structured way.
- Justify and Align: CSS Grid has tools like justify-items, align-items, justify-content and align-content. They help you line up items in cells or across the whole grid, both horizontally and vertically. For example:
- Responsive Design with Media Queries: Use media queries with CSS Grid to make sure your designs fit on all screen sizes. Your design will look great no matter the device. Like this:
.container {
display: grid;
}
This code makes the .container class into a grid.
<div class="container">
<div>Item 1</div>
<div>Item 2</div>
</div>
The <div> elements turn into grid items because they're in .container.
.container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* Three equal columns */
grid-template-rows: repeat(2, 100px); /* Two rows, each 100px tall */
}
.item1 {
grid-column: 1 / 3; /* Starts at column line 1 and ends at column line 3 */
grid-row: 1 / 2; /* Starts at row line 1 and ends at row line 2 */
}
.item2 {
grid-column: 2 / 4; /* Starts at column line 2 and ends at column line 4 */
grid-row: 2 / 3; /* Starts at row line 2 and ends at row line 3 */
}
You can specify the sizes of these gaps using different units:
Here is how you can use these units to set grid gaps in CSS:
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr; /* Three equal columns */
grid-template-rows: auto; /* Rows adjust automatically */
gap: 10px; /* 10 pixels gap between rows and columns */
}
.container-percent {
display: grid;
grid-template-columns: 1fr 1fr 1fr; /* Three equal columns */
grid-template-rows: auto; /* Rows adjust automatically */
gap: 5%; /* 5% gap between rows and columns */
}
.container-fr {
display: grid;
grid-template-columns: 1fr 2fr; /* Two columns, one twice as wide as the other */
grid-template-rows: auto; /* Rows adjust automatically */
gap: 1fr; /* Flexible gap based on available space */
}
.container {
grid-template-columns: repeat(3, 1fr);
grid-template-rows: auto;
}
This creates three columns that are all the same width.
.container {
display: grid;
grid-template-areas:
"header header"
"sidebar content"
"footer footer";
grid-template-columns: 1fr 3fr;
grid-template-rows: auto;
}
.header {
grid-area: header;
}
.sidebar {
grid-area: sidebar;
}
.content {
grid-area: content;
}
.footer {
grid-area: footer;
}
This names an area 'header' and assigns it to an item.
.container {
align-items: center;
}
This puts items in the middle of their cells going up and down.
@media (max-width: 600px) {
.container {
grid-template-columns: 1fr;
}
}
This switches the grid to one column on smaller screens.
Interested in coding? We're here to help!
HTML Structure
Before exploring the details of spacing and alignment, it's important to start with a simple CSS grid. The given HTML code shows a div labeled "snacks" that serves as the main grid container, which contains several other div within it.

After incorporating the HTML content, a touch of CSS is applied to enhance the container's visual prominence against the plain background. You have the freedom to adjust the styling as needed if you're following along.

Advanced Grid Techniques with Bootstrap
For those already familiar with Bootstrap, incorporating CSS Grid can enhance your layout capabilities. Let's explore an example where Bootstrap classes are combined with CSS Grid.

<div class="container mt-5">
<div class="grid-container">
<div class="grid-item bg-primary"></div>
<div class="grid-item bg-success"></div>
<div class="grid-item bg-info"></div>
<div class="grid-item bg-warning"></div>
<div class="grid-item bg-danger"></div>
<div class="grid-item bg-secondary"></div>
<div class="grid-item bg-dark"></div>
<div class="grid-item bg-primary"></div>
<div class="grid-item bg-info"></div>
<div class="grid-item bg-primary"></div>
<div class="grid-item bg-success"></div>
<div class="grid-item bg-warning"></div>
</div>
</div>
.grid-container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
grid-auto-rows: 150px;
gap: 10px;
}
.grid-item {
border-radius: 10px;
}
@media (max-width: 768px) {
.grid-container {
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
}
}
@media (max-width: 576px) {
.grid-container {
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
}
}
Enhancing Grids with Tailwind CSS
- Basic Grid Setup: Tailwind simplifies the creation of a basic grid. Apply the gridclass to your container and define the number of columns using the grid-cols-N class. Additionally, you can set the gap between grid items with the gap-N class
- Responsive Grids: Tailwind excels in responsiveness. Utilize responsive classes to adapt the grid layout for different screen sizes. The example below transforms the grid for various breakpoints.
- Customizing Grid Items: Tailwind empowers you to style individual grid items using utility classes. For instance, you can add padding, borders, and text alignment directly within the HTML structure.
<div class="grid grid-cols-3 gap-4">
<div class="p-4 border">1</div>
<div class="p-4 border">2</div>
<div class="p-4 border">3</div>
<div class="p-4 border">4</div>
<div class="p-4 border">5</div>
<div class="p-4 border">6</div>
</div>
<div class="grid grid-cols-1 md:grid-cols-2 lg:grid-cols-3 gap-4">
<!-- ...grid items... -->
</div>
This method gracefully adjusts to display one column on small screens, two columns on medium screens, and three columns on large screens, maintaining the same functionality.
<div class="grid grid-cols-3 gap-4">
<div class="p-4 border text-center">1</div>
<div class="p-4 border text-center">2</div>
<div class="p-4 border text-center">3</div>
<!-- ... -->
</div>
In this example, p-4 adds padding, border applies a border, and text-center aligns the text in the center.
Conclusion
Mastering CSS Grid opens up a world of possibilities for creating powerful, responsive, and visually appealing web layouts. Despite a learning curve, the advantages, including precise layout control, grid responsiveness, and semantically meaningful markup, make it a valuable skill for modern web designers. Integrating CSS Grid with frameworks like Bootstrap and enhancing it with Tailwind CSS further streamlines the design process. While there are challenges such as browser compatibility and potential complexity, the benefits outweigh them. Embracing CSS Grid empowers designers to craft adaptable and sophisticated layouts, contributing to a seamless user experience across diverse devices.
