Troubleshooting Nuxt.js: A Guide to Overcoming Common Issues

Written by
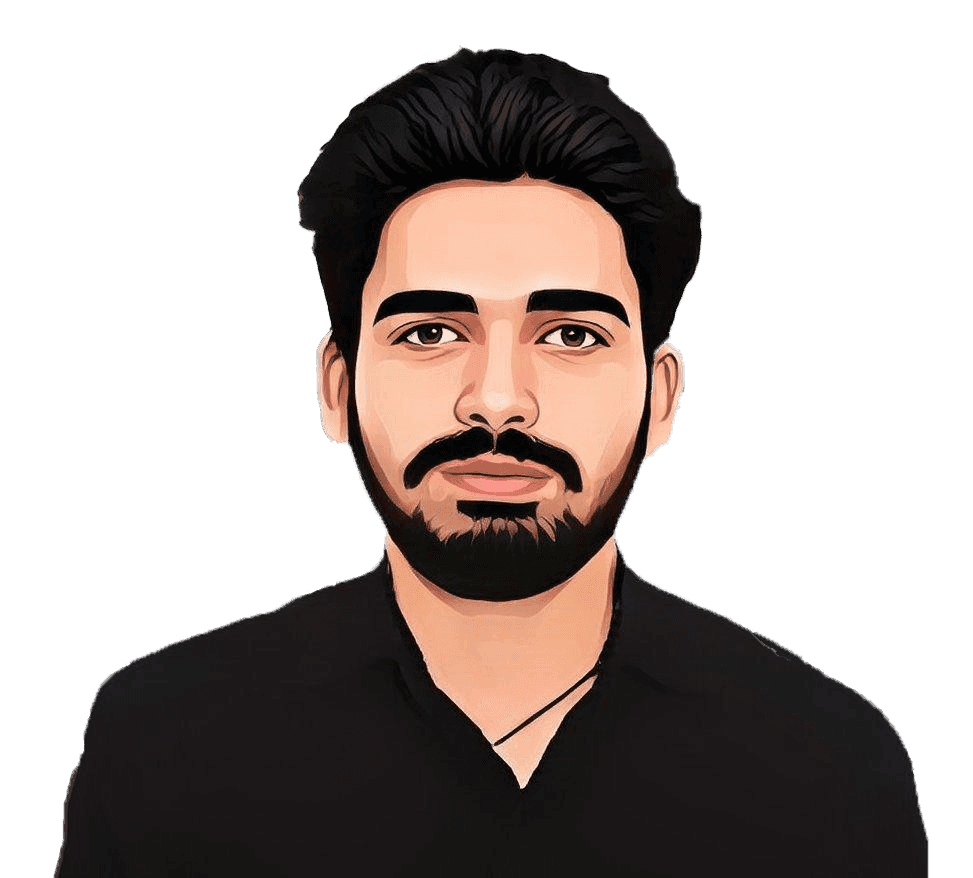
Mohit Bishnoi
Front End Developer
Table of contents
Build with Radial Code
Nuxt.js is a versatile and robust framework for building Vue.js applications, offering features like server-side rendering (SSR), static site generation, and automatic routing. However, as developers dive deeper into the framework, they often encounter various challenges that can slow down the development process. This blog will highlight some common issues developers face when working with Nuxt.js and provide practical solutions with helpful Do’s and Don'ts to streamline your development experience.
Learn how to setup nuxt.js Click here
Page Not Rendering Correctly (SSR Problems)

When using Server-Side Rendering (SSR) in Nuxt.js, certain pages may not render correctly, especially if your application relies on browser-specific objects like window or document. This happens because SSR runs on the server, where these objects are unavailable.
Solution: Always check if the code is running on the client side before accessing browser-specific APIs.
Example:
// Do
if (process.client) {
// This code runs only on the client side
const element = document.querySelector('.some-element');
// Perform DOM operations here
}
// Don't
const element = document.querySelector('.some-element'); // Causes errors on the server side
Do’s:
- Do check process.client before accessing window or document.
Don'ts:
- Don't assume browser APIs are available in a server-side environment.
Route Mismatch Errors

Navigating between routes in Nuxt.js can sometimes trigger errors, especially when the mode is set to history and you refresh the page. These issues typically arise when a route is missing or incorrectly defined.
Solution: Implement error handling for undefined routes and ensure proper route configuration.
Example:
// Do - Use middleware to handle errors
export default function ({ error, route }) {
const validRoutes = ['/home', '/about', '/contact'];
if (!validRoutes.includes(route.path)) {
error({ statusCode: 404, message: 'Page Not Found' });
}
}
Do’s:
- Do set up error handling to manage undefined routes gracefully.
Don'ts:
- Don't leave routes unhandled or assume they will work by default.
Slow Build Times

As your Nuxt.js project grows, you might notice slow build times, especially with large bundles. This is a common issue that can hinder productivity.
Solution: Optimize your build by lazy-loading components and analyzing the bundle size.
Example:
// Do - Lazy load components
export default {
components: {
MyComponent: () => import('@/components/MyComponent.vue')
}
}
// Don't// Eagerly load all components, which leads to a larger bundle size and slower builds.
import MyComponent from '@/components/MyComponent.vue';
Do’s:
- Do use lazy loading to optimize bundle size and reduce build times
Don'ts:
- Don’t import unnecessary components globally.
Errors with Dynamic Routes
Dynamic routes are useful, but if not configured properly, they can lead to "Page Not Found" errors or inconsistent behavior when navigating between pages.
Solution: Use Nuxt.js asyncData or fetchmethods to pre-fetch data for dynamic routes and ensure the routes are defined correctly.
Example:
// Do - Properly define dynamic routes// pages/users/_id.vue
export default {
async asyncData({ params }) {
const user = await fetchUserById(params.id);
return { user };
}
}
// Don't// Forget to handle the fetching of data for dynamic routes.
Do’s:
- Do use asyncData or fetch to load data for dynamic routes ahead of time.
Don'ts:
- Don’t assume Nuxt.js will fetch the data for you automatically.
CSS and Styling Issues

Sometimes, developers encounter issues with CSS not applying correctly, particularly when using scoped styles or global styles in their Nuxt.js applications.
Solution: Make sure global styles are defined in nuxt.config.js and that scoped styles are used appropriately.
Example:
// Do - Import global styles via nuxt.config.js
export default {
css: [
'~/assets/styles/main.css'
]
}
// Don't// Expect scoped styles to handle global styling needs.
Do’s:
- Do define global styles in the nuxt.config.js file for consistent application-wide styling.
Don'ts:
- Don’t overload components with global styles or expect scoped styles to act globally.
Plugins Not Working on the Server-Side

Plugins that depend on browser-specific APIs (like localStorage or cookies) often fail when running on the server during SSR, causing unexpected crashes.
Solution: Use process.client to conditionally register and run plugins on the client side.
Example:
// Do - Conditionally register plugins for client-side use
export default ({ app }, inject) => {
if (process.client) {
const localStoragePlugin = {
getItem: (key) => localStorage.getItem(key)
};
inject('localStorage', localStoragePlugin);
}
};
// Don't// Use `localStorage` or cookies directly without checking if the code is running on the client side.localStorage.getItem('key'); // This will fail during SSR.
Do’s:
- Do register client-side plugins conditionally using process.client.
Don'ts:
- Don’t use browser-specific features without checking the environment.
Conclusion
Nuxt.js provides an excellent framework for building modern Vue.js applications, but it comes with some common pitfalls. By following the best practices discussed in this guide—such as handling SSR issues, optimizing routes, and managing plugins properly—you can avoid many headaches and ensure your development process runs smoothly. Keep these Do’s and Don'ts in mind to troubleshoot effectively and improve your Nuxt.js workflow.
