Next Basics: Building Your First web Page with next js

Written by
Sukhvinder
Front End Developer
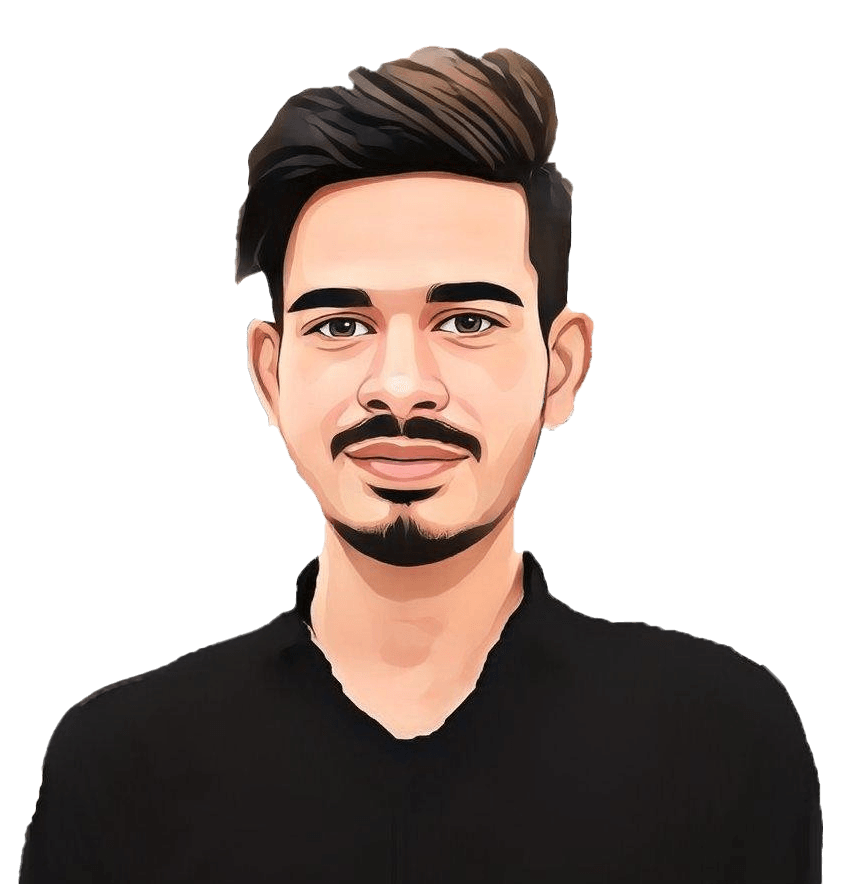
Ravi Kumar
Front End Developer
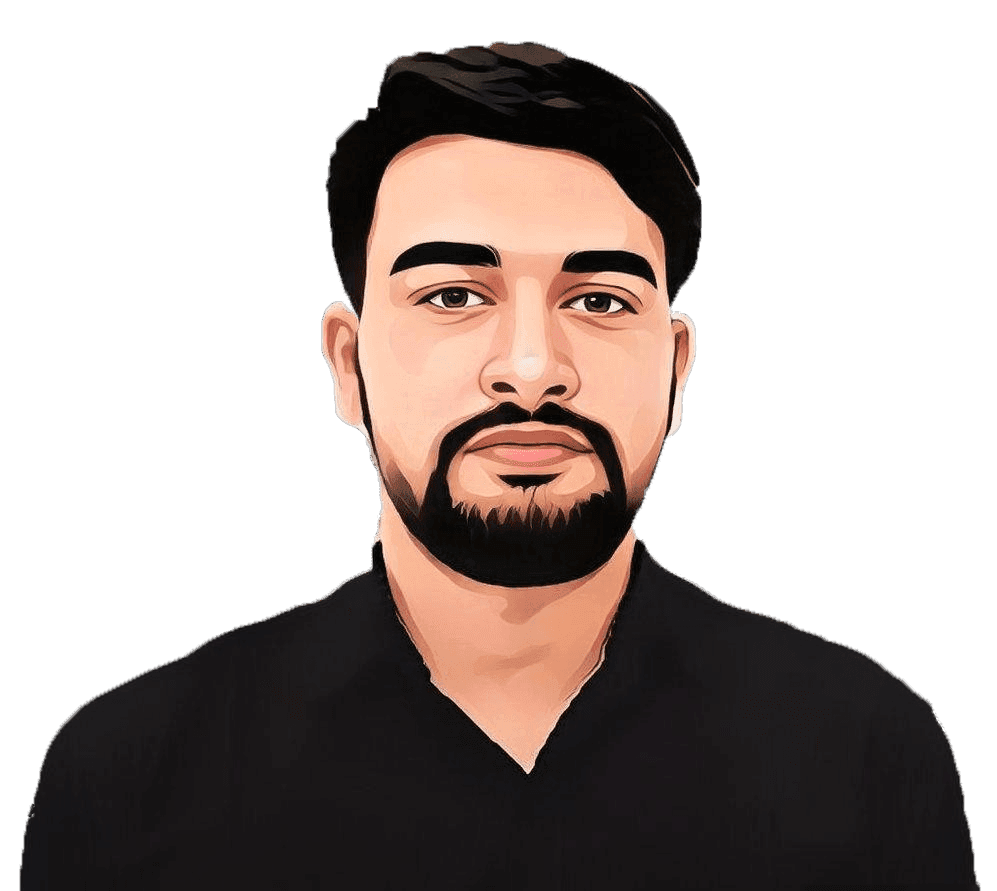
Table of contents
Build with Radial Code
Creating a web page may seem like a daunting task for beginners, but with the right tools and guidance, it becomes an exciting project that anyone can tackle. In this guide, we'll explore building your first web page using Next.js, a powerful framework for building web applications.
What is Next.js?
Next.js is a React framework that facilitates the development of server-side rendering and static web applications. It combines the flexibility of React with the advantages of pre-rendered pages, resulting in fast and user-friendly websites.

Setting Up Your Project
Before diving into the project setup, ensure you have Node.js installed on your computer as it includes npm, the package manager we'll use to install Next.js.
- Installation: To create a new Next.js project, you can use either npm or yarn. Here are the commands for both:
- TypeScript: TypeScript is a superset of JavaScript that adds static typing to the language. Choosing Yeshere means you want to use TypeScript for your project, while Nomeans you prefer to stick with regular JavaScript.
- ESLint: ESLint is a popular tool for identifying and reporting on patterns found in JavaScript code. Selecting Yeshere means you want to use ESLint for your project to ensure code quality and consistency.
- Tailwind CSS: Tailwind CSS is a utility-first CSS framework for quickly building custom designs. Choosing Yeshere means you want to use Tailwind CSS for styling your project.
- Src/ Directory: This option is asking whether you want to organize your project's source code within a src/ directory. It's a common convention to have a dedicated directory for source files. Choosing Yeshere means your source code will be placed inside a src/ directory.
- App Router: This option is asking whether you want to use an App Router. App routers are used for managing different routes within a web application. Choosing "Yes" here means you want to include a router in your project, which is often recommended for single-page applications.
- Customize Default Import Alias (@/ ): This option is asking whether you want to customize the default import alias. Import aliases provide a shorthand way of referencing files or directories in your project. Choosing Yeshere means you want to customize the default alias.
- Import Alias: If you chose to customize the default import alias, you're prompted to specify the alias you want to use. The default alias is commonly set to @/, but you can specify any alias you prefer.
- Development Server: Show how to start the development server using npm run dev or yarn dev. Mention the default port (usually 3000) and how to access the development environment in the browser.
- Deployment: Guide users on how to deploy a Next.js project to platforms like Vercel,, Netlify, Heroku.
npx create-next-app my-next-app
yarn create next-app my-next-app
Replace my-next-appwith the name of your project.
Would you like to use TypeScript? No / Yes
Would you like to use ESLint? No / Yes
Would you like to use Tailwind CSS? No / Yes
Would you like to use `src/` directory? No / Yes
Would you like to use App Router? (recommended) No / Yes
Would you like to customize the default import alias (@/*)? No / Yes
What import alias would you like configured? @/*
npm run dev or yarn dev
Understanding the File Structure

When you start a new Next.js project, you'll find a specific file structure that organizes your project's code and assets. Here's an explanation of the main directories and files:
- pages/: This directory holds your application's pages. Each JavaScript file under this directory represents a route in your application. For example:
- public/: The public directory is where you store static assets such as images, fontsother files. These assets can be referenced in your components using relative paths.
- styles/: This directory is used for organizing your CSS files. Next.js supports CSS modules out of the box, so you can create modular CSS files for each component. For instance:
- components/: While not part of the default file structure, you can create a directory named components to store reusable React components. For example:
- package.json/: This file contains metadata about your project and lists its dependencies. It's where you specify scripts for tasks such as starting the development server and building the project.
- next.config.js: This file allows you to customize Next.js configurations. You can use it to modify webpack configurations, define environment variables, and more.
- .gitignore: This file specifies which files and directories should be ignored by Git. It's typically used to exclude build artifacts, dependencies, and other files that don't need to be versioned.
- README.md: This file contains information about your project, such as installation instructions, usage examples, and any other relevant documentation.
// pages/index.js
function HomePage() {
return <div>Welcome to the home page!</div>;
}
export default HomePage;
This code defines the home page of your application.
/* styles/Header.module.css */
.header {
background-color: #333;
color: #fff;
padding: 1rem;
}
// components/Header.js
import styles from '../styles/Header.module.css';
function Header() {
return This is the header ;
}
export default Header;
Here, we've created a Header component and applied styles from a CSS module file.
This structured organization helps keep your codebase clean and maintainable, making it easier to navigate and collaborate with others.
This file structure is flexible, and you can customize it based on your project's requirements. As your project grows, you may create additional directories and files to better organize your code and assets.
Interested in development services for your project? Visit here.
Creating a New Page
Adding new pages to your Next.js application is straightforward. Each JavaScript file under the pages/ directory represents a route in your application. Follow these steps to create a new page:
- Create a New File: Inside the pages/ directory, create a new JavaScript file. The filename will determine the route of the page. For example, to create an "About" page, you would create a file named about.js.
- Define Page Content: In the newly created file, define the content of your page using React components. Here's an example of how you might create an Aboutpage:
- Save and View: Save the file, and your new page is now accessible at the route corresponding to the filename. For instance, if you created about.js, you can view your About page at http://localhost:3000/about.
// pages/about.js
function AboutPage() {
return (
<div>
<h1>About Us</h1>
<p>Welcome to our website! We are passionate about...</p>
</div>
);
}
export default AboutPage;
By following these steps, you can easily create new pages and add content to your Next.js application. Each page can be as simple or complex as needed, allowing you to build a fully functional web application with ease.
Adding Components
Components allow you to break down your UI into independent and reusable pieces. Let's create a header component:
- Create a Component File: Make a new directory called components/ in your project folder and create a file named Header.jsinside it.
- Define the Component: In Header.js, define the header component by creating a functional component named Header. Customize the content inside the header tag according to your requirements.
- Import and Use: Import the Header component into any page where you want to use it, and include <Header/>in your component's return function.
// Header.js
import React from 'react';
const Header = () => {
return (
<header>
<h1>This is the Header Component</h1>
{/* Add any other header content here */}
</header>
);
};
export default Header;
For example, if you want to use the Header component in a page called HomePage.js:
// HomePage.js
import React from 'react';
import Header from './components/Header'; // Import the Header component
const HomePage = () => {
return (
<div>
<Header /> {/* Include the Header component */}
<h2>Welcome to the Home Page</h2>
{/* Add other content of the home page */}
</div>
);
};
export default HomePage;
Make sure the paths in the import statements are correct according to your project structure. Now, whenever you render HomePagecomponent, the Headercomponent will also be rendered at the top of the page. You can reuse the Headercomponent in any other page where you want to include a header.
Styling Your Pages

Next.js supports CSS modules out of the box, enabling you to write CSS specific to each component without worrying about class name conflicts. Follow these steps to style your pages:
- Create a CSS File: Create a CSS file with the same name as your component, suffixed with .module.css. For example, Header.module.css.
- Write Styles: Write your CSS styles in this file.
- Import and Apply: Import the styles into your component file and use the imported styles object to apply classes to elements in your component.
/* Header.module.css */
.header {
background-color: #333;
color: white;
padding: 10px;
}
.logo {
font-size: 24px;
font-weight: bold;
}
.nav {
list-style: none;
padding: 0;
}
.nav li {
display: inline-block;
margin-right: 10px;
}
.nav li a {
color: white;
text-decoration: none;
}
// Header.js
import styles from './Header.module.css';
const Header = () => {
return (
<header className={styles.header}>
<div className={styles.logo}>My Website</div>
<nav>
<ul className={styles.nav}>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
);
};
export default Header;
Now, you can use the stylesobject imported from the CSS module to apply classes to elements in your component. When the component is rendered, the appropriate CSS classes from the module will be applied to the corresponding elements.
This way, you can keep your CSS scoped to your component and avoid conflicts with other styles in your application.
Deploying Your Web Page

Once you're satisfied with your web page, it's time to share it with the world. Vercel, the creators of Next.js, offer a simple deployment solution:
To deploy a Next.js application using Vercel, follow these steps:
- Push to GitHub: Push your Next.js project code to a GitHub repository.
- Visit Vercel: Visit the Vercel website vercel.com and sign up for an account if you haven't already. Once logged in, import your Next.js project from the GitHub repository.
- Follow Deployment Steps: Vercel will guide you through the deployment process. You'll need to configure settings such as the deployment branch, environment variables, and domain settings.
Here's a basic example of a Next.js project structure and how you can deploy it:
# Create a new Next.js project
npx create-next-app my-next-app
# Navigate into the project directory
cd my-next-app
# Initialize a new Git repository
git init
# Add and commit your code
git add .
git commit -m "Initial commit"
# Create a new GitHub repository
# Push your code to GitHub
git remote add origin <your-github-repo-url>
git push -u origin main
After pushing your code to GitHub, follow these steps:
Visit vercel.com and sign up/login.
- Import your Next.js project from the GitHub repository.
- Follow the deployment steps provided by Vercel, which typically involve selecting the repository, configuring build settings (if necessary), and confirming deployment.
Ensure that your Next.js project is properly configured for deployment. You may need to modify the package.jsonfile to include a buildscript if it's not already present:
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start"
}
This script next buildis crucial for Vercel to build your Next.jsapplication correctly during deployment.
Once deployed, Vercel will provide you with a URL where your Next.js application is hosted. You can then share this URL with others to access your web page.
Congratulations! You've successfully built and deployed your first web page using Next.js. This guide serves as just the beginning of what you can achieve with this powerful framework. Continue learning and experimenting, and you'll be able to create even more amazing web experiences.
By following these steps and understanding the concepts presented, beginners can confidently embark on their journey of web development with Next.js.
Conclusion
Building your first web page with Next.js is both thrilling and empowering, even for beginners. This guide has simplified the process into clear steps, helping you grasp the basics of Next.js. You've gone from project setup to live deployment, learning key skills that form a robust foundation for web development. Next.js merges React's flexibility with server-side rendering and static site generation advantages.
By crafting pages, components, and styling, you've practiced web interface design. Plus, deploying via Vercel means you're ready to share your work globally.
Keep learning and experimenting with Next.js as you progress in web development. With commitment, you'll discover vast possibilities for creating innovative web experiences.
Well done on finishing this guide! With your new knowledge and confidence, you're set to take on more complex projects and deepen your web development expertise. Stay curious and keep improving your abilities to make lasting digital impressions. Happy coding!
