Solving CSS Challenges: Tips and Tricks for Front-End Success
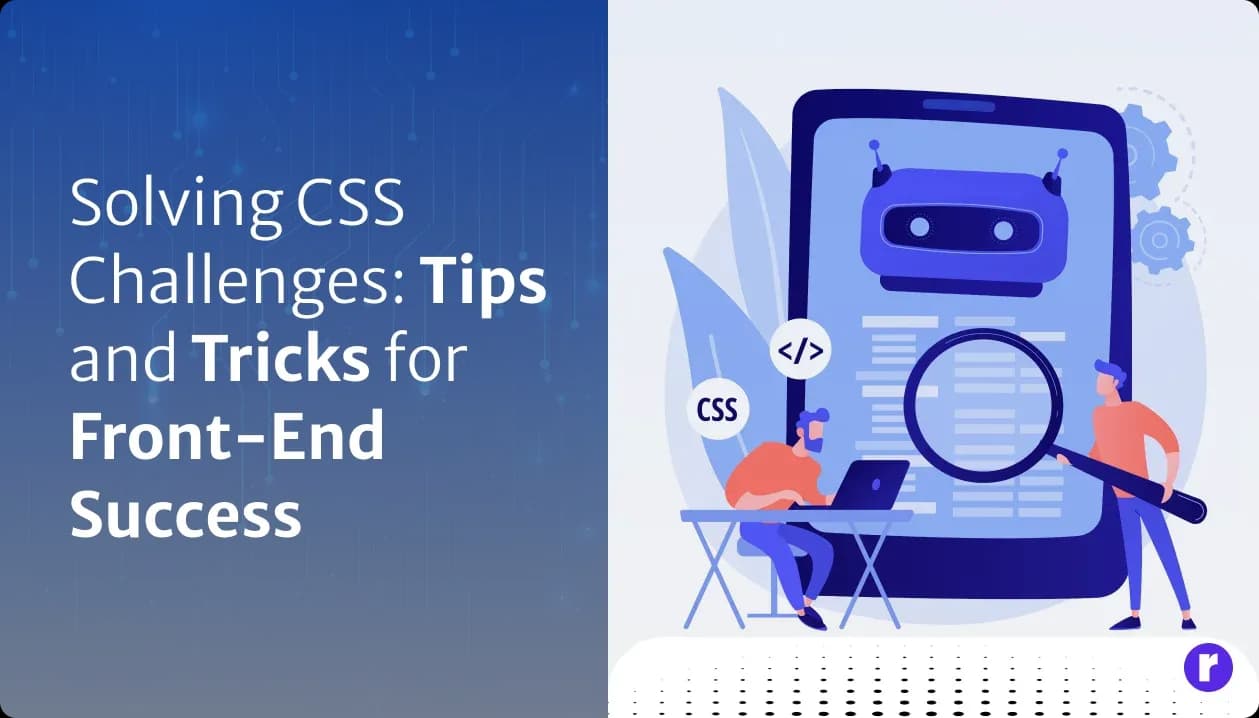
Written by
Pardeep
Front End Developer
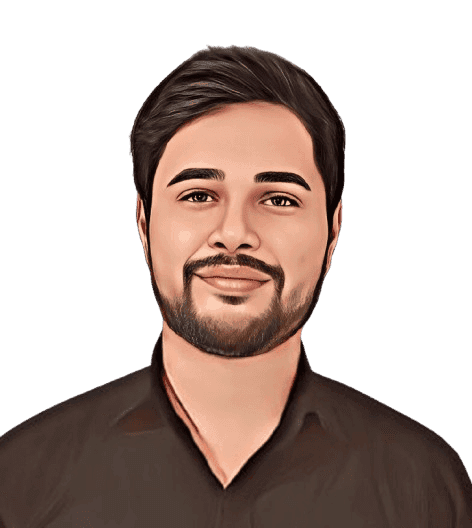
Neha Rai
Front End Developer

Table of contents
Build with Radial Code
Cascading Style Sheets (CSS) form the backbone of web development, offering the means to transform raw HTML content into visually appealing and responsive websites. However, even seasoned developers can find themselves wrestling with CSS challenges. In this guide, we'll explore tips and tricks to help you overcome common front-end hurdles, ensuring a smoother journey toward CSS mastery.
Box Model Mastery
In CSS, there's a fundamental concept known as the box model. It encompasses four essential components: content, padding, border, and margin. These elements work together to shape the layout of an element on a webpage. To control how these elements affect the size of an element, you can use the box-sizing property.
The box-sizing property allows you to define how the browser calculates the total size of an element. There are two main values for this property:
Content-Box (Default)
This is the default value. It includes only the content of the element when calculating its size. Padding, border, and margin are added separately.
Example:
box-sizing: content-box;
Border-Box
This value includes the content, padding, and border when calculating the size. The margin is still added separately.
Example:
box-sizing: border-box;
By adjusting the box-sizing property, you can have more predictable control over the sizing of elements, especially when dealing with padding and borders. This understanding is crucial for creating well-structured and visually appealing layouts in CSS.
Responsive Design
Is crucial in today's world where people use various devices to browse the internet. To make sure your website looks good on all screen sizes, use media queries, flexible grids, and relative units like percentages and em.
Media Queries
These help you apply different styles based on the device's screen size. You can adjust your design for smaller or larger screens.
Example:
/* Responsive styles for smaller screens */
@media screen and (max-width: 600px) {
/* Adjustments for smaller screens */
}
/* Responsive styles for larger screens */
@media screen and (min-width: 1200px) {
/* Enhancements for larger screens */
}
Flexible Grids
Create layouts that adjust to different screen sizes using flexible grids. This means your design can adapt to various devices while keeping a balanced look.
Example:
.container {
width: 100%;
display: grid;
grid-template-columns: repeat(auto-fit,minmax(200px, 1fr));
gap: 20px;
}
Relative Units (Percentages and em)
Instead of fixed measurements, use percentages and em for things like font sizes and container dimensions. This makes your design more adaptable, especially when the user changes their screen size.
Example:
body {
font-size: 16px;
}
.container {
width: 80%;
margin: 0 auto;
}
/* Responsive font size */
h1 {
font-size: 2em;
}
@media screen and (max-width: 768px) {
/* Adjustments for smaller screens */
body {
font-size: 14px;
}
}
Style Queries
A proposed concept in web development could revolutionize CSS by allowing styles to adapt based on individual element characteristics rather than just viewport size. For instance
Example:
/* Hypothetical style query */
.element {
width: 100px;
height: 100px;
}
/* Applying styles based on the width of the element */
@element (min-width: 200px) {
.element {
/* Styles for when the width of the element is at least 200px */
background-color: red;
}
}
This snippet demonstrates how a red background color could be applied to an element when its width exceeds 200 pixels, showcasing the potential of style queries to offer more granular control over CSS styling.
Container Queries
A highly anticipated feature in web development enables CSS rules to dynamically adjust based on the dimensions of containing elements rather than just the viewport size. For instance:
Example:
/* Hypothetical container query */
.container {
max-width: 800px;
}
/* Applying styles based on the container width */
@container (min-width: 600px) {
.element {
/* Media query for container width of at least 600px */
font-size: 18px;
}
}
In this example, the font size of .element dynamically adjusts based on the width of its container, showcasing the potential of container queries to offer more flexible and responsive designs.
Using these techniques ensures that your website looks good and works well on all kinds of devices, providing a better experience for everyone.
Elevate Your Web Design with Our Expert Tips and Tricks of CSS! Learn more.