Routing in React, Next, and html with Vite js

Written by
Anjali Chanda
Front End Developer

Neha Rai
Front End Developer

Table of contents
Build with Radial Code
Routing is a crucial aspect of web development, allowing users to navigate through different pages and content seamlessly. In this blog, we will explore routing in React, Next.js, and HTML with Vite.js. Each of these technologies offers unique features and benefits for handling routing in web applications. Let's dive into how routing works in these frameworks and libraries to create efficient and user-friendly web experiences.
Routing in React
Routing is crucial to building single-page applications (SPAs) in React. It enables navigation between different components or views within the application without reloading the entire page. This is where React Router comes in, a powerful library for handling routing in React applications.
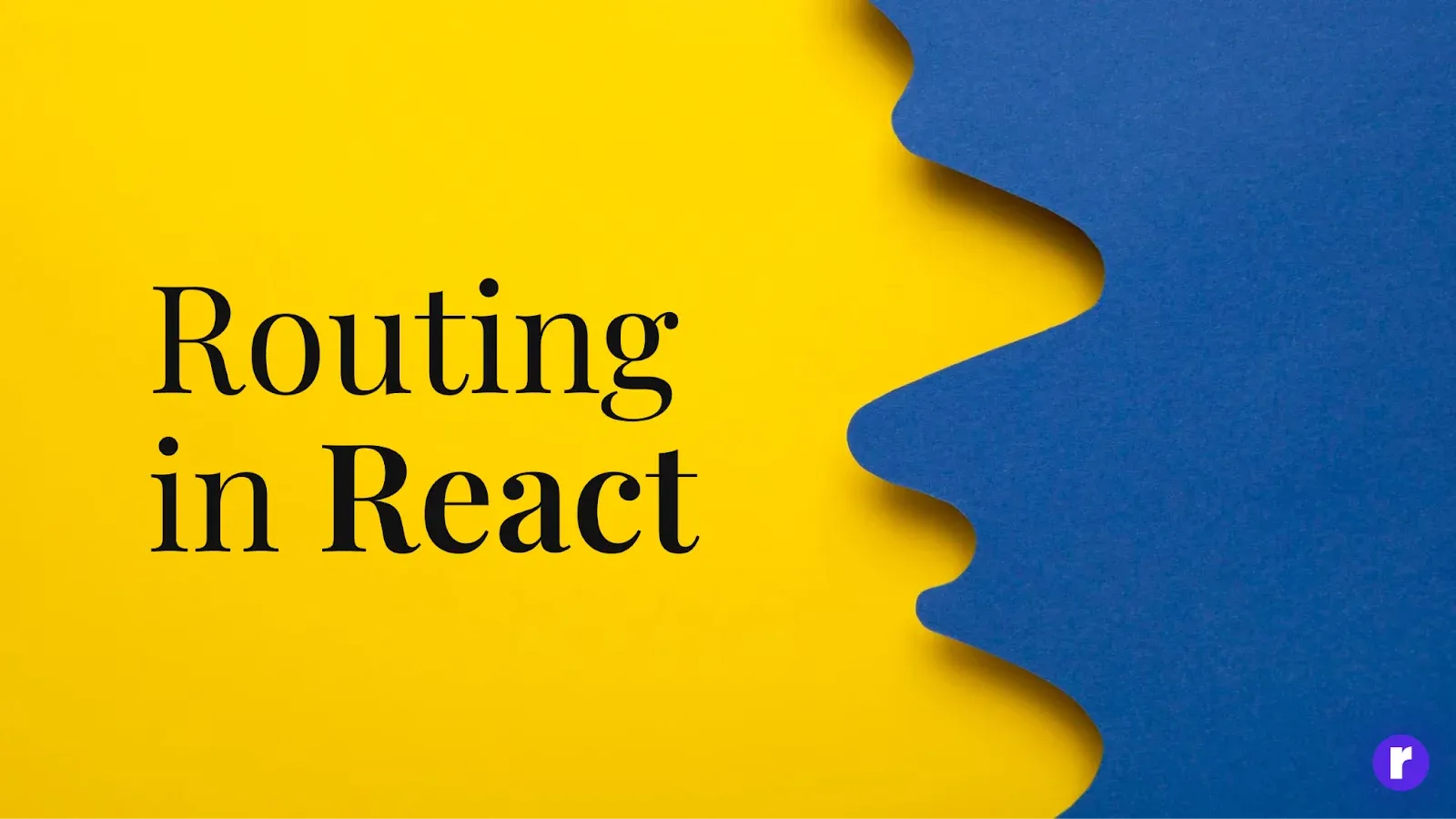
Setting Up React Router
npm install react-router-dom
Import BrowserRouter and Route Components.
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
Define Routes in Your Application
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
);
}
export default App;
In this example, BrowserRouter wraps your application, enabling the routing functionality. The switch ensures that only one route is rendered at a time, and the Route defines the paths and corresponding components.
Navigating Between Routes
import { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
);
}
export default App;
Running the React App
npm start
Routing in Next.js
Next.js is a powerful React framework that simplifies building server-side rendered applications and static websites. One of its standout features is its built-in routing system, which is file-based, making it intuitive and easy to use.
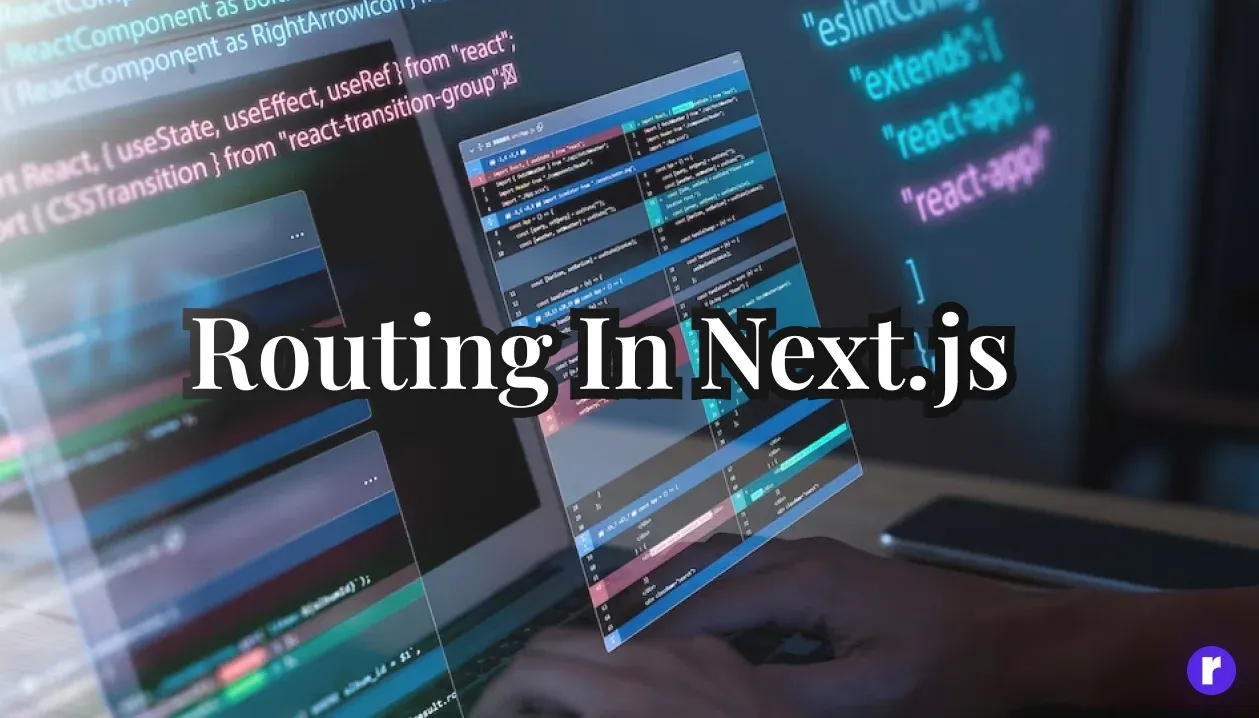
Want to get a website for your business? We're here to help!
File-Based Routing
Next.js is a React framework that provides a powerful, file-based routing mechanism. This system leverages the filesystem structure to generate routes for your application automatically. Here's an in-depth look at how this works.
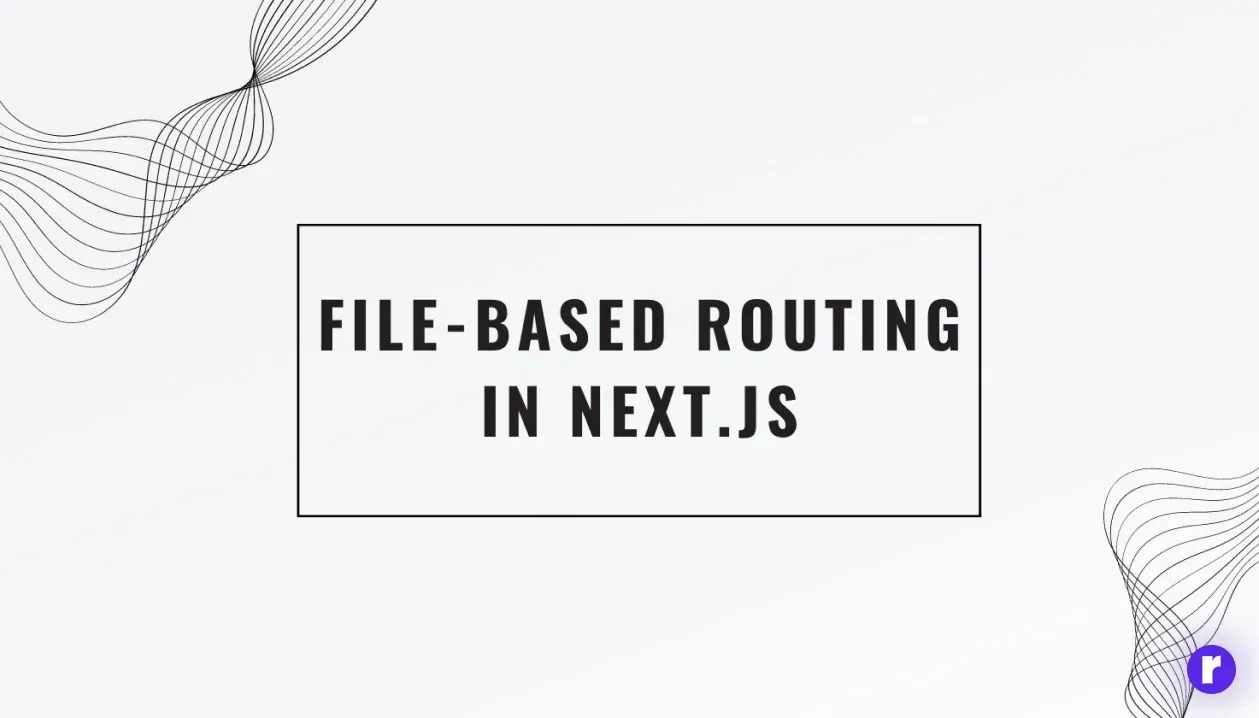
Basic Routes:
Simply create a JavaScript or TypeScript file in the pages directory. For example: pages/index.js becomes the homepage (/).
// pages/index.js
import Link from 'next/link';
const Home = () => (
<div>
<h1>Home Page</h1>
<nav>
<ul>
<li>
<Link href="/about">
<a>About</a>
</Link>
</li>
</li>
<Link href="/contact">
<a>Contact</a>
</Link>
</li>
</ul>
</nav>
</div>
);
export default Home;;
pages/about.js becomes the about page (/about).
//pages/about.js
const About = () => (
<div>
<h1> About Page</h1>
<p> This is the about page.</p>
</div>
);
export default About;
pages/contact.js becomes the contact page (/contact).
//pages/contact.js
const Contact = () => (
<div>
<h1> Contact Page</h1>
<p> This is the Contact page.</p>
</div>
);
export default Contact;
Dynamic Routes:
Dynamic routing in Next.js is just as straightforward. You can create dynamic routes by using square brackets in the file names. For example:
pages/blog/[id].js maps to /blog/:id, where id is a dynamic parameter.
//pages/blog/[id].js
import { useRouter } from 'next/router';
const BlogPost = () => (
const router = useRouter();
const { id } = router.query;
return <div>Blog Post: {id}</div>;
);
export default BlogPost;;
<Link>
In React.js, the <Link> component is typically used from the React Router library to create links within single-page applications (SPAs). It enables navigation between different views or pages without causing a full page reload.
import { Link } from 'react-router-dom';
function MyComponent () {
return (
<div>
<Link to="/about">About</Link>
</div>
);
}
Linking Between Pages
Next.js provides a built-in Link component to navigate between different pages, which optimizes routing by prefetching pages in the background.
import Link from 'next/link';
function Navigation() {
return (
<nav>
<ul>
<li><Link href="/"><a>Home</a></Link></li>
<li><Link href="/about"><a>About</a></Link></li>
<li><Link href="/contact"><a>Contact</a></Link></li>
</ul>
</nav>
);
}
export default Navigation;
Nested Routes
For nested routes, you can create a folder structure within the pages directory:
- pages/blog/index.js for /blog
- pages/blog/[id].js for /blog/:id
Running the Next.js App
npm run dev
Routing for Html with Vite.js
Vite.js is a modern build tool providing a fast and efficient development experience. It is particularly well-suited for modern JavaScript frameworks like Vue and React. However, it can also be configured to handle basic HTML-based projects. Unlike frameworks like Next.js that offer built-in routing, Vite focuses on being an extremely fast and minimalistic build tool. You'll typically rely on client-side routing libraries for routing in a Vite-based HTML project.
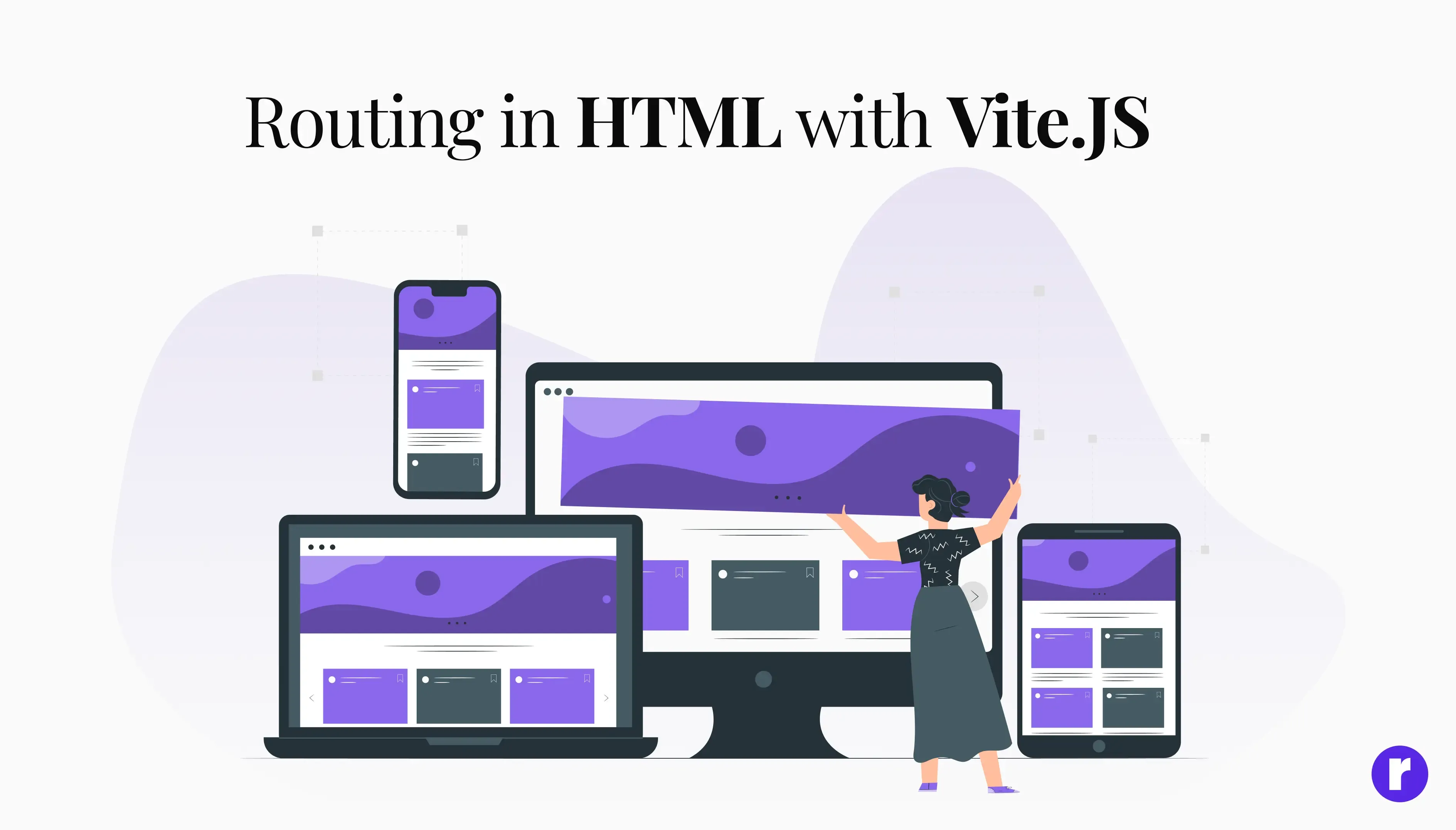
Setting Up Vite.js
To get started with Vite, ensure you have Node.js installed on your machine. Create a new Vite project with the following commands:
npm create vite@latest my-vite-app
cd my-vite-app
npm install
Vite Configuration (vite.config.js)
In your Vite project, the configuration file vite.config.js allows you to customize the build process. Here’s a basic example of a vite.config.js file.
import { resolve } from "path";
import { defineConfig } from "vite";
export default defineConfig({
build: {
rollupOptions: {
input: {
main: resolve("./", "index.html"),
About: resolve("./", "about.html"),
Service: resolve("./", "service.html"),
},
},
},
});
Start the Vite development server using
npm run dev
Conclusion
Routing is a crucial part of any web application, providing a way for users to navigate through different sections.
React: Uses React Router for declarative, component-based routing, making it easy to manage dynamic navigation. Know more about React.js Visit here
Next.js: Simplifies routing with its file-based system, automatically mapping files in the pages directory to routes, supporting dynamic routes, and integrating backend API routes seamlessly. Know more about Next.js Visit here
Vite.js: With HTML leverages lightweight libraries like Navigo or custom hash-based routing, offering a fast development setup with minimal configuration.
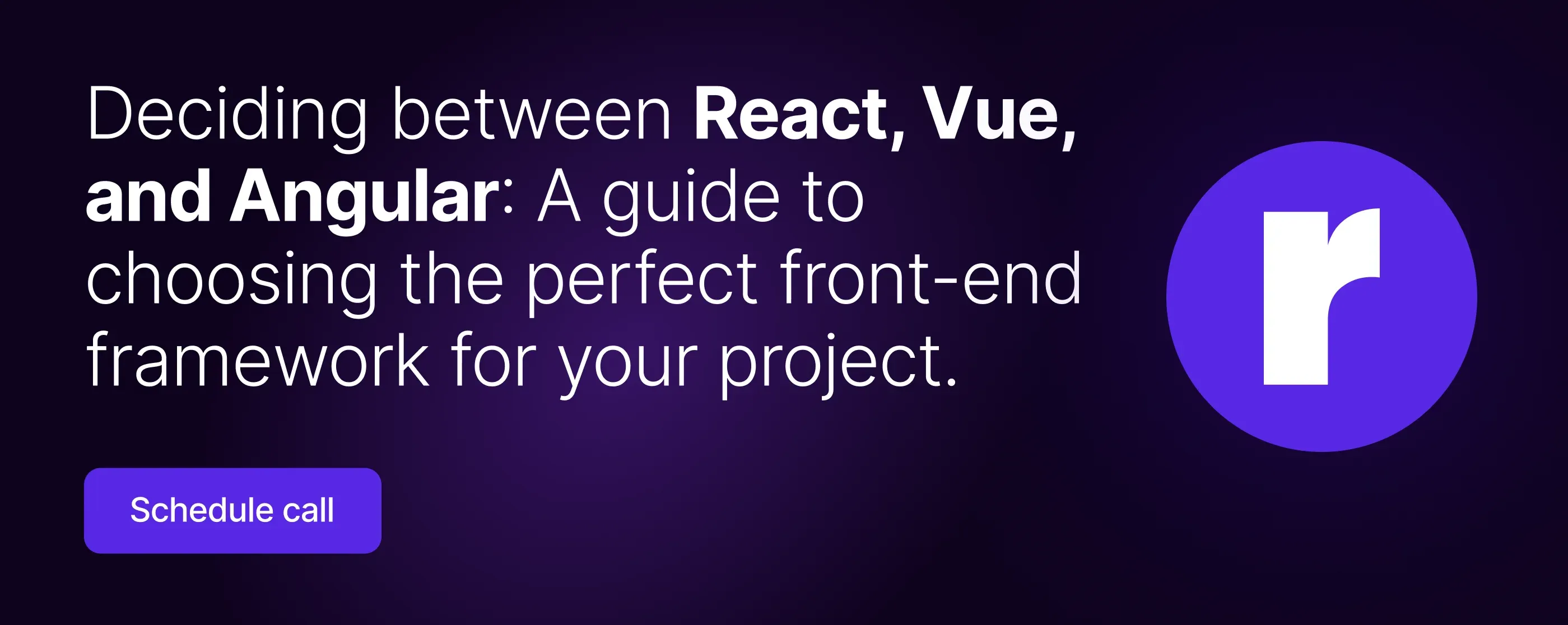