Supercharge Your Forms with React Hook Form: Effortless Form Management for React Apps
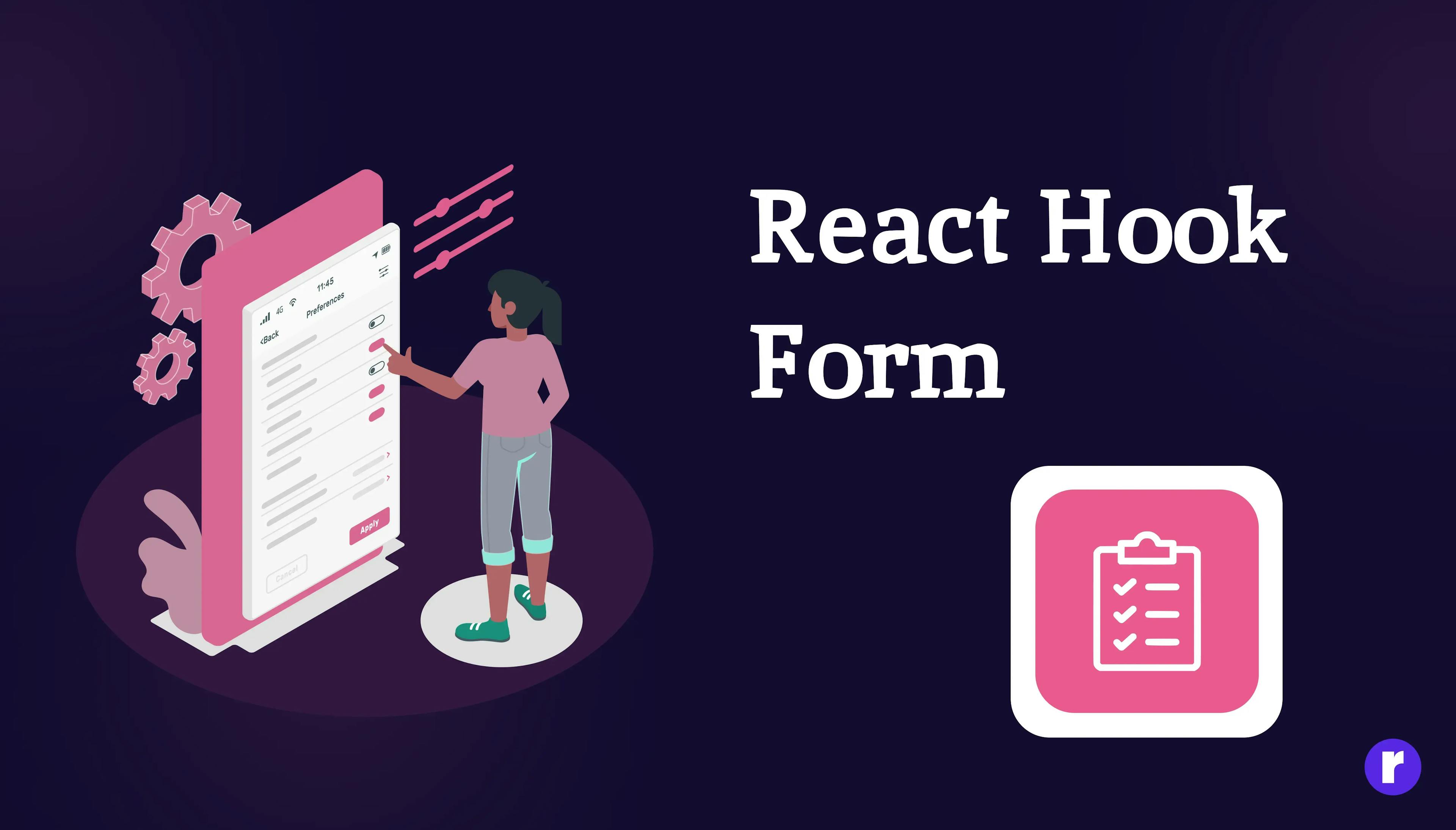
In the realm of web development, forms are the backbone of interactive user experiences. From simple login forms to complex data entry interfaces, forms play a crucial role in collecting user input. However, managing forms in React applications can often be challenging, requiring careful handling of state, validation, and user interactions. This is where React Hook Form comes in – a powerful library that simplifies form management and enhances developer productivity.
Getting Started with React Hook Form
Getting started with React Hook Form is easy. Simply install the library via npm or yarn, and you're ready to go. The library provides a useForm hook, which initializes form state and provides methods for registering form inputs, handling form submissions, and validating input data.
-
Install React Hook Form: Start by installing the library in your project using npm or yarn.
npm install react-hook-form yarn add react-hook-form
-
Import the Hook: Import useForm from the react-hook-form package in your component.
import { useForm } from 'react-hook-form';
-
Initialize Form State: Call the useForm hook in your functional component to initialize the form state and retrieve methods to control the form.
const { register, handleSubmit, errors } = useForm();
-
Attach Input Fields: Add the ref attribute to your form input fields and pass the register function to it. This registers your input fields with React Hook Form.
<input type="text" name="firstName" ref={register} />
-
Handle Form Submission: Define a function to handle form submission. Pass this function to the handleSubmit function returned by useForm.
const onSubmit = (data) => { console.log(data); }; <form onSubmit={handleSubmit(onSubmit)}> {/* Form inputs */} </form>
-
Validate Form Inputs: You can add validation rules using the register function. React Hook Form supports built-in and custom validation rules.
<input type="text" name="email" ref={register({ required: true, pattern: /^\S+@\S+$/i })} /> {errors.email && <span>This field is required.</span>}
Example of React Hook Form Implementation :
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
const {
register,
handleSubmit,
formState: { errors }
} = useForm();
const onSubmit = (data) => {
// Handle form submission
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('firstName')} />
{errors.firstName && <span>{errors.firstName.message}</span>}
<input {...register('lastName')} />
{errors.lastName && <span>{errors.lastName.message}</span>}
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
Effortless Validation with React Hook Form
Validation is a crucial aspect of form management, ensuring that user input meets specific criteria before submission. React Hook Form simplifies validation by allowing developers to define validation rules directly within the registration of form inputs. This approach eliminates the need for complex validation logic and reduces boilerplate code.
<input
{...register('email', {
required: true,
pattern: /^\S+@\S+$/i
})}
/>
{errors.email && <span>Email is required and must be valid</span>}
<input
{...register('password', {
required: true,
minLength: 6
})}
/>
{errors.password && <span>Password is required and must be at least 6 characters long</span>}
Seamless Integration and Extensibility
One of the key strengths of React Hook Form is its seamless integration with existing React components and libraries. Whether you're using functional components, class components, or third-party UI libraries, React Hook Form can be easily integrated into your project without introducing unnecessary complexity. Additionally, the library provides hooks and APIs for handling more advanced form scenarios, such as form arrays, nested fields, and form wizards, making it highly adaptable to a wide range of use cases.

Core Features of React Hook Form
- Effortless Setup: Getting started with React Hook Form is straightforward. By importing the useForm hook, developers can initialize form state and access essential methods for registering form inputs, handling submissions, and managing validation errors.
- Minimalist Syntax: React Hook Form reduces boilerplate code by allowing developers to register form inputs with a simple spread syntax ({...register('fieldName')}). This approach eliminates the need for controlled components and reduces complexity, resulting in cleaner and more maintainable code.
- Built-in Validation: Validating user input is crucial for ensuring data integrity and preventing submission errors. React Hook Form provides built-in support for defining validation rules directly within the registration of form inputs, enabling developers to enforce constraints such as required fields, valid email addresses, and minimum/maximum lengths.
- Performance Optimization: With its focus on performance, React Hook Form minimizes unnecessary re-renders and optimizes form validation processes. By leveraging React's rendering optimizations and memoization techniques, it ensures smooth interactions and efficient resource utilization, even with complex forms and large datasets.
- Seamless Integration: React Hook Form seamlessly integrates with existing React components and libraries, making it compatible with various UI frameworks and development environments. Whether you're using functional components, class components, or third-party UI libraries, React Hook Form can be easily incorporated into your project without disrupting your workflow.
Advanced Functionality and Use Cases

- Custom Validation Rules: In addition to built-in validation rules, React Hook Form allows developers to define custom validation functions to handle complex validation logic and edge cases.
- Dynamic Forms: React Hook Form supports dynamic forms with varying numbers of fields, enabling developers to create dynamic form layouts based on user interactions or data-driven conditions.
- Form Arrays and Nested Fields: For complex data structures, React Hook Form provides support for managing form arrays and nested fields, allowing developers to handle nested data structures with ease.
- Multi-step Forms and Form Wizards: Building multi-step forms or form wizards is simplified with React Hook Form's support for conditional rendering and state management. Developers can implement step-by-step workflows with seamless transitions and persistent form data.
import React, { useState } from 'react';
import { useForm } from 'react-hook-form';
function Step1({ onNext }) {
const { register, handleSubmit } = useForm();
const onSubmit = (data) => {
// Process data if needed
onNext();
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('firstName', { required: true })} />
<button type="submit">Next</button>
</form>
);
}
function Step2({ data, onPrev, onNext }) {
const { register, handleSubmit } = useForm({ defaultValues: data });
const onSubmit = (data) => {
// Process data if needed
onNext();
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('lastName', { required: true })} />
<button type="button" onClick={onPrev}>Previous</button>
<button type="submit">Next</button>
</form>
);
}
function MultiStepForm() {
const [step, setStep] = useState(1);
const [formData, setFormData] = useState({});
const handleNext = () => {
setStep(step + 1);
};
const handlePrev = () => {
setStep(step - 1);
};
const updateFormData = (data) => {
setFormData({ ...formData, ...data });
};
return (
<div>
{step === 1 && (
<Step1 onNext={() => handleNext()} />
)}
{step === 2 && (
<Step2
data={formData}
onPrev={() => handlePrev()}
onNext={() => handleNext()}
/>
)}
{/* Add more steps as needed */}
</div>
);
}
Enhanced Developer Productivity

- Minimal Boilerplate: React Hook Form drastically reduces boilerplate code, allowing developers to focus on building features rather than wrangling form state and validation logic. With its concise syntax and intuitive API, developers can create forms with fewer lines of code and greater clarity.
- Declarative Approach: Leveraging the power of React's declarative paradigm, React Hook Form enables developers to express form logic in a straightforward and intuitive manner. By declaratively defining form inputs and validation rules, developers can create self-descriptive code that is easy to understand and maintain.
- Code Consistency: React Hook Form promotes code consistency by encouraging a unified approach to form management across projects. With its standardized API and best practices, developers can adopt a consistent coding style that improves collaboration and code readability.
Disadvantage of React-form-hook

- Initial Learning Curve: While React Hook Form strives to simplify form management, developers who are new to React or React hooks may face a learning curve when getting started with the library. Understanding the concepts of hooks, form state management, and validation may require some time and effort.
- Compatibility with Class Components: React Hook Form is primarily designed for use with functional components and may not be as compatible with class components. Developers who rely heavily on class components in their projects may find it challenging to integrate React Hook Form seamlessly.
- Limited Built-in UI Components: Unlike some form libraries that provide a comprehensive set of UI components out of the box, React Hook Form focuses primarily on form management and validation. Developers may need to rely on additional libraries or build custom UI components for specific form elements or layouts.
- Community Support and Resources: While React Hook Form has gained popularity within the React community, it may not have as extensive a support network or as many resources available as more established form libraries. Developers may encounter fewer tutorials, guides, or community contributions compared to larger libraries.
- Dependency Management: As with any third-party library, developers must consider the implications of introducing dependencies into their projects. React Hook Form's compatibility with other libraries and frameworks, as well as its potential impact on application size and performance, should be carefully evaluated.
Conclusion
While React Hook Form offers numerous advantages in terms of simplicity, performance, and flexibility, developers should be aware of potential disadvantages such as the learning curve, limited compatibility with class components, and dependency management considerations. By carefully assessing these factors and considering the specific requirements of their projects, developers can make informed decisions about whether React Hook Form is the right choice for their form management needs.
