Understanding the React Router Concept: A Guide for Beginners

Written by
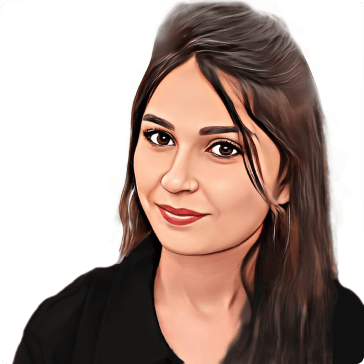
Palvi Tiwari
Front End Developer
Table of contents
Build with Radial Code
React Router is a crucial tool for building Single Page Applications (SPAs) with React. It facilitates smooth navigation by eliminating the need for full page reloads when users interact with links. This guide will explore the fundamental concepts of React Router, helping you create dynamic and intuitive web applications.
React.js Empowers Developers!
React.js simplifies the development of dynamic user interfaces, making it easier to build interactive and responsive web applications.. Leveraging its component-based architecture simplifies UI development, allowing the construction of highly interactive and reusable elements. Its virtual DOM efficiently manages updates, optimizing performance for engaging, responsive web apps. With React, crafting sophisticated user interfaces becomes an intuitive and enjoyable process!
Introduction to React Router

React Router is a powerful library for React that provides routing capabilities for web applications. In an SPA, all of the content loads on a single HTML page, and the view changes based on user interaction with different components. React Router allows you to set up routes within your application, enabling the dynamic loading of components or views based on the specified routes.
By using React Router, developers can create paths within the application, handle complex navigation structures, and manage URL changes seamlessly without refreshing the page.
Why Use React Router?
React Router enhances user experience by making navigation fluid and instantaneous. Here are a few benefits:
- Fast Navigation: The React Router ensures fast navigation by preventing full page reloads, making the application feel more responsive.
- Better User Experience: By providing a seamless experience, users stay engaged as they interact with various parts of the app.
- SEO-Friendly: React Router supports SSR (Server-Side Rendering) through frameworks like Next.js, which helps with SEO.
- Component-Based Routing: It integrates well with React’s component-based architecture, making it easy to maintain and update routes.
Installation of React router
The installation process for React Router involves using package managers like npm or yarn to install the necessary dependencies. React Router primarily includes packages like react-router-dom, react-router, or react-router-native, depending on your specific application requirements.

Installation steps of React router
React Router Dom
React Router Dom is a package used for web applications in React, facilitating navigation and routing functionalities.
Installation using npm
npm install react-router-dom
Installation using yarn
yarn add react-router-dom
Example:
import { BrowserRouter as Router, Route, Link, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<h2>React Router Step By Step Tutorial </h2>
<nav>
<ul>
<li> <Link to='/'>Home </Link> </li>
<li> <Link to='/contact'>Contact </Link> </li>
<li> <Link to='/about'>About </Link> </li>
<li> <Link to='/services'>Services </Link> </li>
</ul>
</nav>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/contact" component={Contact} />
<Route path="/about" component={About} />
<Route path="/services" component={Services} />
</Switch>
</div>
</Router>
);
}
export default App; </code> </pre> </div>
React Router Native
React Router Native is intended for React Native applications, offering navigation and routing capabilities specific to mobile development.
Installation using npm
npm install react-router-native
Installation using yarn
yarn add react-router-native
Example:
import { NativeRouter as Router, Route, Switch } from 'react-router-native';
Core Components of React Router
Let's dive into some essential components of React Router and how each one contributes to setting up navigation:
- BrowserRouter: The BrowserRouter component is the foundation of React Router. It uses the HTML5 history API to keep track of the navigation stack, and it should wrap the entire application. Example:
- Routes and Route: The Routes component is a container for all the defined routes, and Route defines each path and the component to render. Example:
- Link and NavLink: Link are used to navigate between routes without reloading the page. NavLink is similar but adds styling to show the active route. Example:
- useNavigate: useNavigate is a hook that allows programmatically navigating to another route. Example:
- Outlet: Outlet is used for nested routing. It acts as a placeholder where child routes will be rendered within the parent route. Example:
import { BrowserRouter } from 'react-router-dom';
function App() {
return (
<BrowserRouter><Routes />
);
}
import { Routes, Route } from 'react-router-dom';
import Home from './Home';
import About from './About';
function App() {
return (
<BrowserRouter><Routes><Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes></BrowserRouter>
);
}
import { Link, NavLink } from 'react-router-dom';
function Navbar() {
return (
<nav><Link to="/">Home</Link><NavLink to="/about" activeClassName="active">
About
</NavLink></nav>
);
}
import { useNavigate } from 'react-router-dom';
function HomePage() {
const navigate = useNavigate();
function goToAbout() {
navigate('/about');
}
return <button onClick={goToAbout}>Go to About</button>;
}
<Route path="dashboard" element={<Dashboard />}>
<Route path="settings" element={<Settings />} /><Route path="profile" element={<Profile />} />
</Route>
Routing Types
- Static Routing: Defined at build time and does not change. Traditional websites commonly use this.
- Dynamic Routing: Routing structure that adapts as users interact with the app. It is usually fetched and loaded at runtime, making it very useful in SPAs.
React Router Installation Simplified

Basic Setup
Import Router Components
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
Router Component Usage
<Router>
{/* Routes will be defined here */}
</Router>
Defining Routes
Route Component Usage
<Route exact path="/" component={Home} />
<Route path="/about" component={About} /><Route path="/contact" component={Contact} />
Example Components
const Home = () => <h1>Welcome to the Home Page!</h1>;
const About = () => <h1>Learn more About Us here.</h1>;
const Contact = () => <h1>Contact Us</h1>;

Switch Component
import NotFound from './components/NotFound';
import './App.css';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<Nav />
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/user" component={User} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</div>
</Router>
);
}
export default App;
Usage for Matching Routes: Ensures rendering of only the first matched route, optimizing route resolution.
<Switch>
<Route exact path="/" component={Home} /><Route path="/about" component={About} />
<Route path="/contact" component={Contact} /><Route component={NotFound} />
</Switch>
Error Handling (404):
const NotFound = () => <h1>404 - Not Found</h1>;
<Route component={NotFound} />
Nested Routing Structure:
<Route path="/products" component={Products}>
<Route path="/products/:id" component={ProductDetails} />
</Route>
Redirecting to a Route:
import { Redirect } from 'react-router-dom';
<Route exact path="/"><Redirect to="/home" /></Route>
Accessing Parameters in Routes:
<Route path="/user/:username" component={UserProfile} />
Accessing Query Parameters:
// Assuming URL: /search?keyword=react&page=1const searchParams = new
URLSearchParams(location.search);
const keyword = searchParams.get('keyword'); // 'react'const page =
searchParams.get('page'); // '1'
React Router simplifies routing in React applications by enabling seamless navigation between components based on predefined routes. The examples demonstrate how to set up routes, manage errors, create nested routes, perform redirects, and access parameters or query parameters in the URL.
"To learn more about React Router Visit Radial code
Basic Usage of React Router

The basic usage of React Router involves setting up routing within a React application to navigate between different components based on defined routes. Here's a concise outline:
Setting Up Routes
Import necessary components from react-router-dom.
Use the <BrowserRouter> component to wrap your application and define routes using the <Route> component.
Example:
import { BrowserRouter as Router, Route } from 'react-router-dom';
function App() {
return (
<Router><div><Route exact path="/" component={Home} /><Route path="/about" component={About} />
{/* ...other routes */}
</div></Router>
);
}
Navigating Between Routes
Use the <Link> or <NavLink> components to create navigation links within your application.
Example:
import { Link } from 'react-router-dom';
function Navbar() {
return (
<nav><ul><li><Link to="/">Home</Link></li><li><Link to="/about">About</Link></li>
{/* ...other navigation links */}
</ul></nav>
);
}
}
Rendering Components
Each <Route> component renders a specific component when the defined path matches the current URL.
Example:
import { Route } from 'react-router-dom';
function App() {
return (
<div> <Route path="/about" component={About} />
{/* ...other routes */}
</div>
);
}
}
Rendering Components
Each <Route> component renders a specific component when the defined path matches the current URL.
Example:
import { Route } from 'react-router-dom';
function App() {
return (
<div> <Route path="/about" component={About} />
{/* ...other routes */}
</div>
);
}
}
Nested Routes, Redirects, and Switches
Explore advanced features like nested routes, redirects, and using the <Switch> component to render only the first matched route.
React Router enables easy navigation and rendering of different components based on URLs, enhancing the user experience in React applications. This basic setup allows for the creation of a multi-page application within a single-page React app.
Key Components of React Router

BrowserRouter: Provides the capability to synchronize the UI with the current URL in the browser. It uses HTML5 history API to maintain clean URLs.
Example:
import { BrowserRouter as Router } from 'react-router-dom';
<Router>
{/* Your application components and routes */}
</Router><code class="language-html">
Route: Defines a correlation between a URL path and a React component, triggering rendering when the path aligns with the current URL.
Example:
import { Route } from 'react-router-dom';
<Route exact path="/" component={Home} /><Route path="/about" component={About} />
Switch: Renders only the first matched <Route> component. It helps in preventing multiple components from rendering simultaneously for the same URL.
Example:
import { Switch, Route } from 'react-router-dom';
<Switch> <Route path="/about" component={About} />
<Route component={NotFound} /></Switch>
Link/NavLink: Provides navigation functionality by rendering an anchor tag with the benefit of preventing a full page refresh when clicked.
Example:
import { Link, NavLink } from 'react-router-dom';
<Link to="/about">About</Link><NavLink to="/products"
activeClassName="active">Products</NavLink>
Redirect: Redirects the user to another route when a specific condition is met or when triggered programmatically.
Example:
import { Redirect } from 'react-router-dom';
<Route exact path="/"><Redirect to="/home" /></Route>
These components collectively form the core of React Router, allowing developers to build dynamic and navigable web applications in React, handling routing, navigation, and rendering different components based on URLs efficiently.
Common Use Cases
- Creating a Multi-Page Form: Each page of the form can be a separate route, making it easy to navigate between them.
- Dynamic URL Segments: React Router allows adding dynamic segments to the URL, which is helpful for rendering pages like user profiles or blog posts based on unique IDs.
- Breadcrumb Navigation: By nesting routes, you can show users their current location within the site structure with breadcrumbs.
Conclusion
React.js simplifies UI development through its component-based architecture and virtual DOM, optimizing performance for engaging web apps. React Router empowers seamless single-page experiences, enabling dynamic user journeys with BrowserRouter, Route, Link, Switch, and Redirect components. These form the core of React Router, facilitating efficient navigation and rendering based on URLs.
Installation of React Router via npm or yarn allows effortless setup of routes for dynamic navigation and URL handling in React applications. The basic usage of React Router facilitates easy navigation between components, route setup, redirects, and URL parameter access, ensuring a smooth user experience.
